Suggested Videos
Part 20 - Preventing unintended updates
Part 21 - Including and excluding properties from model binding using bind attribute
Part 22 - Including and excluding properties from model binding using interfaces
In this video we will discuss, why deleting database records using GET request is bad. Please watch Part 22, before proceeding.
First let's discuss, how to delete data in MVC using GET request and then we will discuss, why it is bad to do so.
Step 1: Create a stored procedure to delete employee data by "ID"
Create procedure spDeleteEmployee
@Id int
as
Begin
Delete from tblEmployee
where Id = @Id
End
Step 2: Add the following DeleteEmployee() method to "EmployeeBusinessLayer.cs" file in "BusinessLayer" project. This method calls the stored procedure "spDeleteEmployee" that we just created.
public void DeleteEmployee(int id)
{
string connectionString =
ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(connectionString))
{
SqlCommand cmd = new SqlCommand("spDeleteEmployee", con);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter paramId = new SqlParameter();
paramId.ParameterName = "@Id";
paramId.Value = id;
cmd.Parameters.Add(paramId);
con.Open();
cmd.ExecuteNonQuery();
}
}
Step 3: Add the following "DELETE" controller action method to "EmployeeController".
public ActionResult Delete(int id)
{
EmployeeBusinessLayer employeeBusinessLayer =
new EmployeeBusinessLayer();
employeeBusinessLayer.DeleteEmployee(id);
return RedirectToAction("Index");
}
Run the application and navigate to "Index" action. Click the "Delete" link. This issues "GET" request to the following URL, and deletes the record.
http://localhost/MVCDemo/Employee/Delete/1
Deleting database records using GET request opens a security hole and is not recommended by Microsoft. Just imagine what can happen if there is an image tag in a malicious email as shown below. The moment we open the email, the image tries to load and issues a GET request, which would delete the data.
<img src="http://localhost/MVCDemo/Employee/Delete/2" />
Also, when search engines index your page, they issue a GET request which would delete the data. In general GET request should be free of any side-effects, meaning it should not change the state.
Deletes should always be performed using a POST request. We will discuss, implementing this in our next video.
Part 20 - Preventing unintended updates
Part 21 - Including and excluding properties from model binding using bind attribute
Part 22 - Including and excluding properties from model binding using interfaces
In this video we will discuss, why deleting database records using GET request is bad. Please watch Part 22, before proceeding.
First let's discuss, how to delete data in MVC using GET request and then we will discuss, why it is bad to do so.
Step 1: Create a stored procedure to delete employee data by "ID"
Create procedure spDeleteEmployee
@Id int
as
Begin
Delete from tblEmployee
where Id = @Id
End
Step 2: Add the following DeleteEmployee() method to "EmployeeBusinessLayer.cs" file in "BusinessLayer" project. This method calls the stored procedure "spDeleteEmployee" that we just created.
public void DeleteEmployee(int id)
{
string connectionString =
ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(connectionString))
{
SqlCommand cmd = new SqlCommand("spDeleteEmployee", con);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter paramId = new SqlParameter();
paramId.ParameterName = "@Id";
paramId.Value = id;
cmd.Parameters.Add(paramId);
con.Open();
cmd.ExecuteNonQuery();
}
}
Step 3: Add the following "DELETE" controller action method to "EmployeeController".
public ActionResult Delete(int id)
{
EmployeeBusinessLayer employeeBusinessLayer =
new EmployeeBusinessLayer();
employeeBusinessLayer.DeleteEmployee(id);
return RedirectToAction("Index");
}
Run the application and navigate to "Index" action. Click the "Delete" link. This issues "GET" request to the following URL, and deletes the record.
http://localhost/MVCDemo/Employee/Delete/1
Deleting database records using GET request opens a security hole and is not recommended by Microsoft. Just imagine what can happen if there is an image tag in a malicious email as shown below. The moment we open the email, the image tries to load and issues a GET request, which would delete the data.
<img src="http://localhost/MVCDemo/Employee/Delete/2" />
Also, when search engines index your page, they issue a GET request which would delete the data. In general GET request should be free of any side-effects, meaning it should not change the state.
Deletes should always be performed using a POST request. We will discuss, implementing this in our next video.
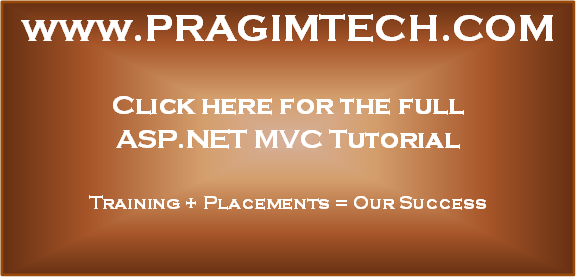
Hello Venkat Sir,
ReplyDeleteYour videos are just awesome!!!!
One question is - As you said deleting database records using get request is bad. And In This video, we are deleting record by using POST Request.
As you have shown in the video - "Part 19 - Unintended updates in mvc", We can make dummy POST Request via fiddler.
So Same we can issue such a POST Request to delete the Record.... So whats the USE then... plz plz answer it...
Eagerly waiting for reply!!!
and THANKS A TON FOR ALL YOUR VIDEOS!!!!!! It helps a LOT....