Suggested Videos
Part 4 - Change gridview row color when checkbox is checked
Part 5 - ASP.NET TextBox and JavaScript
Part 6 - Watermark in ASP.NET TextBox using JavaScript
In this video we will discuss how to disable ASP.NET button after click to prevent double clicking. Preventing accidental double clicks on a submit button is very important for applications. If double clicks are not prevented you may end up executing the submit button code twice. This means if you are inserting data, duplicate rows may be inserted in the database table.
Let us understand this with an example : The following asp.net web page inserts Name and Gender of an employee to Employees table.
At the moment, after clicking the Sumbit button once it does not get disabled. So there is a chance use can double click. If the double click occurs then we end up with 2 rows in Employees table.
What we want to do is disable the Submit button on first click and display a message saying Please wait...
To disable the submit button after the first click, use OnClientClick and UseSubmitBehavior attributes of the Button control
When the click event occurs, the JavaScript that is associated with the client click event disables the Submit button and displays "Please wait..." message. After the server has processed the request, the page will be reloaded in the client browser, and the Submit button becomes enabled.
Why should we set UseSubmitBehavior to false
When UseSubmitBehavior is set to false, ASP.NET injects client side script to postback the page to the server when click event occurs. With UseSubmitBehavior="false", when you view the page source, the following is the HTML that is generated.
Notice that __doPostBack() method is injected by ASP.NET. This is the method that causes the page to postback when you click the button. If you don't set UseSubmitBehavior="false" on the button, __doPostBack() method is not injected by ASP.NET. This means when you click the button the page will not be posted to the server. Here is the HTML that is generated without UseSubmitBehavior="false"
Disabling ASP.NET ImageButton to prevent double click
1. Add a folder with name=Images to your project. Copy the following 2 images into this folder.
2. Include the following ImageButton declaration in the ASPX page
3. Copy and paste the following code in Page_Load() event in the code-behind file. GetPostBackEventReference() method generates __doPostBack() method that causes the postback to occur when the ImageButton is clicked.
SQL, HTML & Service side code used in the demo
SQL to create Employees table
Insert Stored Procedure
Web.config connection string
WebForm1.aspx
using declarations on WebForm1.aspx.cs
WebForm1.aspx.cs
Part 4 - Change gridview row color when checkbox is checked
Part 5 - ASP.NET TextBox and JavaScript
Part 6 - Watermark in ASP.NET TextBox using JavaScript
In this video we will discuss how to disable ASP.NET button after click to prevent double clicking. Preventing accidental double clicks on a submit button is very important for applications. If double clicks are not prevented you may end up executing the submit button code twice. This means if you are inserting data, duplicate rows may be inserted in the database table.
Let us understand this with an example : The following asp.net web page inserts Name and Gender of an employee to Employees table.

At the moment, after clicking the Sumbit button once it does not get disabled. So there is a chance use can double click. If the double click occurs then we end up with 2 rows in Employees table.

What we want to do is disable the Submit button on first click and display a message saying Please wait...
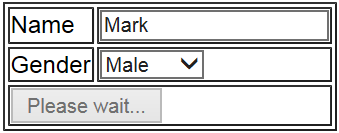
To disable the submit button after the first click, use OnClientClick and UseSubmitBehavior attributes of the Button control
<asp:Button ID="btnSubmit" runat="server" Text="Submit" OnClick="btnSubmit_Click"
UseSubmitBehavior="false"
OnClientClick="this.disabled='true';
this.value='Please wait...';" />
When the click event occurs, the JavaScript that is associated with the client click event disables the Submit button and displays "Please wait..." message. After the server has processed the request, the page will be reloaded in the client browser, and the Submit button becomes enabled.
Why should we set UseSubmitBehavior to false
When UseSubmitBehavior is set to false, ASP.NET injects client side script to postback the page to the server when click event occurs. With UseSubmitBehavior="false", when you view the page source, the following is the HTML that is generated.
<input id="Button1" type="button" name="btnSubmit" value="Submit" onclick="this.disabled='true';
this.value='Please
wait...';__doPostBack('btnSubmit','')"
/>
Notice that __doPostBack() method is injected by ASP.NET. This is the method that causes the page to postback when you click the button. If you don't set UseSubmitBehavior="false" on the button, __doPostBack() method is not injected by ASP.NET. This means when you click the button the page will not be posted to the server. Here is the HTML that is generated without UseSubmitBehavior="false"
<input id="Submit1" type="submit" name="btnSubmit" value="Submit" onclick="this.disabled='true'; this.value='Please
wait...';" />
Disabling ASP.NET ImageButton to prevent double click
1. Add a folder with name=Images to your project. Copy the following 2 images into this folder.


