Suggested Videos
Part 43 - Single concurrency mode in WCF
Part 44 - Multiple concurrency mode in WCF
Part 45 - Reentrant concurrency mode in WCF
In this video we will discuss throttling in WCF with examples.
Let's understand throttling with an example
Step 1: Create a class library project with name = SimpleService. Delete the autogenerated class1.cs file.
Step 2: Right click on SimpleService project in solution explorer and add a WCF service with name = SimpleService.
Step 3: Copy and paste the following code in ISimpleService.cs file
Step 4: Copy and paste the following code in SimpleService.cs file
Step 5: Right click on SimpleService solution in solution explorer and add a console project with name = Host. We will use this project to host the WCF service.
Step 6: Right click on References folder under Host project and add a reference to SimpleService project and System.ServiceModel assembly.
Step 7: Right click on Host project and add Application Configuration file. This should add App.config file.
Step 8: Copy and paste the following configuration in App.config file
Step 9: Copy and paste the following code in Program.cs file in the Host project.
Step 10: Set Host project as the startup project and run the application by pressing CTRL + F5 key. At this point we have the WCF service up and running.
Step 11: Now let's create a client for the WCF service. Create a new Console application with name = Client
Step 12: Add a service reference to SimpleService. Right click on References folder and select Add Service Reference. In the Add Service Reference window type Address = http://localhost:8080/ and click GO button. This should bring up the SimpleService. In the Namespace textbox type SimpleService and click OK.
Step 13: Copy and paste the following code in Program.cs file.
What is throughput?
Throughput is the amount of work done in a given time. In addition to Service Instance Context Mode and Concurrency Mode, Throttling settings also influence the throughput of a WCF service.
Throttling settings can be specified either in the config file or in code
Throttling settings in config file
Throttling settings in code
Please Note: ServiceThrottlingBehavior class is present in System.ServiceModel.Description namespace
With the above throttling settings a maximum of 3 concurrent calls are processed.
In addition to maxConcurrentCalls property, maxConcurrentInstances and maxConcurrentSessions may also impact the number of calls processed concurrently.
For example, if we set maxConcurrentCalls="3", maxConcurrentInstances="2", and maxConcurrentSessions="100",
With a PerCall instance context mode, only 2 calls are processed concurrently. This is because every call requires a new service instance, and here we are allowing only a maximum of 2 concurrent instances to be created.
With a Single instance context mode, 3 calls are processed concurrently. This is because with a singleton service there is only one service instance which handles all the requests from all the clients. With singleton maxConcurrentInstances property does not have any influence.
Please note: maxConcurrentSessions is the sum of all types of sessions, that is
1. Application sessions
2. Transport sessions
3. Reliable sessions
4. Secure sessions
Defaults:
Before WCF 4.0
MaxConcurrentCalls: 16
MaxConcurrentSessions: 10
MaxConcurrentInstances: MaxConcurrentCalls + MaxConcurrentSessions (26)
WCF 4.0 and later
MaxConcurrentCalls: 16 * processor count
MaxConcurrentSessions: 100 * processor count
MaxConcurrentInstances: MaxConcurrentCalls + MaxConcurrentSessions
Part 43 - Single concurrency mode in WCF
Part 44 - Multiple concurrency mode in WCF
Part 45 - Reentrant concurrency mode in WCF
In this video we will discuss throttling in WCF with examples.
Let's understand throttling with an example
Step 1: Create a class library project with name = SimpleService. Delete the autogenerated class1.cs file.
Step 2: Right click on SimpleService project in solution explorer and add a WCF service with name = SimpleService.
Step 3: Copy and paste the following code in ISimpleService.cs file
using System.ServiceModel;
namespace SimpleService
{
[ServiceContract]
public interface ISimpleService
{
[OperationContract(IsOneWay = true)]
void DoWork();
}
}
Step 4: Copy and paste the following code in SimpleService.cs file
using System;
using System.ServiceModel;
using System.Threading;
namespace SimpleService
{
[ServiceBehavior(ConcurrencyMode = ConcurrencyMode.Multiple,
InstanceContextMode = InstanceContextMode.PerCall)]
public class SimpleService : ISimpleService
{
public void DoWork()
{
Thread.Sleep(1000);
Console.WriteLine("Thread {0} processing request @ {1}",
Thread.CurrentThread.ManagedThreadId,
DateTime.Now);
}
}
}
Step 5: Right click on SimpleService solution in solution explorer and add a console project with name = Host. We will use this project to host the WCF service.
Step 6: Right click on References folder under Host project and add a reference to SimpleService project and System.ServiceModel assembly.
Step 7: Right click on Host project and add Application Configuration file. This should add App.config file.
