Suggested Videos
Part 14 - ng-hide and ng-show in AngularJS
Part 15 - AngularJS ng-init directive
Part 16 - ng-include directive in AngularJS
In this video we will discuss how to consume an ASP.NET Web Service in an AngularJS application. Let us understand this step by step from creating an ASP.NET web service to consuming it in an Angular application.
Here is what we want to do
1. Create an ASP.NET Web service. This web service retrieves the data from SQL Server database table, returns it in JSON formt.
2. Call the web service using AngularJS and display employee data on the web page
Step 1 : Create SQL Server table and insert employee data
Step 2 : Create new empty asp.net web application project. Name it Demo.
Step 3 : Include the following settings in web.config file.
Step 4 : Add a class file to the project. Name it Employee.cs. Copy and paste the following code.
Step 5 : Add a new WebService (ASMX). Name it EmployeeService.asmx. Copy and paste the following code.
Step 6 : Add a new folder to the project. Name it Scripts. Download angular.js script file from http://angularjs.org, and past it in Scripts folder.
Step 7 : Add a new JavaScript file to the Scripts folder. Name it Script.js. Copy and paste the following code.
Step 8 : Add a new stylesheet to the project. Name it Styles.css. Copy and paste the following styles in it.
Step 9 : Add an HTML page to the ASP.NET project. Copy and paste the following HTML and Angular code
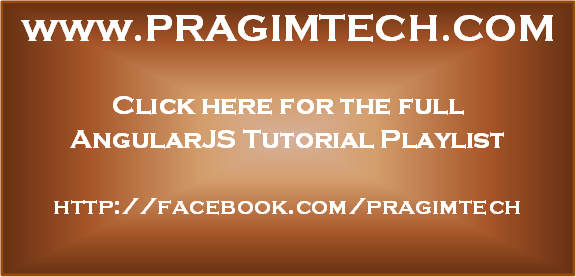
Part 14 - ng-hide and ng-show in AngularJS
Part 15 - AngularJS ng-init directive
Part 16 - ng-include directive in AngularJS
In this video we will discuss how to consume an ASP.NET Web Service in an AngularJS application. Let us understand this step by step from creating an ASP.NET web service to consuming it in an Angular application.
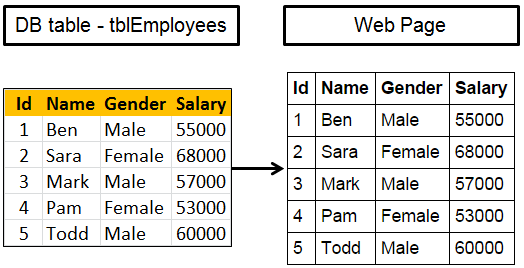
Here is what we want to do
1. Create an ASP.NET Web service. This web service retrieves the data from SQL Server database table, returns it in JSON formt.
2. Call the web service using AngularJS and display employee data on the web page
Step 1 : Create SQL Server table and insert employee data
Create table tblEmployees
(
Id int primary
key identity,
Name nvarchar(50),
Gender nvarchar(10),
Salary int
)
Go
Insert into tblEmployees values ('Ben', 'Male', 55000)
Insert into tblEmployees values ('Sara', 'Female', 68000)
Insert into tblEmployees values ('Mark', 'Male', 57000)
Insert into tblEmployees values ('Pam', 'Female', 53000)
Insert into tblEmployees values ('Todd', 'Male', 60000)
Go
Step 2 : Create new empty asp.net web application project. Name it Demo.
Step 3 : Include the following settings in web.config file.
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<connectionStrings>
<add name="DBCS"
connectionString="server=.;database=SampleDB; integrated security=SSPI"/>
</connectionStrings>
<system.web>
<webServices>
<protocols>
<add name="HttpGet"/>
</protocols>
</webServices>
</system.web>
</configuration>
Step 4 : Add a class file to the project. Name it Employee.cs. Copy and paste the following code.
namespace Demo
{
public class Employee
{
public int id { get; set; }
public string name { get; set; }
public string gender { get; set; }
public int salary { get; set; }
}
}
Step 5 : Add a new WebService (ASMX). Name it EmployeeService.asmx. Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data.SqlClient;
using System.Web.Script.Serialization;
using System.Web.Services;
namespace Demo
{
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class EmployeeService : System.Web.Services.WebService
{
[WebMethod]
public void GetAllEmployees()
{
List<Employee> listEmployees = new List<Employee>();
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("Select * from
tblEmployees", con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Employee employee = new Employee();
employee.id = Convert.ToInt32(rdr["Id"]);
employee.name = rdr["Name"].ToString();
employee.gender = rdr["Gender"].ToString();
employee.salary = Convert.ToInt32(rdr["Salary"]);
listEmployees.Add(employee);
}
}
JavaScriptSerializer js = new JavaScriptSerializer();
Context.Response.Write(js.Serialize(listEmployees));
}
}
}
Step 6 : Add a new folder to the project. Name it Scripts. Download angular.js script file from http://angularjs.org, and past it in Scripts folder.