2. Include the following ImageButton declaration in the ASPX page
<asp:ImageButton ID="ImageButton2" runat="server" ImageUrl="Images/submit.png" OnClick="ImageButton1_Click" />
3. Copy and paste the following code in Page_Load() event in the code-behind file. GetPostBackEventReference() method generates __doPostBack() method that causes the postback to occur when the ImageButton is clicked.
protected void Page_Load(object sender, EventArgs
e)
{
ImageButton1.Attributes.Add("onclick", "this.disabled = 'true';"
+ ClientScript.GetPostBackEventReference(ImageButton1,
null)
+ ";this.src
= 'Images/wait.png';");
}
SQL, HTML & Service side code used in the demo
SQL to create Employees table
Create Database SampleDB
GO
Use SampleDB
GO
Create Table Employees
(
ID int identity
primary key,
Name nvarchar(100),
Gender nvarchar(10)
)
GO
Insert Stored Procedure
Create procedure spInsertEmployee
@Name nvarchar(100),
@Gender nvarchar(10)
as
Begin
Insert into
Employees values (@Name, @Gender)
End
Web.config connection string
<add name="SampleDBConnectionString" connectionString="Data
Source=(local);Initial Catalog=SampleDB;Integrated Security=True" providerName="System.Data.SqlClient" />
WebForm1.aspx
<table border="1" >
<tr>
<td>Name</td>
<td>
<asp:TextBox ID="txtName" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>Gender</td>
<td>
<asp:DropDownList ID="ddlGender" runat="server">
<asp:ListItem Text="Male" Value="Male"></asp:ListItem>
<asp:ListItem Text="Female" Value="Female"></asp:ListItem>
</asp:DropDownList>
</td>
</tr>
<tr>
<td colspan="2">
<%--<asp:Button
ID="btnSubmit" runat="server" Text="Submit"
OnClick="btnSubmit_Click"
UseSubmitBehavior="false"
OnClientClick="this.disabled='true'; this.value='Please
wait...';" />--%>
<asp:ImageButton ID="ImageButton1" runat="server"
ImageUrl="Images/submit.png" OnClick="ImageButton1_Click" />
</td>
</tr>
</table>
using declarations on WebForm1.aspx.cs
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
WebForm1.aspx.cs
protected void Page_Load(object sender, EventArgs
e)
{
ImageButton1.Attributes.Add("onclick", "this.disabled = 'true';"
+ ClientScript.GetPostBackEventReference(ImageButton1,
null)
+ ";this.src
= 'Images/wait.png';");
}
protected void btnSubmit_Click(object sender, EventArgs
e)
{
SaveDataToDataBase();
// To simulate artifical latency
System.Threading.Thread.Sleep(3000);
}
protected void ImageButton1_Click(object sender, ImageClickEventArgs
e)
{
SaveDataToDataBase();
// To simulate artifical latency
System.Threading.Thread.Sleep(3000);
}
private void SaveDataToDataBase()
{
string cs = ConfigurationManager.ConnectionStrings["SampleDBConnectionString"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new
SqlCommand("spInsertEmployee", con);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter nameParameter = new SqlParameter()
{
ParameterName = "@Name",
Value = txtName.Text
};
SqlParameter genderParameter = new SqlParameter()
{
ParameterName = "@Gender",
Value = ddlGender.SelectedValue
};
cmd.Parameters.Add(nameParameter);
cmd.Parameters.Add(genderParameter);
con.Open();
cmd.ExecuteNonQuery();
}
}
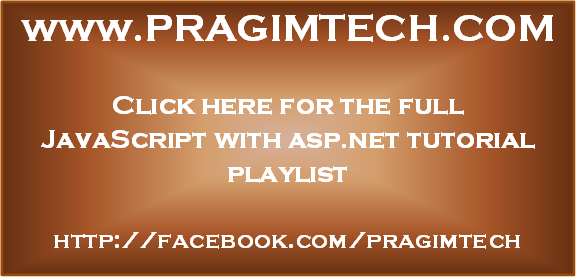
How can i achieve disable button after click to prevent double clicking this in mvc 4
ReplyDeleteSumit Rahul
sumit.rahul@hotmail.com