Suggested Videos
Part 45 - Reentrant concurrency mode in WCF
Part 46 - WCF throttling
Part 47 - WCF security
In this video we will discuss how to control the WCF message protection using ProtectionLevel parameter.
The following 6 attributes has the ProtectionLevel named parameter, which implies that ProtectionLevel can be specified using any of the below 6 attributes. They are specified in the order of precedence. For example ProtectionLevel specified at an operation contract level overrides the ProtectionLevel specified at the service contract level.
ServiceContractAttribute
OperationContractAttribute
FaultContractAttribute
MessageContractAttribute
MessageHeaderAttribute
MessageBodyMemberAttribute
ProtectionLevel specified on the ServiceContract will be applicable for all the operation contracts within that service contract. ProtectionLevel specified on the OperationContract will be applicable for just that OperationContract. If we specify ProtectionLevel on a MessageContract, then that protection level will be applicable for all messages of that MessageContract type.
When we use wsHttpBinding, by default the messages are encrypted and signed. Encryption provides confidentiality and signing provides integrity. To customize the level of message protection use ProtectionLevel parameter.
Please Note: ProtectionLevel enum is present in System.Net.Security namespace.
ProtectionLevel enum has the following values
None - No protection. Message is not signed and not encrypted. Provides only authentication.
Sign - No encryption but is digitally signed to ensure the integrity of the message
EncryptAndSign - Message is encrypted and then signed to ensure confidentiality and integrity of the message
Let's understand using ProtectionLevel parameter with an example.
Step 1: Create a class library project with name = HelloService. Delete the autogenerated class1.cs file.
Step 2: Right click on HelloService project in solution explorer and add a WCF service with name = HelloService
Step 3: Copy and paste the following code in IHellotService.cs file
Step 4: Copy and paste the following code in HelloService.cs file
Step 5: Right click on HelloService solution in solution explorer and add a console project with name = Host. We will use this project to host the WCF service.
Step 6: Right click on References folder under Host project and add a reference to HelloService project and System.ServiceModel assembly.
Step 7: Right click on Host project and add Application Configuration file. This should add App.config file.
Step 8: Copy and paste the following configuration in App.config file
Step 9: Copy and paste the following code in Program.cs file in the Host project.
Step 10: Set Host project as the startup project and run the application by pressing CTRL + F5 key. At this point we have the WCF service up and running.
Step 11: Now let's create a client for the WCF service. Create a new windows forms application with name = WindowsClient
Step 12: Add a service reference to HelloService. Right click on References folder and select Add Service Reference. In the Add Service Reference window type Address = http://localhost:8080/ and click GO button. This should bring up the HelloService. In the Namespace textbox type HelloService and click OK.
Step 13: Drag and drop 3 button controls on Form1. Set the properties as shown below
For button1
Name = btnGetMessage
Text = Get Message - Not Signed and Not Encrypted
For button2
Name = btnGetSignedMessage
Text = Get Message - Signed but Not Encrypted
For button3
Name = btnGetSignedEncryptedMessage
Text = Get Message - Signed and Encrypted
Step 14: Double click on all of the button controls to generate their respective "click event handler" methods. Copy and paste the following code in Form1.cs file.
Now enable message logging for the WCF Service. We discussed enabling message logging in Part 9 of the WCF tutorial. Run the WCF service and the client and examine the logged messages.
Part 45 - Reentrant concurrency mode in WCF
Part 46 - WCF throttling
Part 47 - WCF security
In this video we will discuss how to control the WCF message protection using ProtectionLevel parameter.
The following 6 attributes has the ProtectionLevel named parameter, which implies that ProtectionLevel can be specified using any of the below 6 attributes. They are specified in the order of precedence. For example ProtectionLevel specified at an operation contract level overrides the ProtectionLevel specified at the service contract level.
ServiceContractAttribute
OperationContractAttribute
FaultContractAttribute
MessageContractAttribute
MessageHeaderAttribute
MessageBodyMemberAttribute
ProtectionLevel specified on the ServiceContract will be applicable for all the operation contracts within that service contract. ProtectionLevel specified on the OperationContract will be applicable for just that OperationContract. If we specify ProtectionLevel on a MessageContract, then that protection level will be applicable for all messages of that MessageContract type.
When we use wsHttpBinding, by default the messages are encrypted and signed. Encryption provides confidentiality and signing provides integrity. To customize the level of message protection use ProtectionLevel parameter.
Please Note: ProtectionLevel enum is present in System.Net.Security namespace.
ProtectionLevel enum has the following values
None - No protection. Message is not signed and not encrypted. Provides only authentication.
Sign - No encryption but is digitally signed to ensure the integrity of the message
EncryptAndSign - Message is encrypted and then signed to ensure confidentiality and integrity of the message
Let's understand using ProtectionLevel parameter with an example.
Step 1: Create a class library project with name = HelloService. Delete the autogenerated class1.cs file.
