Suggested Videos
Part 15 - AngularJS ng-init directive
Part 16 - ng-include directive in AngularJS
Part 17 - Consuming ASP.NET Web Service in AngularJS using $http
In this video we will discuss $http service in AngularJS.
In Angular there are several built in services. $http service is one of them. In this video, we will discuss another built in service, $log. It is also possible to create our own custom services in Angular.
At this point several questions come to our mind
So, let's start our discussion with $http service.
$http service in Angular is used to make HTTP requests to remote server
$http service is a function that has a single input parameter i.e a configuration object.
Example : The following example issues a GET request to the specified URL
In the example above we are only using 2 properties of the configuration object. Check the link below for the complete list of properties supported by the configuration object
https://docs.angularjs.org/api/ng/service/$http#usage
Shortcut methods like get, post, put, delete etc are also available to be used with $http service
Example : Using the short cut method get()
$http.get('EmployeeService.asmx/GetAllEmployees')
$http service returns a promise object. This means the functions are executed asynchronously and the data that these functions return may not be available immediately. Because of this reason you cannot use the return value of the $http service as shown below.
$scope.employees = $http.get('EmployeeService.asmx/GetAllEmployees');
Instead you will use the then() method. The successCallback function that is passed as the parameter to the then function is called when the request completes. The successCallback function receives a single object that contains several properties. Use the data property of the object to retrieve the data received from the server.
You can use the $log service to log the response object to the console to inspect all of it's properties
If there is an error processing the request, the errorCallback function is called. The errorCallback function is passed as the second parameter to the then() function. The errorCallback function receives a single object that contains several properties. Use the data or statusText properties of the returned object to find the reasons for the failure.
You can use the $log service to log the response object to the console to inspect all of it's properties
You can also create separate functions and associate them as successCallback and errorCallback functions
Default Transformations provided by Angular's http service
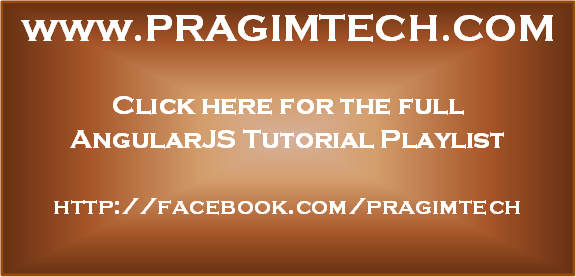
Part 15 - AngularJS ng-init directive
Part 16 - ng-include directive in AngularJS
Part 17 - Consuming ASP.NET Web Service in AngularJS using $http
In this video we will discuss $http service in AngularJS.
In Angular there are several built in services. $http service is one of them. In this video, we will discuss another built in service, $log. It is also possible to create our own custom services in Angular.
At this point several questions come to our mind
- What are services in Angular
- When should we be creating services in Angular
- How to create our own custom Angular services
- Where do they fit, in an angular application architecture
- What are the benefits of using services
So, let's start our discussion with $http service.
$http service in Angular is used to make HTTP requests to remote server
$http service is a function that has a single input parameter i.e a configuration object.
Example : The following example issues a GET request to the specified URL
$http({
method: 'GET',
url: 'EmployeeService.asmx/GetAllEmployees'
});
In the example above we are only using 2 properties of the configuration object. Check the link below for the complete list of properties supported by the configuration object
https://docs.angularjs.org/api/ng/service/$http#usage
Shortcut methods like get, post, put, delete etc are also available to be used with $http service
Example : Using the short cut method get()
$http.get('EmployeeService.asmx/GetAllEmployees')
$http service returns a promise object. This means the functions are executed asynchronously and the data that these functions return may not be available immediately. Because of this reason you cannot use the return value of the $http service as shown below.
Instead you will use the then() method. The successCallback function that is passed as the parameter to the then function is called when the request completes. The successCallback function receives a single object that contains several properties. Use the data property of the object to retrieve the data received from the server.
$scope.employees
= $http.get('EmployeeService.asmx/GetAllEmployees')
.then(function (response) {
$scope.employees =
response.data;
});
You can use the $log service to log the response object to the console to inspect all of it's properties
$scope.employees
= $http.get('EmployeeService.asmx/GetAllEmployees')
.then(function (response) {
$scope.employees =
response.data;
$log.info(response);
});
If there is an error processing the request, the errorCallback function is called. The errorCallback function is passed as the second parameter to the then() function. The errorCallback function receives a single object that contains several properties. Use the data or statusText properties of the returned object to find the reasons for the failure.
$scope.employees
= $http.get('EmployeeService.asmx/GetAllEmployee')
.then(function (response) {
$scope.employees =
response.data;
}, function (reason) {
$scope.error = reason.data;
});
You can use the $log service to log the response object to the console to inspect all of it's properties
$scope.employees
= $http.get('EmployeeService.asmx/GetAllEmployee')
.then(function (response) {
$scope.employees =
response.data;
}, function (reason) {
$scope.error =
reason.data;
$log.info(reason);
});
You can also create separate functions and associate them as successCallback and errorCallback functions
var successCallBack = function (response) {
$scope.employees = response.data;
};
var errorCallBack = function (reason) {
$scope.error = reason.data;
}
$scope.employees
= $http.get('EmployeeService.asmx/GetAllEmployees')
.then(successCallBack,
errorCallBack);
Default Transformations provided by Angular's http service
- If the data property of the request configuration object contains a JavaScript object, it is automatically converted into JSON object
- If JSON response is detected, it is automatically converted into a JavaScript object
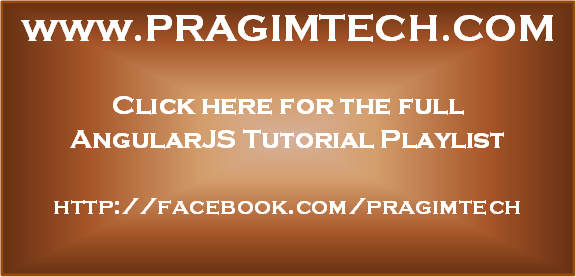
Very nice tutorial. Thank you very much.
ReplyDeleteHi Venkat....please create one tutorial for $http.post service also
ReplyDelete.....which have CORS in it...
Thanks
Manish
Hi Venkat, I have everything setup the same as you do but $log.info(response) is not outputting anything to the console in my Chrome Version 60.0.3112.90 (Official Build) (64-bit). Any ideas to why is that?
ReplyDeleteSolved it: apparently chrome was using cashed version of the page, after using incognito or removing history it works fine now
DeleteFrom next time onward, use Cntrl + Shift + R to reload page and it will not use cached JS file.
Delete