Suggested Videos
Part 44 - JavaScript to detect which mouse button is clicked
Part 45 - JavaScript mouse events
Part 46 - JavaScript popup window
What is a Regular Expression
A regular expression is a sequence of characters that forms a search pattern.
Let us understand the use of regular expressions with an example. The following strings contain words and numbers. From the string we want to extract all the numbers. Bsically the program should work with any string.
Mark-9 Tim-890 Sam-10 Sara-9902
Result : 9, 890, 10, 9902
908ABC12XYZ34
Result : 908, 12, 34
$1 $2 $901 ABC(100)
Result : 1, 2, 901, 100
Implement a web page as shown below.
Here is what we want the page to do
1. User enters the string in the first textbox
2. When "Process String" button is clicked, the numbers should be extracted from the string and displayed in the text area element.
It will be very complex and error prone if we have to achieve this without using regular expressions.
Part 44 - JavaScript to detect which mouse button is clicked
Part 45 - JavaScript mouse events
Part 46 - JavaScript popup window
What is a Regular Expression
A regular expression is a sequence of characters that forms a search pattern.
Let us understand the use of regular expressions with an example. The following strings contain words and numbers. From the string we want to extract all the numbers. Bsically the program should work with any string.
Mark-9 Tim-890 Sam-10 Sara-9902
Result : 9, 890, 10, 9902
908ABC12XYZ34
Result : 908, 12, 34
$1 $2 $901 ABC(100)
Result : 1, 2, 901, 100
Implement a web page as shown below.

Here is what we want the page to do
1. User enters the string in the first textbox
2. When "Process String" button is clicked, the numbers should be extracted from the string and displayed in the text area element.
It will be very complex and error prone if we have to achieve this without using regular expressions.
<input type="text" id="txtBox" style="width:250px" />
<br /><br />
<input type="button" value="Process String" onclick="processString()"
style="width:250px" />
<br /><br />
<textarea id="txtArea" rows="4" cols="30"></textarea>
<script type="text/javascript">
function processString()
{
// Clear the textarea element
document.getElementById("txtArea").value = "";
// Retrieve the user intput from the
textbox
var inputString = document.getElementById("txtBox").value;
// Regular expression should be in 2
forward slashes //
// Letter g at the end of the regular
expression performs a global match
// match() method returns all substrings
that match the given regular expression
var result = inputString.match(/\d+/g);
if (result != null)
{
// Add the retrieved numbers to
the textarea element
for (var i = 0; i < result.length;
i++)
{
document.getElementById("txtArea").value += result[i]
+ "\r\n";
}
}
}
</script>
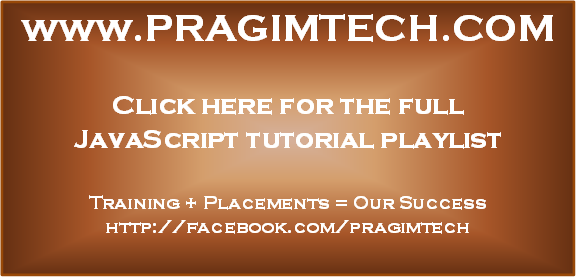
No comments:
Post a Comment
It would be great if you can help share these free resources