Suggested Videos
Part 92 - jquery draggable widget
Part 93 - Draggable element on top
Part 94 - jquery droppable widget
In this video we will discuss customizing droppable widget. This is continuation to Part 94. Please watch Part 94 from jQuery tutorial before proceeding.
The helper option of draggable widget specifies the element that you want to use as a helper while dragging the draggable. This option supports multiple types - string or function.
String: If set to "clone", then the element will be cloned and the clone will be dragged.
Function: A function that will return a DOMElement to use while dragging.
Part 92 - jquery draggable widget
Part 93 - Draggable element on top
Part 94 - jquery droppable widget
In this video we will discuss customizing droppable widget. This is continuation to Part 94. Please watch Part 94 from jQuery tutorial before proceeding.
Option/Event | Description |
over | This event is triggered when an acceptable draggable is dragged over the droppable |
out | This event is triggered when an acceptable draggable is dragged out of the droppable |
activate | This event is triggered when an acceptable draggable starts dragging |
deactivate | This event is triggered when an acceptable draggable stops dragging |
activeClass | The specified class will be applied to the droppable while an acceptable draggable is being dragged |
hoverClass | The specified class will be applied to the droppable while an acceptable draggable is being hovered over the droppable |
The helper option of draggable widget specifies the element that you want to use as a helper while dragging the draggable. This option supports multiple types - string or function.
String: If set to "clone", then the element will be cloned and the clone will be dragged.
Function: A function that will return a DOMElement to use while dragging.
<%@ Page Language="C#" AutoEventWireup="true"
CodeBehind="WebForm1.aspx.cs" Inherits="Demo.WebForm1" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src="jquery-1.11.2.js"></script>
<script src="jquery-ui.js"></script>
<link href="jquery-ui.css" rel="stylesheet" />
<script type="text/javascript">
$(document).ready(function () {
$('#source
li').draggable({
helper: function () {
return '<b><u>' + $(this).text() + '</b></u>';
},
revert: 'invalid'
});
$('#divCountries').droppable({
accept: 'li[data-value="country"]',
//over:
function (event, ui) {
// $(this).addClass('highlight');
//},
//out:
function (event, ui) {
// $(this).removeClass('highlight');
//},
//hoverClass:
'highlight',
//activeClass:
'highlight',
activate: function (event, ui) {
$(this).addClass('highlight');
},
deactivate: function (event, ui) {
$(this).removeClass('highlight');
},
drop: function (event, ui) {
$('#countries').append(ui.draggable);
}
});
$('#divCities').droppable({
accept: 'li[data-value="city"]',
//over:
function (event, ui) {
// $(this).addClass('highlight');
//},
//out:
function (event, ui) {
// $(this).removeClass('highlight');
//},
//hoverClass:
'highlight',
//activeClass:
'highlight',
activate: function (event, ui) {
$(this).addClass('highlight');
},
deactivate: function (event, ui) {
$(this).removeClass('highlight');
},
drop: function (event, ui) {
$('#cities').append(ui.draggable);
}
});
});
</script>
<style>
.divClass {
border: 3px solid black;
font-size: 25px;
background-color: lightgray;
width: 250px;
padding: 5px;
display: inline-table;
}
li {
font-size: 20px;
}
.highlight {
background-color: green;
color: white;
border: 3px solid grey;
}
</style>
</head>
<body style="font-family:
Arial">
<form id="form1" runat="server">
<div class="divClass">
Countries & Cities
<ul id="source">
<li data-value="country">USA</li>
<li data-value="country">India</li>
<li data-value="country">UK</li>
<li data-value="city">New York</li>
<li data-value="city">Chennai</li>
<li data-value="city">London</li>
</ul>
</div>
<div class="divClass" id="divCountries">
Countries
<ul id="countries"></ul>
</div>
<div class="divClass" id="divCities">
Cities
<ul id="cities"></ul>
</div>
</form>
</body>
</html>
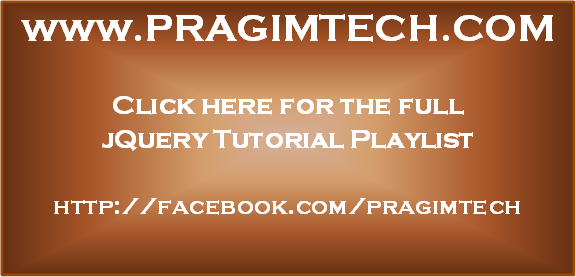
No comments:
Post a Comment
It would be great if you can help share these free resources