Step 8: Copy and paste the following configuration in App.config file
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<system.serviceModel>
<behaviors>
<serviceBehaviors>
<behavior name="mexBehavior">
<serviceMetadata httpGetEnabled="true" />
</behavior>
</serviceBehaviors>
</behaviors>
<services>
<service behaviorConfiguration="mexBehavior"
name="SimpleService.SimpleService">
<endpoint address="SimpleService"
binding="basicHttpBinding"
contract="SimpleService.ISimpleService"
/>
<host>
<baseAddresses>
<add baseAddress="http://localhost:8080" />
</baseAddresses>
</host>
</service>
</services>
</system.serviceModel>
</configuration>
Step 9: Copy and paste the following code in Program.cs file in the Host project.
using System.ServiceModel;
using System;
namespace Host
{
class Program
{
public static void Main()
{
using (ServiceHost host = new
ServiceHost(typeof(SimpleService.SimpleService)))
{
host.Open();
Console.WriteLine("Host started @ " + DateTime.Now.ToString());
Console.ReadLine();
}
}
}
}
Step 10: Set Host project as the startup project and run the application by pressing CTRL + F5 key. At this point we have the WCF service up and running.
Step 11: Now let's create a client for the WCF service. Create a new Console application with name = Client
Step 12: Add a service reference to SimpleService. Right click on References folder and select Add Service Reference. In the Add Service Reference window type Address = http://localhost:8080/ and click GO button. This should bring up the SimpleService. In the Namespace textbox type SimpleService and click OK.
Step 13: Copy and paste the following code in Program.cs file.
using System.Threading;
namespace Client
{
class Program
{
static void Main()
{
SimpleService.SimpleServiceClient
client = new SimpleService.SimpleServiceClient();
for (int i = 1; i <= 100; i++)
{
Thread thread = new Thread(client.DoWork);
thread.Start();
}
}
}
}
What is throughput?
Throughput is the amount of work done in a given time. In addition to Service Instance Context Mode and Concurrency Mode, Throttling settings also influence the throughput of a WCF service.
Throttling settings can be specified either in the config file or in code
Throttling settings in config file
<behaviors>
<serviceBehaviors>
<behavior name="throttlingBehavior">
<serviceThrottling
maxConcurrentCalls="3"
maxConcurrentInstances="3"
maxConcurrentSessions="100"/>
</behavior>
</serviceBehaviors>
</behaviors>
Throttling settings in code
using (ServiceHost host = new ServiceHost(typeof(SimpleService.SimpleService)))
{
ServiceThrottlingBehavior throttlingBehavior = new ServiceThrottlingBehavior
{
MaxConcurrentCalls = 3,
MaxConcurrentInstances = 3,
MaxConcurrentSessions = 100
};
host.Description.Behaviors.Add(throttlingBehavior);
host.Open();
Console.WriteLine("Host
started @ " + DateTime.Now.ToString());
Console.ReadLine();
}
Please Note: ServiceThrottlingBehavior class is present in System.ServiceModel.Description namespace
With the above throttling settings a maximum of 3 concurrent calls are processed.
In addition to maxConcurrentCalls property, maxConcurrentInstances and maxConcurrentSessions may also impact the number of calls processed concurrently.
For example, if we set maxConcurrentCalls="3", maxConcurrentInstances="2", and maxConcurrentSessions="100",
With a PerCall instance context mode, only 2 calls are processed concurrently. This is because every call requires a new service instance, and here we are allowing only a maximum of 2 concurrent instances to be created.
With a Single instance context mode, 3 calls are processed concurrently. This is because with a singleton service there is only one service instance which handles all the requests from all the clients. With singleton maxConcurrentInstances property does not have any influence.
Please note: maxConcurrentSessions is the sum of all types of sessions, that is
1. Application sessions
2. Transport sessions
3. Reliable sessions
4. Secure sessions
Defaults:
Before WCF 4.0
MaxConcurrentCalls: 16
MaxConcurrentSessions: 10
MaxConcurrentInstances: MaxConcurrentCalls + MaxConcurrentSessions (26)
WCF 4.0 and later
MaxConcurrentCalls: 16 * processor count
MaxConcurrentSessions: 100 * processor count
MaxConcurrentInstances: MaxConcurrentCalls + MaxConcurrentSessions
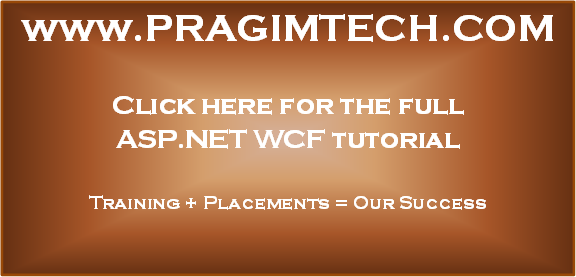
First time i have seen such a nice explanation on throttling.
ReplyDeleteHats Off to you Venket.
thanx for posting.
Hi Venkat,
ReplyDeleteJust wanna say, for you respect comes from the core of my heart!!!.
May God Bless You like you have showered your knowledge on us!!! :) :)