Suggested Videos
Part 35 - How to create image slideshow using JavaScript
Part 36 - Events in JavaScript
Part 37 - Assigning event handlers in JavaScript using DOM object property
In this video we will discuss assigning event handlers in JavaScript using the following special methods
addEventListener
removeEventListener
attachEvent
detachEvent
Internet Explorer 9 (and later versions) & all the other modern browsers support addEventListener() and removeEventListener() methods.
Sytnax for assigning event handler using addEventListener() method
element.addEventListener(event, handler, phase)
Sytnax for removing event handler using removeEventListener() method
element.removeEventListener(event, handler, phase)
Please note : The third parameter phase is usually set to false as it is not used.
Example : In this example, we are passing values for all the 3 parameters including phase.
Since the third parameter "phase" is optional you can omit it if you wish.
btn.addEventListener("mouseover", changeColorOnMouseOver);
btn.addEventListener("mouseout", changeColorOnMouseOut);
Example : This example demonstrates removing event handlers.
Using this approach you can assign more than one event handler method to a given event. The order in which handler methods are executed is not defined. In this example, 2 event handler methods (clickHandler1 & clickHandler3) are assigned to click event of the button control. When you click the button both the handler methods are executed.
attachEvent() and detachEvent() methods only work in Internet Explorer 8 and earlier versions.
Sytnax for assigning event handler using attachEvent() method
element.attachEvent( "on"+event, handler)
Sytnax for removing event handler using detachEvent() method
element.detachEvent( "on"+event, handler)
Example : This example will only work in Internet Explorer 8 and earlier versions.
Cross browser solution : For the above example to work in all browsers, modify the script as shown below.
Part 35 - How to create image slideshow using JavaScript
Part 36 - Events in JavaScript
Part 37 - Assigning event handlers in JavaScript using DOM object property
In this video we will discuss assigning event handlers in JavaScript using the following special methods
addEventListener
removeEventListener
attachEvent
detachEvent
Internet Explorer 9 (and later versions) & all the other modern browsers support addEventListener() and removeEventListener() methods.
Sytnax for assigning event handler using addEventListener() method
element.addEventListener(event, handler, phase)
Sytnax for removing event handler using removeEventListener() method
element.removeEventListener(event, handler, phase)
Please note : The third parameter phase is usually set to false as it is not used.
Example : In this example, we are passing values for all the 3 parameters including phase.
<input type="button" value="Click me" id="btn"/>
<script type="text/javascript">
btn.addEventListener("mouseover", changeColorOnMouseOver,
false);
btn.addEventListener("mouseout", changeColorOnMouseOut,
false);
function changeColorOnMouseOver()
{
this.style.background = 'red';
this.style.color = 'yellow';
}
function changeColorOnMouseOut()
{
this.style.background = 'black';
this.style.color = 'white';
}
</script>
Since the third parameter "phase" is optional you can omit it if you wish.
btn.addEventListener("mouseover", changeColorOnMouseOver);
btn.addEventListener("mouseout", changeColorOnMouseOut);
Example : This example demonstrates removing event handlers.
<input type="button" value="Click me" id="btn"/>
<input type="button" value="Remove Event Handlers"
onclick="removeEventHandlers()" />
<script type="text/javascript">
btn.addEventListener("mouseover", changeColorOnMouseOver);
btn.addEventListener("mouseout", changeColorOnMouseOut);
function changeColorOnMouseOver()
{
this.style.background = 'red';
this.style.color = 'yellow';
}
function changeColorOnMouseOut()
{
this.style.background = 'black';
this.style.color = 'white';
}
function removeEventHandlers()
{
btn.removeEventListener("mouseover", changeColorOnMouseOver);
btn.removeEventListener("mouseout", changeColorOnMouseOut);
}
</script>
Using this approach you can assign more than one event handler method to a given event. The order in which handler methods are executed is not defined. In this example, 2 event handler methods (clickHandler1 & clickHandler3) are assigned to click event of the button control. When you click the button both the handler methods are executed.
<input type="button" value="Click me" id="btn"/>
<script type="text/javascript">
btn.addEventListener("click", clickHandler1);
btn.addEventListener("click", clickHandler2);
function clickHandler1()
{
alert("Handler
1");
}
function clickHandler2()
{
alert("Handler
2");
}
</script>
attachEvent() and detachEvent() methods only work in Internet Explorer 8 and earlier versions.
Sytnax for assigning event handler using attachEvent() method
element.attachEvent( "on"+event, handler)
Sytnax for removing event handler using detachEvent() method
element.detachEvent( "on"+event, handler)
Example : This example will only work in Internet Explorer 8 and earlier versions.
<input type="button" value="Click me" id="btn"/>
<script type="text/javascript">
btn.attachEvent("onclick",
clickEventHandler);
function clickEventHandler()
{
alert("Click
Handler");
}
</script>
Cross browser solution : For the above example to work in all browsers, modify the script as shown below.
<input type="button" value="Click me" id="btn"/>
<script type="text/javascript">
if (btn.addEventListener)
{
btn.addEventListener("click", clickEventHandler);
}
else
{
btn.attachEvent("onclick",
clickEventHandler);
}
function clickEventHandler()
{
alert("Click
Handler");
}
</script>
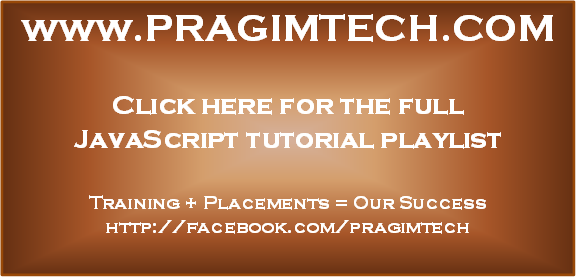
No comments:
Post a Comment
It would be great if you can help share these free resources