Suggested Videos
Part 2 - ASP.NET core 3.0 project file | Text | Slides
Part 3 - Layout view in razor pages project | Text | Slides
Part 4 - Razor Pages - Creating Reusable Models Project | Text | Slides
In this video we will discuss, creating a reusable data access class library project. This project can then be reused with a razor pages web application, windows application, web api etc. It works cross platform.
Creating .Net Standard Class Library Project
In the Solution Explorer, right click on the solution and select Add > New Project.
In the Add a new project dialog, type Class Library. Select .NET Standard Class Library.
On the next screen provide a name for the project. Since this project is going to contain the data access services, let's name it RazorPagesTutorial.Services and click Create.
Adding project reference
The services project (RazorPagesTutorial.Services) needs the types (Employee and Dept) defined in the Models project (RazorPagesTutorial.Models). So we need to add a reference to the Models project in the Services project. The following are the steps.
Right click on RazorPagesTutorial.Services project, and select Add > Reference
In the Reference Manager window, click on the Projects tab. Select RazorPagesTutorial.Models project from the list and click OK.
In the solution explorer you will see RazorPagesTutorial.Models project listed as a project dependency
In the project file (RazorPagesTutorial.Services.csproj), you will see RazorPagesTutorial.Models included as a project reference.
IEmployeeRepository interface
We will be using the following IEmployeeRepository interface for data access. This interface abstraction allows us to use dependency injection. If you are new to the concept of dependency injection, please check out Part 19 of ASP.NET Core MVC tutorial for beginners course.
MockEmployeeRepository class
At the moment we have employee data hard-coded in the application code. We will discuss how to retrieve employee data from a SQL Server database in our upcoming videos.
Part 2 - ASP.NET core 3.0 project file | Text | Slides
Part 3 - Layout view in razor pages project | Text | Slides
Part 4 - Razor Pages - Creating Reusable Models Project | Text | Slides
In this video we will discuss, creating a reusable data access class library project. This project can then be reused with a razor pages web application, windows application, web api etc. It works cross platform.
Creating .Net Standard Class Library Project
In the Solution Explorer, right click on the solution and select Add > New Project.
In the Add a new project dialog, type Class Library. Select .NET Standard Class Library.
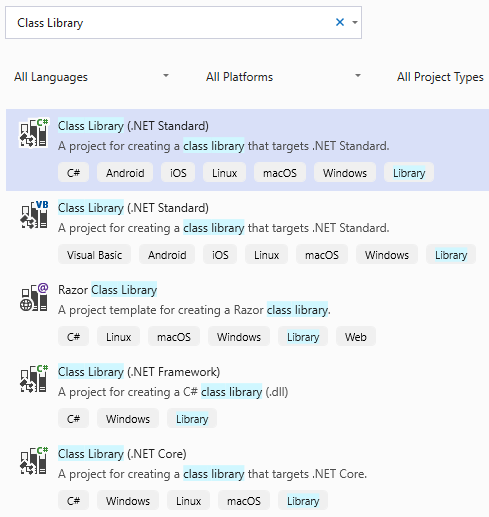
On the next screen provide a name for the project. Since this project is going to contain the data access services, let's name it RazorPagesTutorial.Services and click Create.
Adding project reference
The services project (RazorPagesTutorial.Services) needs the types (Employee and Dept) defined in the Models project (RazorPagesTutorial.Models). So we need to add a reference to the Models project in the Services project. The following are the steps.
Right click on RazorPagesTutorial.Services project, and select Add > Reference
In the Reference Manager window, click on the Projects tab. Select RazorPagesTutorial.Models project from the list and click OK.

In the solution explorer you will see RazorPagesTutorial.Models project listed as a project dependency

In the project file (RazorPagesTutorial.Services.csproj), you will see RazorPagesTutorial.Models included as a project reference.
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
</PropertyGroup>
<ItemGroup>
<ProjectReference
Include="..\RazorPagesTutorial.Models\RazorPagesTutorial.Models.csproj" />
</ItemGroup>
</Project>
IEmployeeRepository interface
We will be using the following IEmployeeRepository interface for data access. This interface abstraction allows us to use dependency injection. If you are new to the concept of dependency injection, please check out Part 19 of ASP.NET Core MVC tutorial for beginners course.
using RazorPagesTutorial.Models;
using System.Collections.Generic;
namespace RazorPagesTutorial.Services
{
public interface IEmployeeRepository
{
IEnumerable<Employee> GetAllEmployees();
}
}
using System.Collections.Generic;
namespace RazorPagesTutorial.Services
{
public interface IEmployeeRepository
{
IEnumerable<Employee> GetAllEmployees();
}
}
MockEmployeeRepository class
At the moment we have employee data hard-coded in the application code. We will discuss how to retrieve employee data from a SQL Server database in our upcoming videos.
using RazorPagesTutorial.Models;
using System.Collections.Generic;
namespace RazorPagesTutorial.Services
{
public class MockEmployeeRepository :
IEmployeeRepository
{
private List<Employee> _employeeList;
public MockEmployeeRepository()
{
_employeeList = new List<Employee>()
{
new Employee() { Id = 1, Name = "Mary", Department = Dept.HR,
Email = "mary@pragimtech.com",
PhotoPath="mary.png" },
new Employee() { Id = 2, Name = "John", Department = Dept.IT,
Email = "john@pragimtech.com",
PhotoPath="john.png" },
new Employee() { Id = 3, Name = "Sara", Department = Dept.IT,
Email = "sara@pragimtech.com",
PhotoPath="sara.png" },
new Employee() { Id = 4, Name = "David", Department = Dept.Payroll,
Email = "david@pragimtech.com" },
};
}
public IEnumerable<Employee> GetAllEmployees()
{
return _employeeList;
}
}
}

No comments:
Post a Comment
It would be great if you can help share these free resources