Suggested Videos
Part 1 - LINQ to SQL
Part 2 - Insert Update Delete using LINQ to SQL
In this video we will discuss, how to view the SQL statements generated by LINQ to SQL. For debugging, it is necessary to view the generated SQL statements. There are several ways to do this.
Using the Log property of the DataContext object
Using ToString() method
Using GetCommand() method of DataContext object
Finally, we can also use SQL profiler.
Part 1 - LINQ to SQL
Part 2 - Insert Update Delete using LINQ to SQL
In this video we will discuss, how to view the SQL statements generated by LINQ to SQL. For debugging, it is necessary to view the generated SQL statements. There are several ways to do this.
Using the Log property of the DataContext object
using (SampleDataContext dbContext = new SampleDataContext())
{
// Write the generated sql query to the
webform
dbContext.Log = Response.Output;
// Write the generated sql query to the
Console window
// dbContext.Log = Console.Out;
var linqQuery = from employee
in dbContext.Employees
select employee;
GridView1.DataSource = linqQuery;
GridView1.DataBind();
}
Using ToString() method
using (SampleDataContext dbContext = new SampleDataContext())
{
var linqQuery = from employee
in dbContext.Employees
select employee;
string sqlQuery = linqQuery.ToString();
Response.Write(sqlQuery);
GridView1.DataSource = linqQuery;
GridView1.DataBind();
}
Using GetCommand() method of DataContext object
using (SampleDataContext dbContext = new SampleDataContext())
{
var linqQuery = from employee
in dbContext.Employees
select employee;
Response.Write(dbContext.GetCommand(linqQuery).CommandText);
Response.Write("<br/>");
Response.Write(dbContext.GetCommand(linqQuery).CommandType);
GridView1.DataSource = linqQuery;
GridView1.DataBind();
}
Finally, we can also use SQL profiler.
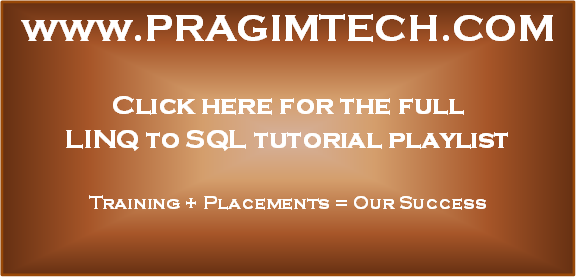
No comments:
Post a Comment
It would be great if you can help share these free resources