Suggested Videos
Part 23 - Interfaces in Angular 2 | Text | Slides
Part 24 - Angular component lifecycle hooks | Text | Slides
Part 25 - Angular services tutorial | Text | Slides
In this video we will discuss creating ASP.NET Web API service that retrieves employees data from a database table. In our next video we will discuss, how to call this ASP.NET Web API service using Angular
Step 1 : Execute the following SQL Script using SQL Server Management studio. This script creates
Step 2 : To keep Angular and Web API projects separate, let's create a new project for our Web API Service. Right click on "Angular2Demo" solution in the Solution Explorer and select Add - New Project.
Step 3 : In the Add New Project window
Step 5 : Add ADO.NET Entity Data Model to retrieve data from the database. Right click on "EmployeeWebAPIService" project and select Add - New Item
Step 7 : On the next screen, click "New Connection" button
Step 8 : On "Connection Properties" window, set
Adding Web API Controller
1. Right click on the Controllers folder in EmployeeWebAPIService project and select Add - Controller
2. Select "Web API 2 Controller - Empty" and click "Add"
3. On the next screen set the Controller Name = EmployeesController and click Add
4. Copy and paste the following code in EmployeesController.cs
At this point when you navigate to /api/employees you will see all the employees as expected. However, when you navigate to /api/employees/emp101, we expect to see employee whose employee code is emp101, but we still see the list of all employees.
This is because the parameter name for the Get() method in EmployeesController is "code"
but in the default Web API route in WebApiConfig.cs file the parameter name is {id}. Change this to "code" as shown below
With this change if we navigate to /api/employees/emp101 we see just that employee whose employee code is "emp101"
In our next video we will discuss, how to call this ASP.NET Web API service using Angular
Part 23 - Interfaces in Angular 2 | Text | Slides
Part 24 - Angular component lifecycle hooks | Text | Slides
Part 25 - Angular services tutorial | Text | Slides
In this video we will discuss creating ASP.NET Web API service that retrieves employees data from a database table. In our next video we will discuss, how to call this ASP.NET Web API service using Angular
Step 1 : Execute the following SQL Script using SQL Server Management studio. This script creates
- EmployeeDB database
- Creates the Employees table and populate it with sample data
Create Database EmployeeDB
Go
Use EmployeeDB
Go
Create table Employees
(
code nvarchar(50) primary key,
name nvarchar(50),
gender nvarchar(50),
annualSalary
decimal(18,3),
dateOfBirth
nvarchar(50)
)
Go
Insert into Employees values ('emp101', 'Tom', 'Male', 5500, '6/25/1988')
Insert into Employees values ('emp102', 'Alex', 'Male', 5700.95, '9/6/1982')
Insert into Employees values ('emp103', 'Mike', 'Male', 5900, '12/8/1979')
Insert into Employees values ('emp104', 'Mary', 'Female', 6500.826, '10/14/1980')
Insert into Employees values ('emp105', 'Nancy', 'Female', 6700.826, '12/15/1982')
Insert into Employees values ('emp106', 'Steve', 'Male', 7700.481, '11/18/1979')
Step 2 : To keep Angular and Web API projects separate, let's create a new project for our Web API Service. Right click on "Angular2Demo" solution in the Solution Explorer and select Add - New Project.
Step 3 : In the Add New Project window
- Select "Visual C#" under "Installed - Templates"
- From the middle pane select, ASP.NET Web Application
- Name the project "EmployeeWebAPIService" and click "OK"
Step 5 : Add ADO.NET Entity Data Model to retrieve data from the database. Right click on "EmployeeWebAPIService" project and select Add - New Item
- In the "Add New Item" window
- Select "Data" from the left pane
- Select ADO.NET Entity Data Model from the middle pane
- In the Name text box, type EmployeeDataModel and click Add
Step 7 : On the next screen, click "New Connection" button
Step 8 : On "Connection Properties" window, set
- Server Name = (local)
- Authentication = Windows Authentication
- Select or enter a database name = EmployeeDB
- Click OK and then click Next
Adding Web API Controller
1. Right click on the Controllers folder in EmployeeWebAPIService project and select Add - Controller
2. Select "Web API 2 Controller - Empty" and click "Add"
3. On the next screen set the Controller Name = EmployeesController and click Add
4. Copy and paste the following code in EmployeesController.cs
using System.Collections.Generic;
using System.Linq;
using System.Web.Http;
namespace EmployeeWebAPIService.Controllers
{
public class EmployeesController : ApiController
{
public IEnumerable<Employee> Get()
{
using(EmployeeDBEntities entities = new EmployeeDBEntities())
{
return entities.Employees.ToList();
}
}
public Employee Get(string code)
{
using (EmployeeDBEntities entities = new EmployeeDBEntities())
{
return entities.Employees.FirstOrDefault(e => e.code == code);
}
}
}
}
At this point when you navigate to /api/employees you will see all the employees as expected. However, when you navigate to /api/employees/emp101, we expect to see employee whose employee code is emp101, but we still see the list of all employees.
This is because the parameter name for the Get() method in EmployeesController is "code"
public Employee Get(string code)
{
using (EmployeeDBEntities entities = new EmployeeDBEntities())
{
return entities.Employees.FirstOrDefault(e
=> e.code == code);
}
}
but in the default Web API route in WebApiConfig.cs file the parameter name is {id}. Change this to "code" as shown below
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{code}",
defaults: new { code = RouteParameter.Optional }
);
With this change if we navigate to /api/employees/emp101 we see just that employee whose employee code is "emp101"
In our next video we will discuss, how to call this ASP.NET Web API service using Angular
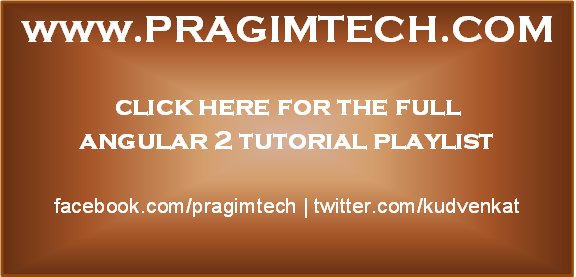
I am getting the following error when viewing the api project:
ReplyDeleteThe resource cannot be found.
Description: HTTP 404. The resource you are looking for (or one of its dependencies) could have been removed, had its name changed, or is temporarily unavailable. Please review the following URL and make sure that it is spelled correctly.
Requested URL: /api.
Does some setting in my project needs to be changed?
I also had this issue. so I installed:
DeleteInstall-Package Microsoft.AspNet.WebApi.WebHost at nuget prompt, and then added the following to the global.aspx
GlobalConfiguration.Configure(WebApiConfig.Register);
And then it worked.
after step 8 Entity Data Model wizard is going to close.....can u tell me solution
ReplyDeleteI am using VS2015 with mysql window 8 64-bit.
can you make the tutorial angular with java. it will really helpful for me.
ReplyDeleteThanks
My Goal to "minimize using server resources with better performance".
ReplyDeleteI understand Angular as Client Side Model (may correct me).
In best practices when using angular to access a database. which is the best approach?
* Using angular with web services only.
OR
* Using angular along with other server-side such as .Net framework.