Suggested Videos
Part 21 - Including and excluding properties from model binding using bind attribute
Part 22 - Including and excluding properties from model binding using interfaces
Part 23 - Why deleting database records using get request is bad
In this video we will discuss
1. Deleting database records using POST request
2. Showing the client side javascript confirmation dialog box before deleting
Please watch Part 23, before proceeding.
Step 1: Mark "Delete" action method in "Employee" controller with [HttpPost] attribute. With this change, the "Delete" method will no longer respond to "GET" request. At this point, if we run the application and click on "Delete" link on the "Index" view, we get an error stating - "The resource cannot be found"
[HttpPost]
public ActionResult Delete(int id)
{
EmployeeBusinessLayer employeeBusinessLayer =
new EmployeeBusinessLayer();
employeeBusinessLayer.DeleteEmployee(id);
return RedirectToAction("Index");
}
Step 2: In "Index.cshtml"
REPLACE THE FOLLOWING CODE
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.Gender)
</td>
<td>
@Html.DisplayFor(modelItem => item.City)
</td>
<td>
@Html.DisplayFor(modelItem => item.DateOfBirth)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { id=item.ID }) |
@Html.ActionLink("Details", "Details", new { id=item.ID }) |
@Html.ActionLink("Delete", "Delete", new { id=item.ID })
</td>
</tr>
}
WITH
@foreach (var item in Model)
{
using (Html.BeginForm("Delete", "Employee", new { id = item.ID }))
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.Gender)
</td>
<td>
@Html.DisplayFor(modelItem => item.City)
</td>
<td>
@Html.DisplayFor(modelItem => item.DateOfBirth)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { id = item.ID }) |
<input type="submit" value="Delete" />
</td>
</tr>
}
}
Notice that, we are using "Html.BeginForm()" html helper to generate a form tag.
Step 3: To include client-side confirmation, before the data can be deleted, add the "onclick" attribute to "Delete" button as shown below.
<input type="submit" value="Delete" onclick="return confirm('Are you sure you want to delete record with ID = @item.ID');" />
Part 21 - Including and excluding properties from model binding using bind attribute
Part 22 - Including and excluding properties from model binding using interfaces
Part 23 - Why deleting database records using get request is bad
In this video we will discuss
1. Deleting database records using POST request
2. Showing the client side javascript confirmation dialog box before deleting
Please watch Part 23, before proceeding.
Step 1: Mark "Delete" action method in "Employee" controller with [HttpPost] attribute. With this change, the "Delete" method will no longer respond to "GET" request. At this point, if we run the application and click on "Delete" link on the "Index" view, we get an error stating - "The resource cannot be found"
[HttpPost]
public ActionResult Delete(int id)
{
EmployeeBusinessLayer employeeBusinessLayer =
new EmployeeBusinessLayer();
employeeBusinessLayer.DeleteEmployee(id);
return RedirectToAction("Index");
}
Step 2: In "Index.cshtml"
REPLACE THE FOLLOWING CODE
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.Gender)
</td>
<td>
@Html.DisplayFor(modelItem => item.City)
</td>
<td>
@Html.DisplayFor(modelItem => item.DateOfBirth)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { id=item.ID }) |
@Html.ActionLink("Details", "Details", new { id=item.ID }) |
@Html.ActionLink("Delete", "Delete", new { id=item.ID })
</td>
</tr>
}
WITH
@foreach (var item in Model)
{
using (Html.BeginForm("Delete", "Employee", new { id = item.ID }))
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.Gender)
</td>
<td>
@Html.DisplayFor(modelItem => item.City)
</td>
<td>
@Html.DisplayFor(modelItem => item.DateOfBirth)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { id = item.ID }) |
<input type="submit" value="Delete" />
</td>
</tr>
}
}
Notice that, we are using "Html.BeginForm()" html helper to generate a form tag.
Step 3: To include client-side confirmation, before the data can be deleted, add the "onclick" attribute to "Delete" button as shown below.
<input type="submit" value="Delete" onclick="return confirm('Are you sure you want to delete record with ID = @item.ID');" />
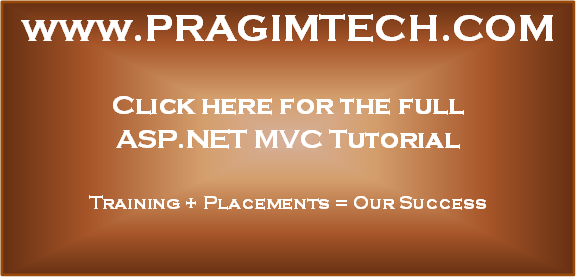
Hi Sir,
ReplyDeleteIn Update method using (Html.BeginForm("Update", "Employee") this will post the form data automatically to controller but while deleting it is not posting automatically. And we have to pass explicitly
using (Html.BeginForm("Delete", "Employee", item)..then only I get the Employee data in controller.
[HttpPost]
public ViewResult Delete(Employee emp)
{
}
Please tell me the reason of this.
Regards.
Its Because,if you observe Beginform() Overload methods,it has 13 overloads, it says ActionName,controllername, routevlaues
Deleteyou should have an "Update" action Name in Employee Controller without any parameters, if your Update Action expects any parameter like ID.. then you need overload ActionName,Controller, RouteValues Method i.e., you need to pass Id to Beginform().
Thanks,
I cut and pasted this line directly from the blog for part 24:
ReplyDelete"
I deliberately misspelled "input" above because your blogger won't accept the HTML tag if input is spelled correctly. I have it spelled correctly in my code.
But I get a bunch of errors, chiefly "Unterminated string constant". If I remove the "@item.ID" so that the line contains no substitutions, it works as expected but then, of course, without the item number. I'm running VS2012. Any suggestions?
I have since discovered that even though VS2012 regards the "onclick" statement above as an error, it is actually only a warning, and the application will run and work as shown in the tutorial.
ReplyDeleteStill, I don't like compiling projects with preventable warnings, so I am open to suggestions for a workaround.
Hi Venkat sir,
ReplyDeleteI was wondering if this code would be considered good or not (I mean using the style attribute on the form like that)
@using (Html.BeginForm("Delete", "Employee", new { id = item.ID }, FormMethod.Post, new { @style="display: inline;" }))
{
<input type="submit" value="Delete" onclick="return confirm('Are you sure you want to delete the employee with ID = @item.ID')" />
}