Suggested Videos
Part 29 - Using Bootstrap with Angular 2 | Text | Slides
Part 30 - Angular 2 routing tutorial | Text | Slides
Part 31 - Angular 2 route parameters | Text | Slides
In this video we will discuss
1. What is Dependency Injection
2. How dependency injection works in angular
Let us understand Dependency Injection in Angular with an example. Consider this piece of code in EmployeeListComponent.
What is Dependency Injection
It's a coding pattern in which a class receives its dependencies from an external source rather than creating them itself.
So if we relate this definition to our example, EmployeeListComponent has a dependency on EmployeeService. The EmployeeListComponent receives the dependency instance (i.e EmployeeService instance) from the the external source (i.e the angular injector) rather than creating the instance itself.
Part 29 - Using Bootstrap with Angular 2 | Text | Slides
Part 30 - Angular 2 routing tutorial | Text | Slides
Part 31 - Angular 2 route parameters | Text | Slides
In this video we will discuss
1. What is Dependency Injection
2. How dependency injection works in angular
Let us understand Dependency Injection in Angular with an example. Consider this piece of code in EmployeeListComponent.
export class EmployeeListComponent
implements OnInit {
private _employeeService: EmployeeService;
constructor(_employeeService: EmployeeService) {
this._employeeService = _employeeService;
}
ngOnInit() {
this._employeeService.getEmployees()
.subscribe(
employeesData => this.employees = employeesData,
error => this.statusMessage = 'Error');
}
// Rest of the
code
}
- In EmployeeListComponent we need an instance of EmployeeService, so we could use that instance to call getEmployees() method of the service which retrieves the list of employees which this component needs.
- But if you look at this code, the EmployeeListComponent is not creating an instance of EmployeeService.
- We declared a private field _employeeService of type EmployeeService. The constructor also has a parameter _employeeService of type EmployeeService. The constructor is then initializing the private class field _employeeService with it's parameter.
- We are then using this private field _employeeService to call the service method getEmployees()
- The obvious question that we get at this point is how are we getting an instance of the EmployeeService class.
- We know for sure the EmployeeListComponent is not creating the instance of the EmployeeService class.
- From looking at the code we can understand that the constructor is provided with an instance of EmployeeService class, and then the constructor is assigning that instance to the private field _employeeService.
- At this point, the next question that comes to our mind is who is creating and providing the instance to the constructor.
- The answer to this question is the Angular Injector. When an instance of EmployeeListComponent is created, the angular injector creates an instance of the EmployeeService class and provides it to the EmployeeListComponent constructor. The constructor then assigns that instance to the private field _employeeService. We then use this private field _employeeService to call the EmployeeService method getEmployees().
- Another question that comes to our mind is - How does the angular injector knows about EmployeeService.
- For the Angular injector to be able to create and provide an instance of EmployeeService, we will have to first register the EmployeeService with the Angular Injector. We register a service with the angular injector by using the providers property of @Component decorator or @NgModule decorator.
- We already know we decorate an angular component with @Component decorator and an angular module with @NgModule decorator. So this means if we are registering a service using the providers property of the @Component decorator then we are registering the service with an angular injector at the component level. The service is then available to that component and all of it's children.
- On the other hand if we register the service using the providers property of the @NgModule decorator then we are registering the service with an angular injector at the module level which is the root injector. The service registered with the root injector is then available to all the component accross the entire application.
- We will discuss these hierarchical injectors in Angular in detail with an example in our upcoming videos.
- We register a service with the angular injector by using the providers property of @Component decorator or @NgModule decorator.
- When a component in Angular needs a service instance, it does not explicitly create it. Instead it just specifies it has a dependency on a service and needs an instance of it by including the service as a constructor parameter.
- When an instance of the component is created, the angular injector creates an instance of the service class and provides it to component constructor.
- So the component which is dependent on a service instance, receives the instance from an external source rather than creating it itself. This is called Dependency Injection.

What is Dependency Injection
It's a coding pattern in which a class receives its dependencies from an external source rather than creating them itself.
So if we relate this definition to our example, EmployeeListComponent has a dependency on EmployeeService. The EmployeeListComponent receives the dependency instance (i.e EmployeeService instance) from the the external source (i.e the angular injector) rather than creating the instance itself.
- Why should we use Dependency Injection?
- What benefits it provide?
- Why can't we explicitly create an instance of the EmployeeService class using the new keyword and use that instance instead in our EmployeeListComponent?
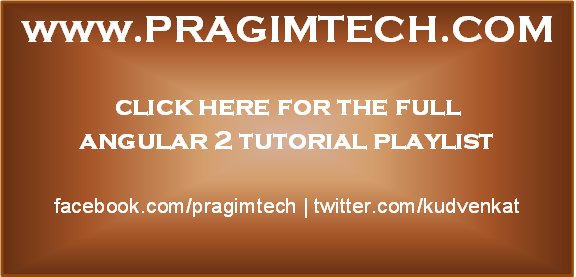
Hi Venkat sir please can u Explain angular2 with CURD operation using WebApi ?
ReplyDelete