Suggested Videos
Part 46 - JavaScript popup window
Part 47 - Using regular expressions in JavaScript
Part 48 - Tools for writing regular expressions
In this video we will discuss using regular expressions with some of the JavaScript string object methods.
In JavaScript regular expressions can be used with the following string methods.
1. match()
2. replace()
3. split()
4. search()
Along with regular expressions you can use modifiers to specify the kind of search you want to perform.
Let us look at some examples of using the above methods with regular expressions
Using regular expression with JavaScript string object's match() method. All the numbers in the string will be returned. The letter g at the end of the regular expression performs a global match. If the letter g is omitted we will only get the first match.
var string = "Tom contact number is 1011011010. His age is 35.";
string += "Mark contact number is 8912398912. His age is 45";
document.write(string.match(/\d+/g));
Output : 1011011010,35,8912398912,45
Using regular expression with JavaScript string object's replace() method. All the numbers in the string will be replaced with XXX.
var string = "Tom contact number is 1011011010. His age is 35.";
string += "Mark contact number is 8912398912. His age is 45";
document.write(string.replace(/\d+/g, "XXX"));
Output : Tom contact number is XXX. His age is XXX.Mark contact number is XXX. His age is XXX
Using regular expression with JavaScript string object's split() method. split() method breaks a string into an array of substrings. The following example breaks the string into an array of substrings wherever it finds a number.
var string = "Tom contact number is 1011011010. His age is 35.";
string += "Mark contact number is 8912398912. His age is 45";
document.write(string.split(/\d+/))
Output : Tom contact number is ,. His age is ,.Mark contact number is ,. His age is ,
Using regular expression with JavaScript string object's search() method. search() method returns the index of the match, or -1 if the search item is not found. search() method returns the index of the first matching item only. The following example returns the index of the first occurrence of a number in the string.
var string = "Tom contact number is 1011011010. His age is 35.";
string += "Mark contact number is 8912398912. His age is 45";
document.write(string.search(/\d+/))
Output : 22
Global case insensitive match using a regular expression
var string = "TOM contact number is 1011011010. tom is 35";
document.write(string.match(/tom/gi));
Output : TOM,tom
Part 46 - JavaScript popup window
Part 47 - Using regular expressions in JavaScript
Part 48 - Tools for writing regular expressions
In this video we will discuss using regular expressions with some of the JavaScript string object methods.
In JavaScript regular expressions can be used with the following string methods.
1. match()
2. replace()
3. split()
4. search()
Along with regular expressions you can use modifiers to specify the kind of search you want to perform.

Let us look at some examples of using the above methods with regular expressions
Using regular expression with JavaScript string object's match() method. All the numbers in the string will be returned. The letter g at the end of the regular expression performs a global match. If the letter g is omitted we will only get the first match.
var string = "Tom contact number is 1011011010. His age is 35.";
string += "Mark contact number is 8912398912. His age is 45";
document.write(string.match(/\d+/g));
Output : 1011011010,35,8912398912,45
Using regular expression with JavaScript string object's replace() method. All the numbers in the string will be replaced with XXX.
var string = "Tom contact number is 1011011010. His age is 35.";
string += "Mark contact number is 8912398912. His age is 45";
document.write(string.replace(/\d+/g, "XXX"));
Output : Tom contact number is XXX. His age is XXX.Mark contact number is XXX. His age is XXX
Using regular expression with JavaScript string object's split() method. split() method breaks a string into an array of substrings. The following example breaks the string into an array of substrings wherever it finds a number.
var string = "Tom contact number is 1011011010. His age is 35.";
string += "Mark contact number is 8912398912. His age is 45";
document.write(string.split(/\d+/))
Output : Tom contact number is ,. His age is ,.Mark contact number is ,. His age is ,
Using regular expression with JavaScript string object's search() method. search() method returns the index of the match, or -1 if the search item is not found. search() method returns the index of the first matching item only. The following example returns the index of the first occurrence of a number in the string.
var string = "Tom contact number is 1011011010. His age is 35.";
string += "Mark contact number is 8912398912. His age is 45";
document.write(string.search(/\d+/))
Output : 22
Global case insensitive match using a regular expression
var string = "TOM contact number is 1011011010. tom is 35";
document.write(string.match(/tom/gi));
Output : TOM,tom
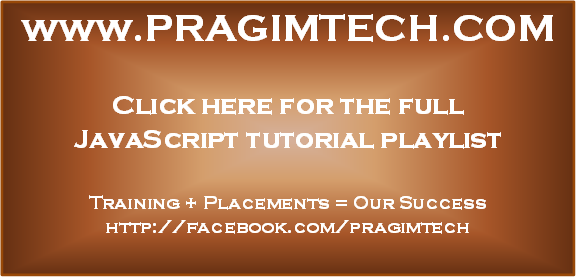
No comments:
Post a Comment
It would be great if you can help share these free resources