Suggested Videos
Part 6 - Watermark in ASP.NET TextBox using JavaScript
Part 7 - Disable ASP.NET button after click to prevent double clicking
Part 8 - JavaScript to automatically tab to next textbox
These 2 methods are used to add JavaScript to asp.net pages.
1. RegisterStartupScript
2. RegisterClientScriptBlock
RegisterStartupScript method : This method places the JavaScript at the bottom of the page just before the closing </form> element.
There are 2 overloaded versions of this method.
RegisterStartupScript(Type type, string key, string script)
RegisterStartupScript(Type type, string key, string script, bool addScriptTags)
Type Parameter : The type of the startup script to register. When you register a client script, you cannot use the same key and type. They are considered duplicates. The Type parameter allows us to use the same key value across different types of controls.
Example: In this example, we are using the first overloaded version that has 3 parameters. In this example we are including the the script tags in the script block that we want to register.
ASPX
Code-Behind
If you want the script tags to be generated, instead of hardcoding them in the script block, you can use the second overloaded version that has 4 parameters.
The following is the generated HTML. Notice that JavaScript is placed at the bottom of the page just before the closing </form> element.
RegisterClientScriptBlock method : This method is very similar to RegisterStartupScript method, except that this method places JavaScript after the opening <form> element in the page but before the HTML elements are loaded.
The following is the generated HTML. Notice that JavaScript is placed just after the opening <form> element in the page.
What is the difference between RegisterStartupScript and RegisterClientScriptBlock. When to use one over the other
The main difference is that, RegisterStartupScript method places the script at the bottom of the page (after all the elements in the page) just before the closing </form> element. The advantage of this is that by the time the script executes, all the HTML elements are already loaded. This means the script can reference and HTML element without NULL reference errors.
The following example works because the script is placed on the page just befor the closing </form> element. So the script finds the referenced element with id=Label1
ASPX : <asp:Label ID="Label1" runat="server"></asp:Label>
Code-Behind
However, if you use RegisterClientScriptBlock method you will get a null reference error. This is because RegisterClientScriptBlock method places the script before the HTML elements on the page, so by the time the script is executed the HTML elements are not loaded and hence we get a NULL reference error.
In summary here are the differences
1. RegisterStartupScript method places JavaScript just before the closing </form> element i.e after all the HTML elements. RegisterClientScriptBlock method places JavaScript after opening <form> element i.e before all the HTML elements.
2. Use RegisterClientScriptBlock() method for scripts encapsulated in functions and that you don't need to run on page load. RegisterStartupScript() method can be used for any script, even if it's not inside a function. Generally RegisterStartupScript() method is used for scripts that must run on page load.
Page.RegisterStartupScript and Page.RegisterClientScriptBlock methods are obsolete, instead use ClientScript.RegisterStartupScript and ClientScript.RegisterClientScriptBlock methods.
Part 6 - Watermark in ASP.NET TextBox using JavaScript
Part 7 - Disable ASP.NET button after click to prevent double clicking
Part 8 - JavaScript to automatically tab to next textbox
These 2 methods are used to add JavaScript to asp.net pages.
1. RegisterStartupScript
2. RegisterClientScriptBlock
RegisterStartupScript method : This method places the JavaScript at the bottom of the page just before the closing </form> element.
There are 2 overloaded versions of this method.
RegisterStartupScript(Type type, string key, string script)
RegisterStartupScript(Type type, string key, string script, bool addScriptTags)
Type Parameter : The type of the startup script to register. When you register a client script, you cannot use the same key and type. They are considered duplicates. The Type parameter allows us to use the same key value across different types of controls.
Example: In this example, we are using the first overloaded version that has 3 parameters. In this example we are including the the script tags in the script block that we want to register.
ASPX
<asp:TextBox ID="TextBox1" runat="server" onkeyup="getCharacterCount()">
</asp:TextBox>
<asp:Label ID="Label1" runat="server"></asp:Label>
Code-Behind
protected void Page_Load(object sender, EventArgs
e)
{
ClientScript.RegisterStartupScript(this.GetType(), "clientScript",
"<script
type=\"text/javascript\" language=\"javascript\">" +
"function getCharacterCount() {
document.getElementById('Label1').innerHTML" +
"=
document.getElementById('TextBox1').value.length; } </script>");
}
If you want the script tags to be generated, instead of hardcoding them in the script block, you can use the second overloaded version that has 4 parameters.
protected void Page_Load(object sender, EventArgs
e)
{
ClientScript.RegisterStartupScript(this.GetType(), "clientScript",
"function getCharacterCount() {
document.getElementById('Label1').innerHTML" +
"=
document.getElementById('TextBox1').value.length; }", true);
}
The following is the generated HTML. Notice that JavaScript is placed at the bottom of the page just before the closing </form> element.
