Suggested Videos
Part 31 - Repository pattern in asp.net core razor pages | Text | Slides
Part 32 - Using stored procedure in entity framework core | Text | Slides
Part 33 - FromSqlRaw vs ExecuteSqlRaw in ASP.NET Core | Text | Slides
In this video we will discuss how to implement CRUD operations (i.e Create, Read, Update and Delete) in ASP.NET core using visual studio scaffolding feature. This scaffolding feature generates the full set of CRUD pages. We can then modify and fine-tune these pages to meet our application specific requirements.
To use the scaffolding tools you need 2 things
ASP.NET Core Scaffolding Tools
In the Solution Explorer, right click on the Pages folder or in a sub-folder in the Pages folder and select - Add - New Scaffolded Item from the context menu.
In the subsequent window, select Razor Pages using Entity Framework (CRUD)
Next, select the Model class and your application DBContext class. Un-check Create a partial view. Finally click the Add button.
Give it a few seconds and the fully functional CRUD pages are generated.
Validation attributes and scaffolding
The code generated by the scaffolding tools will take the validation attributes (if present) into consideration and include the relevant tag helpers on the respective CRUD pages.
You can repeat this process for other model classes (i.e entities) in your application. This scaffolding feature saves lot of time.
Using the scaffolding feature we can also generate API and MVC controllers.
Part 31 - Repository pattern in asp.net core razor pages | Text | Slides
Part 32 - Using stored procedure in entity framework core | Text | Slides
Part 33 - FromSqlRaw vs ExecuteSqlRaw in ASP.NET Core | Text | Slides
In this video we will discuss how to implement CRUD operations (i.e Create, Read, Update and Delete) in ASP.NET core using visual studio scaffolding feature. This scaffolding feature generates the full set of CRUD pages. We can then modify and fine-tune these pages to meet our application specific requirements.
To use the scaffolding tools you need 2 things
- The Model class for which we want to scaffold the CRUD pages and
- The application DBContext class that knows how to connect and work with the data in the underlying database
ASP.NET Core Scaffolding Tools
In the Solution Explorer, right click on the Pages folder or in a sub-folder in the Pages folder and select - Add - New Scaffolded Item from the context menu.
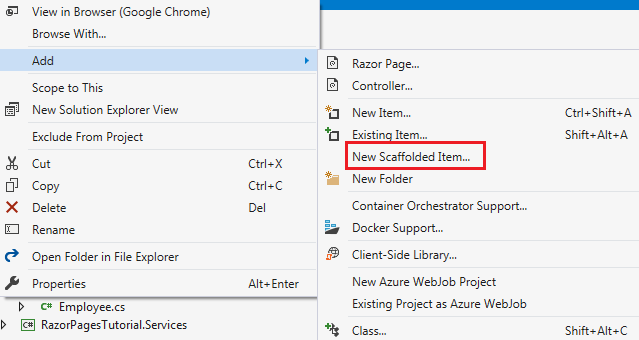
In the subsequent window, select Razor Pages using Entity Framework (CRUD)
Next, select the Model class and your application DBContext class. Un-check Create a partial view. Finally click the Add button.
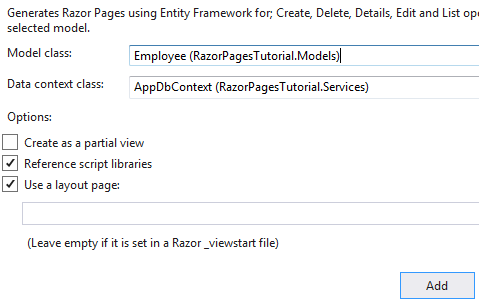
Give it a few seconds and the fully functional CRUD pages are generated.
Validation attributes and scaffolding
The code generated by the scaffolding tools will take the validation attributes (if present) into consideration and include the relevant tag helpers on the respective CRUD pages.
public class Employee
{
public int Id { get; set; }
[Required, MinLength(3, ErrorMessage = "Name must contain at least 3 characters")]
public string Name { get; set; }
[Required]
[Display(Name = "Office Email")]
[RegularExpression(@"^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$",
ErrorMessage = "Invalid email format")]
public string Email { get; set; }
public string PhotoPath { get; set; }
[Required]
public Dept? Department { get; set; }
}
{
public int Id { get; set; }
[Required, MinLength(3, ErrorMessage = "Name must contain at least 3 characters")]
public string Name { get; set; }
[Required]
[Display(Name = "Office Email")]
[RegularExpression(@"^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$",
ErrorMessage = "Invalid email format")]
public string Email { get; set; }
public string PhotoPath { get; set; }
[Required]
public Dept? Department { get; set; }
}
You can repeat this process for other model classes (i.e entities) in your application. This scaffolding feature saves lot of time.
Using the scaffolding feature we can also generate API and MVC controllers.

No comments:
Post a Comment
It would be great if you can help share these free resources