Suggested Videos
Part 93 - Draggable element on top
Part 94 - jquery droppable widget
Part 95 - Customizing droppable widget
In this video we will discuss jQuery Resizable Widget
jQuery Resizable Widget allows you to change the size of an element.
Consider the following HTML and CSS
To make the div element resizable
$(document).ready(function () {
$('#redDiv').resizable();
});
jQuery Resizable Widget Options
jQuery Resizable Widget Events
Resizing redDiv will also resize blueDiv
HTML
jQuery
Constrains re-sizing to within the bounds of the container div
HTML
jQuery
$('#redDiv').resizable({
containment: '#container'
});
The following example handles start, stop and resize events of resizable widget
Part 93 - Draggable element on top
Part 94 - jquery droppable widget
Part 95 - Customizing droppable widget
In this video we will discuss jQuery Resizable Widget
jQuery Resizable Widget allows you to change the size of an element.
Consider the following HTML and CSS
<div id="redDiv" class="divClass" style="background-color:
red">
Red Div
</div>
<style>
.divClass {
font-family: Arial;
height: 150px;
width: 150px;
text-align: center;
color: white;
}
</style>
To make the div element resizable
$(document).ready(function () {
$('#redDiv').resizable();
});
jQuery Resizable Widget Options
Option | Description |
alsoResize | Allows to resize one or more elements synchronously with the resizable element |
animate | Animates to the final size after resizing. Include .ui-resizable-helper class to display outline while resizing |
aspectRatio | Specifies whether the element should preserve aspect ratio |
autoHide | Specifies whether the resize handles should hide when the user is not hovering over the element |
containment | Constrains resizing to within the bounds of the specified element or region |
ghost | specifies whether a semi-transparent helper element should be shown for resizing |
maxHeight | The maximum height you can resize to |
minHeight | The minimum height you can resize to |
maxWidth | The maximum width you can resize to |
minWidth | The minimum width you can resize to |
jQuery Resizable Widget Events
Event | Description |
start | Triggered at the start of a resize operation |
stop | Triggered at the end of a resize operation |
resize | Triggered during the resize operation |
Resizing redDiv will also resize blueDiv
HTML
<div id="redDiv" class="divClass" style="background-color:
red">
Red Div
</div>
<br />
<div id="blueDiv" class="divClass" style="background-color:
blue">
Red Div
</div>
jQuery
$(document).ready(function () {
$('#redDiv').resizable({
alsoResize: '#blueDiv'
});
});
Constrains re-sizing to within the bounds of the container div
HTML
<div id="container" style="border: 3px solid black;
height: 300px; width: 300px; padding: 5px">
<div id="redDiv" class="divClass" style="background-color:
red">
Red Div
</div>
</div>
jQuery
$('#redDiv').resizable({
containment: '#container'
});
The following example handles start, stop and resize events of resizable widget
<%@ Page Language="C#" AutoEventWireup="true"
CodeBehind="WebForm1.aspx.cs" Inherits="Demo.WebForm1" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src="jquery-1.11.2.js"></script>
<script src="jquery-ui.js"></script>
<link href="jquery-ui.css" rel="stylesheet" />
<script type="text/javascript">
$(document).ready(function () {
$('#redDiv').resizable({
start: function (event, ui) {
$('#startDimensions').html(getDimensions(event,
ui));
},
stop: function (event, ui) {
$('#stopDimensions').html(getDimensions(event,
ui));
},
resize: function (event, ui) {
$('#resizingDimensions').html(getDimensions(event,
ui));
}
});
function getDimensions(event, ui) {
var html = 'Height = ' + ui.size.height + '<br/>';
html += 'Width = ' + ui.size.width;
return html;
}
});
</script>
<style>
.divClass {
font-family: Arial;
height: 150px;
width: 150px;
text-align: center;
color: white;
}
</style>
</head>
<body style="font-family:
Arial">
<form id="form1" runat="server">
<table border="1" style="border-collapse: collapse;">
<tr>
<td>Dimensions at Start</td>
<td>
<div id="startDimensions"></div>
</td>
</tr>
<tr>
<td>Dimensions while Resizing</td>
<td>
<div id="resizingDimensions"></div>
</td>
</tr>
<tr>
<td>Dimensions at Stop</td>
<td>
<div id="stopDimensions"></div>
</td>
</tr>
</table>
<br />
<div id="redDiv" class="divClass" style="background-color:
red">
Red Div
</div>
</form>
</body>
</html>
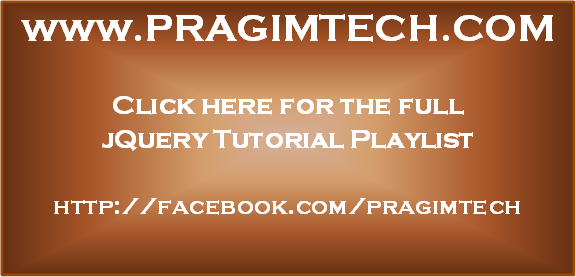
No comments:
Post a Comment
It would be great if you can help share these free resources