<html><head><title></title></head>
<body style="font-family: Arial">
<form method="post" action="WebForm1.aspx" id="form1">
<div class="aspNetHidden">
<input type="hidden" id="__VIEWSTATE" value="..." />
</div>
<div class="aspNetHidden">
<input type="hidden" id="__EVENTVALIDATION" value="..." />
</div>
<input name="TextBox1" type="text" id="TextBox1" onkeyup="getCharacterCount()" />
<span id="Label1"></span>
<script type="text/javascript" language="javascript">
function getCharacterCount()
{
document.getElementById('Label1').innerHTML =
document.getElementById('TextBox1').value.length;
}
</script>
</form>
</body>
</html>
RegisterClientScriptBlock method : This method is very similar to RegisterStartupScript method, except that this method places JavaScript after the opening <form> element in the page but before the HTML elements are loaded.
protected void Page_Load(object sender, EventArgs
e)
{
ClientScript.RegisterClientScriptBlock(this.GetType(), "clientScript",
"<script
type=\"text/javascript\" language=\"javascript\">" +
"function getCharacterCount() { document.getElementById('Label1').innerHTML" +
"=
document.getElementById('TextBox1').value.length; } </script>");
}
The following is the generated HTML. Notice that JavaScript is placed just after the opening <form> element in the page.
<html><head><title></title></head>
<body style="font-family: Arial">
<form method="post" action="WebForm1.aspx" id="form1">
<div class="aspNetHidden">
<input type="hidden" id="__VIEWSTATE" value="..." />
</div>
<script type="text/javascript" language="javascript">
function getCharacterCount()
{
document.getElementById('Label1').innerHTML =
document.getElementById('TextBox1').value.length;
}
</script>
<div class="aspNetHidden">
<input type="hidden" id="__EVENTVALIDATION" value="..." />
</div>
<input name="TextBox1" type="text" id="TextBox1"
onkeyup="getCharacterCount()" />
<span id="Label1"></span>
</form>
</body>
</html>
What is the difference between RegisterStartupScript and RegisterClientScriptBlock. When to use one over the other
The main difference is that, RegisterStartupScript method places the script at the bottom of the page (after all the elements in the page) just before the closing </form> element. The advantage of this is that by the time the script executes, all the HTML elements are already loaded. This means the script can reference and HTML element without NULL reference errors.
The following example works because the script is placed on the page just befor the closing </form> element. So the script finds the referenced element with id=Label1
ASPX : <asp:Label ID="Label1" runat="server"></asp:Label>
Code-Behind
protected void Page_Load(object sender, EventArgs
e)
{
ClientScript.RegisterStartupScript(this.GetType(), "clientScript",
"document.getElementById('Label1').innerHTML
= new Date();",
true);
}
However, if you use RegisterClientScriptBlock method you will get a null reference error. This is because RegisterClientScriptBlock method places the script before the HTML elements on the page, so by the time the script is executed the HTML elements are not loaded and hence we get a NULL reference error.
protected void Page_Load(object sender, EventArgs
e)
{
ClientScript.RegisterClientScriptBlock(this.GetType(), "clientScript",
"document.getElementById('Label1').innerHTML
= new Date();",
true);
}
In summary here are the differences
1. RegisterStartupScript method places JavaScript just before the closing </form> element i.e after all the HTML elements. RegisterClientScriptBlock method places JavaScript after opening <form> element i.e before all the HTML elements.
2. Use RegisterClientScriptBlock() method for scripts encapsulated in functions and that you don't need to run on page load. RegisterStartupScript() method can be used for any script, even if it's not inside a function. Generally RegisterStartupScript() method is used for scripts that must run on page load.
Page.RegisterStartupScript and Page.RegisterClientScriptBlock methods are obsolete, instead use ClientScript.RegisterStartupScript and ClientScript.RegisterClientScriptBlock methods.
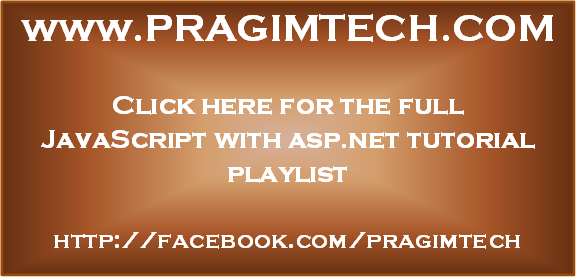
No comments:
Post a Comment
It would be great if you can help share these free resources