Suggested Videos
Part 11 - Search filter in AngularJS
Part 12 - Angularjs filter by multiple properties
Part 13 - Create a custom filter in AngularJS
In this video we will discuss ng-hide and ng-show directives in Angular with examples
ng-hide and ng-show directives are used to control the visibility of the HTML elements. Let us understand this with an example
When Hide Salary checkbox is checked, the Salary column should be hidden.
When it is unchecked the Salary column should be unhidden
Script.js : The controller function builds the model
HtmlPage1.html : Notice ng-model directive on the checkbox is set to hideSalary. hideSalary variable is then used as the value for ng-hide directive on the th and td elements that displays Salary. When the page is first loaded, hideSalary variable will be undefined which evaluates to false, as a result Salary column will be visible. When the checkbox is checked, hideSalary variable will be attached to the $scope object and true value is stored in it. This value is then used by the ng-hide directive to hide the salary td and it's th element. When the checkbox is unchecked, false value is stored in the hideSalary variable, which is then used by the ng-hide directive to display the Salary column.
With the above example we can also use ng-show directive instead of ng-hide directive. For this example to behave the same as before, we will have to negate the value of hideSalary variable using ! operator.
The following example masks and unmasks the Salary column values using ng-hide and ng-show directives, depending on the checked status of the Hide Salary checkbox.
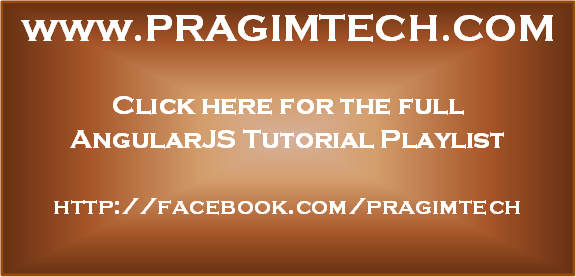
Part 11 - Search filter in AngularJS
Part 12 - Angularjs filter by multiple properties
Part 13 - Create a custom filter in AngularJS
In this video we will discuss ng-hide and ng-show directives in Angular with examples
ng-hide and ng-show directives are used to control the visibility of the HTML elements. Let us understand this with an example
When Hide Salary checkbox is checked, the Salary column should be hidden.

When it is unchecked the Salary column should be unhidden

Script.js : The controller function builds the model
var app = angular
.module("myModule", [])
.controller("myController",
function
($scope) {
var employees = [
{ name: "Ben",
gender: "Male", city: "London", salary: 55000 },
{ name: "Sara",
gender: "Female", city: "Chennai", salary: 68000 },
{ name: "Mark",
gender: "Male", city: "Chicago", salary: 57000 },
{ name: "Pam",
gender: "Female", city: "London", salary: 53000 },
{ name: "Todd", gender:
"Male", city: "Chennai", salary: 60000 }
];
$scope.employees = employees;
});
HtmlPage1.html : Notice ng-model directive on the checkbox is set to hideSalary. hideSalary variable is then used as the value for ng-hide directive on the th and td elements that displays Salary. When the page is first loaded, hideSalary variable will be undefined which evaluates to false, as a result Salary column will be visible. When the checkbox is checked, hideSalary variable will be attached to the $scope object and true value is stored in it. This value is then used by the ng-hide directive to hide the salary td and it's th element. When the checkbox is unchecked, false value is stored in the hideSalary variable, which is then used by the ng-hide directive to display the Salary column.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title></title>
<script src="Scripts/angular.min.js"></script>
<script src="Scripts/Script.js"></script>
<link href="Styles.css" rel="stylesheet" />
</head>
<body ng-app="myModule">
<div ng-controller="myController">
<input type="checkbox" ng-model="hideSalary" />Hide Salary
<br /><br />
<table>
<thead>
<tr>
<th>Name</th>
<th>Gender</th>
<th>City</th>
<th ng-hide="hideSalary">Salary</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="employee in
employees">
<td> {{ employee.name }}
</td>
<td> {{ employee.gender}} </td>
<td> {{ employee.city}} </td>
<td ng-hide="hideSalary"> {{
employee.salary }} </td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
With the above example we can also use ng-show directive instead of ng-hide directive. For this example to behave the same as before, we will have to negate the value of hideSalary variable using ! operator.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title></title>
<script src="Scripts/angular.min.js"></script>
<script src="Scripts/Script.js"></script>
<link href="Styles.css" rel="stylesheet" />
</head>
<body ng-app="myModule">
<div ng-controller="myController">
<input type="checkbox" ng-model="hideSalary" />Hide Salary
<br /><br />
<table>
<thead>
<tr>
<th>Name</th>
<th>Gender</th>
<th>City</th>
<th ng-show="!hideSalary">Salary</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="employee in
employees">
<td> {{ employee.name }}
</td>
<td> {{ employee.gender}} </td>
<td> {{ employee.city}} </td>
<td ng-show="!hideSalary"> {{
employee.salary }} </td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
The following example masks and unmasks the Salary column values using ng-hide and ng-show directives, depending on the checked status of the Hide Salary checkbox.


<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title></title>
<script src="Scripts/angular.min.js"></script>
<script src="Scripts/Script.js"></script>
<link href="Styles.css" rel="stylesheet" />
</head>
<body ng-app="myModule">
<div ng-controller="myController">
<input type="checkbox" ng-model="hideSalary" />Hide Salary
<br /><br />
<table>
<thead>
<tr>
<th>Name</th>
<th>Gender</th>
<th>City</th>
<th ng-hide="hideSalary">Salary</th>
<th ng-show="hideSalary">Salary</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="employee in
employees">
<td> {{ employee.name }}
</td>
<td> {{ employee.gender}} </td>
<td> {{ employee.city}} </td>
<td ng-hide="hideSalary"> {{
employee.salary }} </td>
<td ng-show="hideSalary">
##### </td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
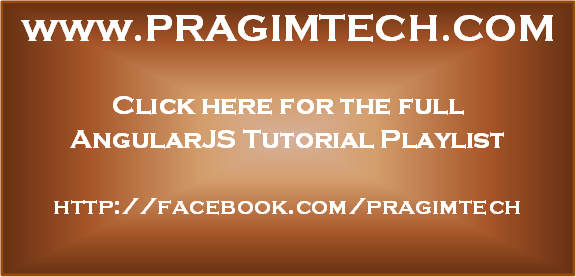
Sir,what should I do to fix the error of focus () method ,as I have been trying set focus onn1st checkbox from three.
ReplyDeleteHi do i have to learn asp .net or other than javascriot-css-html to learn angular ?
ReplyDeletethank you in advance.
hi sir i have gone through upto this video. i have one doubt here suppose if i have more than 1000 column then how we will perform for this operation??
ReplyDeleteHello Venkat Garu,
ReplyDeleteI did same as shown by you with extra , declaring "$scope.hideSalary = false" in controller . in that case the hide and show is not working properly. did i done any thing wrong?.
Thanks
Sithender