Step 2: Right click on HelloService project in solution explorer and add a WCF service with name = HelloService
Step 3: Copy and paste the following code in IHellotService.cs file
using System.Net.Security;
using System.ServiceModel;
namespace HelloService
{
[ServiceContract]
public interface IHelloService
{
[OperationContract(ProtectionLevel = ProtectionLevel.None)]
string GetMessageWithoutAnyProtection();
[OperationContract(ProtectionLevel = ProtectionLevel.Sign)]
string GetSignedMessage();
[OperationContract(ProtectionLevel = ProtectionLevel.EncryptAndSign)]
string GetSignedAndEncryptedMessage();
}
}
Step 4: Copy and paste the following code in HelloService.cs file
namespace HelloService
{
public class HelloService : IHelloService
{
public string GetMessageWithoutAnyProtection()
{
return "Message without signature and
encryption";
}
public string GetSignedMessage()
{
return "Message with signature but without
encryption";
}
public string GetSignedAndEncryptedMessage()
{
return "Message signed and encrypted";
}
}
}
Step 5: Right click on HelloService solution in solution explorer and add a console project with name = Host. We will use this project to host the WCF service.
Step 6: Right click on References folder under Host project and add a reference to HelloService project and System.ServiceModel assembly.
Step 7: Right click on Host project and add Application Configuration file. This should add App.config file.
Step 8: Copy and paste the following configuration in App.config file
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<system.serviceModel>
<behaviors>
<serviceBehaviors>
<behavior name="mexBehavior">
<serviceMetadata httpGetEnabled="true" />
</behavior>
</serviceBehaviors>
</behaviors>
<services>
<service behaviorConfiguration="mexBehavior"
name="HelloService.HelloService">
<endpoint address="HelloService"
binding="wsHttpBinding"
contract="HelloService.IHelloService"/>
<host>
<baseAddresses>
<add baseAddress="http://localhost:8080" />
</baseAddresses>
</host>
</service>
</services>
</system.serviceModel>
</configuration>
Step 9: Copy and paste the following code in Program.cs file in the Host project.
using System.ServiceModel;
using System;
namespace Host
{
class Program
{
public static void Main()
{
using (ServiceHost host = new ServiceHost(typeof(HelloService.HelloService)))
{
host.Open();
Console.WriteLine("Host started @ " + DateTime.Now.ToString());
Console.ReadLine();
}
}
}
}
Step 10: Set Host project as the startup project and run the application by pressing CTRL + F5 key. At this point we have the WCF service up and running.
Step 11: Now let's create a client for the WCF service. Create a new windows forms application with name = WindowsClient
Step 12: Add a service reference to HelloService. Right click on References folder and select Add Service Reference. In the Add Service Reference window type Address = http://localhost:8080/ and click GO button. This should bring up the HelloService. In the Namespace textbox type HelloService and click OK.
Step 13: Drag and drop 3 button controls on Form1. Set the properties as shown below
For button1
Name = btnGetMessage
Text = Get Message - Not Signed and Not Encrypted
For button2
Name = btnGetSignedMessage
Text = Get Message - Signed but Not Encrypted
For button3
Name = btnGetSignedEncryptedMessage
Text = Get Message - Signed and Encrypted
Step 14: Double click on all of the button controls to generate their respective "click event handler" methods. Copy and paste the following code in Form1.cs file.
using System;
using System.Windows.Forms;
namespace WindowsClient
{
public partial class
Form1 : Form
{
SimpleService.HelloServiceClient
client;
public Form1()
{
InitializeComponent();
client = new
SimpleService.HelloServiceClient();
}
private void btnGetMessage_Click(object sender, EventArgs
e)
{
MessageBox.Show(client.GetMessageWithoutAnyProtection());
}
private void btnGetSignedMessage_Click
(object sender, EventArgs
e)
{
MessageBox.Show(client.GetSignedMessage());
}
private void btnGetSignedEncryptedMessage_Click
(object sender, EventArgs
e)
{
MessageBox.Show(client.GetSignedAndEncryptedMessage());
}
}
}
Now enable message logging for the WCF Service. We discussed enabling message logging in Part 9 of the WCF tutorial. Run the WCF service and the client and examine the logged messages.
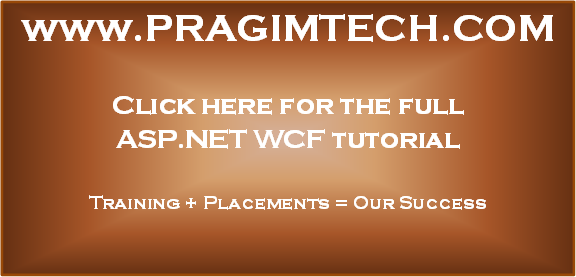
Hi SIR
ReplyDeleteGreat Lectures and Great Effort....
Please give details lectures on jquery ajax jason
Sir, I'm from Lahore, Pakistan and a regular subscriber of your lecs.....
the lec given by you on this blogspot are very helpful in my carrier as .dot net dev. and ALSO SIR your lecs are priceless SIR....
So, Thnx SIR