Step 7 : Add a new JavaScript file to the Scripts folder. Name it Script.js. Copy and paste the following code.
///
<reference path="angular.min.js" />
var app = angular
.module("myModule", [])
.controller("myController",
function
($scope, $http) {
$http.get("EmployeeService.asmx/GetAllEmployees")
.then(function (response) {
$scope.employees =
response.data;
});
});
Step 8 : Add a new stylesheet to the project. Name it Styles.css. Copy and paste the following styles in it.
body {
font-family: Arial;
}
table {
border-collapse: collapse;
}
td {
border: 1px solid black;
padding: 5px;
}
th {
border: 1px solid black;
padding: 5px;
text-align: left;
}
Step 9 : Add an HTML page to the ASP.NET project. Copy and paste the following HTML and Angular code
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title></title>
<script src="Scripts/angular.js"></script>
<script src="Scripts/Script.js"></script>
<link href="Styles.css" rel="stylesheet" />
</head>
<body ng-app="myModule">
<div ng-controller="myController">
<table>
<thead>
<tr>
<th>Id</th>
<th>Name</th>
<th>Gender</th>
<th>Salary</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="employee in
employees">
<td>{{employee.id}}</td>
<td>{{employee.name}}</td>
<td>{{employee.gender}}</td>
<td>{{employee.salary}}</td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
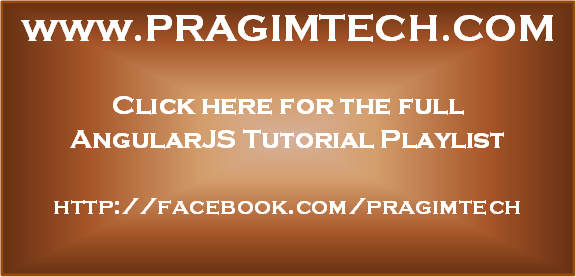
Hi Venkat,May i know? How can we apply validations using angular js.Thank you very much for post angular js tutorials.
ReplyDeletehi pragim can u upload some project based on your tutorials
ReplyDeleteexplain insert , update ,delete also sir by using angularjs with database
ReplyDeleteDear Venkat,
ReplyDeleteThnaks for sharing web service using in Angular js tutorial.
Hii Venkat i am using angularjs with rest api i amunable to bind so please look into my code and correct it
ReplyDeletevar myAngApp = angular.module('SharePointAngApp', []);
alert('sss');
myAngApp.controller('spCustomerController', function ($scope, $http) {
$http({
method: 'Post',
url: appWebUrl + "/_api/SP.AppContextSite(@TargetSite)/web/lists/getByTitle('InfoList')/items?@TargetSite='" + targetSiteUrl + "'",
// url: appWebUrl + "/_api/SP.AppContextSite(@)/web/lists/getByTitle('InfoList')/items?$select=Title,Employee,Company",
headers: { "Accept": "application/json;odata=verbose" }
}).success(function (data, status, headers, config) {
$scope.customers = data.d.results;
}).error(function (data, status, headers, config) {
});
$(document).ready(function () {
var appWebUrl = "";
var targetSiteUrl = "";
var ready = false;
var params = document.URL.split("?")[1].split("&");
for (var i = 0; i < params.length; i = i + 1) {
var param = params[i].split("=");
switch (param[0]) {
case "SPAppWebUrl":
appWebUrl = decodeURIComponent(param[1]);
break;
case "SPHostUrl":
targetSiteUrl = decodeURIComponent(param[1]);
break;
}
}
// load the request executor script, once ready set a flag that can be used to check against later
$.getScript(appWebUrl + "/_layouts/15/SP.RequestExecutor.js", function () {
ready = true;
});
});
});
Hi Venkat,
ReplyDeleteShall you please show a demo of AngularJS Connecting with Database using web API and Rest API (These components are mainly used in real time scenario. Rather than using old asmx).
thank you vekant for such tutorials but it would have been so much helpful for lazy programmers if you provide json output here. :D
ReplyDeleteSo nice of you, i appreciate your way of Explaining Examples
ReplyDeletewonder way to explain the Concept i have ever seen.
ReplyDeleteCan you do an insert, update and delete from this example as well?
ReplyDeleteHi Venkat. Thanks for your very useful tutorials. I am new to programming and I just have finished Html, SQL, CSS and JavaScript. I just have started AngularJs and it is a bit difficult for me, especially this tutorial. What do you recommend to me to help me learn AngularJS better?
ReplyDeleteThanks
hello sir,
ReplyDeletecan u please tell me how to retrieve the data from database using php page and display it using angularjs if u have any such code please share it with me sir
Hello Sir, Thanks for your very useful tutorials. you have any spring mvc based tutorials.
ReplyDeleteHow I can send a params please?
ReplyDeleteHi Venkat could you do a video on insert,update and delete
ReplyDeleteHi Venkat, I am using Visual Studio 2010. I am getting this error:The type or namespace name 'Employee' could not be found (are you missing a using directive or an assembly reference?) Please advise
ReplyDelete