Suggested Videos
Part 27 - jQuery map method
Part 28 - Difference between each and map in jquery
Part 29 - jQuery change event
In this video we will discuss jQuery mouse events
If you want to follow along with the example, you will need the image below. Please download the image.
Consider the HTML below
When the mouse is over the help icon, we want to display the help text, when the mouse is out, hide the help text.
To achieve this mouseover and mouseout events can be used as shown below.
$(document).ready(function () {
mouseenter and mouseleave events can also be used.
We can also achieve the same using hover. hover() function accepts two function arguments, one for mouseenter event and one for mouseleave event.
$( selector ).hover( handlerIn, handlerOut )
is shorthand for
$( selector ).mouseenter( handlerIn ).mouseleave( handlerOut )
Part 27 - jQuery map method
Part 28 - Difference between each and map in jquery
Part 29 - jQuery change event
In this video we will discuss jQuery mouse events
If you want to follow along with the example, you will need the image below. Please download the image.

Consider the HTML below
<table>
<tr>
<td>First Name</td>
<td style="vertical-align:middle">
<input id="txtFirstName" type="text" class="inputRequired" />
</td>
<td><img id="imgFirstNameHelp" src="help.png" /></td>
<td><div id="divFirstNameHelp" style="display:none">
First Name as shown in passport</div></td>
</tr>
<tr>
<td>Last Name</td>
<td>
<input id="txtLastName" type="text" class="inputRequired" />
</td>
<td><img id="imgLastNameHelp" src="help.png" /></td>
<td><div id="divLastNameHelp" style="display:none">
Last Name as shown in passport</div></td>
</tr>
<tr>
<td>City</td>
<td>
<select id="ddlCity" class="inputRequired">
<option value="Select">Select</option>
<option value="New
York">New York</option>
<option value="London">London</option>
<option value="Chennai">Chennai</option>
<option value="Sydney">Sydney</option>
</select>
</td>
<td><img id="imgCityHelp" src="help.png" /></td>
<td><div id="divCityHelp" style="display:none">
Your residence city</div></td>
</tr>
<tr>
<td>Favourite Color</td>
<td>
<input id="radioRed" name="color" type="radio" value="Red"
class="inputRequired" />Red
<input id="radioGreen" name="color" type="radio" value="Green"
class="inputRequired" />Green<br />
<input id="radioBlue" name="color" type="radio" value="Blue"
class="inputRequired" />Blue
</td>
<td><img id="imgColorHelp" src="help.png" /></td>
<td><div id="divColorHelp" style="display:none">
Your Favourite Color</div></td>
</tr>
<tr>
<td>Contact Method</td>
<td>
<input id="chkBoxEmail" type="checkbox" value="Email"
class="inputRequired" />Email
<input id="chkBoxPhone" type="checkbox" value="Phone"
class="inputRequired" />Phone<br />
<input id="chkBoxSocialMedia" type="checkbox" value="Social
Media"
class="inputRequired" />Social Media
</td>
<td><img id="imgContactMethodHelp" src="help.png" /></td>
<td><div id="divContactMethodHelp" style="display:none">
How should we contact you</div></td>
</tr>
<tr>
<td>
Comments
</td>
<td>
<textarea id="txtComments" class="inputRequired"></textarea>
</td>
<td><img id="imgCommentsHelp" src="help.png" /></td>
<td><div id="divCommentsHelp" style="display:none">
Your comments please</div></td>
</tr>
<tr>
<td>
<input id="btnSubmit" type="button" value="Submit" />
</td>
<td>
<div id="divResult"></div>
</td>
<td></td>
</tr>
</table>
When the mouse is over the help icon, we want to display the help text, when the mouse is out, hide the help text.

To achieve this mouseover and mouseout events can be used as shown below.
$(document).ready(function () {
$('img[src="help.png"]').mouseover(function () {
$('#' + getDivId(this)).fadeIn(400);
$(this).css('cursor', 'pointer');
}).mouseout(function () {
$('#' + getDivId(this)).fadeOut(400);
});
function getDivId(helpIcon) {
var helpIconId = $(helpIcon).attr('id');
return helpIconId.replace('img', 'div');
}
});
$(document).ready(function () {
$('img[src="help.png"]').mouseenter(function () {
$('#' + getDivId(this)).fadeIn(400);
$(this).css('cursor', 'pointer');
}).mouseleave(function () {
$('#' + getDivId(this)).fadeOut(400);
});
function getDivId(helpIcon) {
var helpIconId = $(helpIcon).attr('id');
return helpIconId.replace('img', 'div');
}
});
We can also achieve the same using hover. hover() function accepts two function arguments, one for mouseenter event and one for mouseleave event.
$( selector ).hover( handlerIn, handlerOut )
is shorthand for
$( selector ).mouseenter( handlerIn ).mouseleave( handlerOut )
$(document).ready(function () {
$('img[src="help.png"]').hover(function () {
$('#' + getDivId(this)).fadeIn(400);
$(this).css('cursor', 'pointer');
}, function () {
$('#' + getDivId(this)).fadeOut(400);
});
function getDivId(helpIcon) {
var helpIconId = $(helpIcon).attr('id');
return helpIconId.replace('img', 'div');
}
});
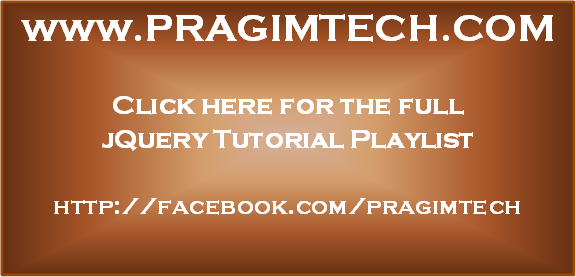
HI, I have been following along this tutorials, the previous video to this one, is there anyway I can stop the code to repeating the item on each click?
ReplyDelete