Suggested Tutorials
C# tutorial for beginners
ASP.NET Core MVC tutorial for beginners
SQL Server tutorial for beginners
In this video and in our upcoming videos in this series, we will discuss everything you need to know to build web applications using ASP.NET core Razor Pages.
At this point, you might be wondering we can build a web application using ASP.NET core MVC as well. So, why do we need a different technology like razor pages again. Well, we will discuss the difference between MVC and Razor pages and the guidelines on when to use one over the other in just a bit. Before that, let's understand the prerequisites to follow along with this course. Install the software's required and setup our machine.
Course Prerequisites
To follow along with this course you need to have a basic working knowledge of
Knowledge of the following ASP.NET Core concepts will be extremely helpful. We discussed these in detail in our ASP.NET Core MVC tutorial for beginners course.
Software required
We need 2 software's - Visual Studio 2019 and .NET Core 3.0
Visual Studio 2019 : Visual Studio 2019 is now released. So we will be using this as the editor. The community edition is free and can be downloaded from the following URL.
https://visualstudio.microsoft.com/vs/
Click on Community 2019 option from the dropdownlist
An executable will be downloaded. Run the executable as an administrator. On the workloads selection screen, select ASP.NET and web development workload and then click the install button.
.NET Core 3.0 : As of this recording, the latest version of .NET Core is 3.0 and this is the version we will use in this course. Download and install .NET Core 3.0 from the following link.
https://dotnet.microsoft.com/download/dotnet-core
Create razor pages web application
We will use Visual Studio 2019 to create a new web application using razor pages. The following are the steps.
Launch Visual Studio 2019
Click on Create a new project option
On the subsequent screen, select ASP.NET Core Web Application template and click Next
On the next screen, provide a name for the project. I named it RazorPagesTutorial. Specify a location where the project should be created and then click Create button.
Understanding Razor Pages
Razor pages are in the Pages folder in the root web application folder
Index.cshtml
The PageModel class (Index.html.cs)
Notice, the public property Message is available in the display template through @Model.Message
When we run this project and navigate to https://localhost:44342/index we see the index page in the browser. Similarly if you have a ContactUs.chtml razor page and when you navigate to https://localhost:44342/ContactUs, you will see the ContactUs page in the browser. The extension .CSHTML is not required in the URL.
ASP.NET Webforms vs Razor Pages
ASP.NET Core Razor Pages framework is a new technology to build page-focused web applications quicker and more efficiently with clean separation of concerns. Razor pages are introduced in .NET Core 2.0. It is lightweight, flexible and provides the developer the full control over the rendered HTML.
In some respects, razor pages are similar to the classic asp.net webforms framework. In ASP.NET Webforms, we have an ASPX page and a code-dehind class. The ASPX page contains the HTML and controls the visual part. The code-behind class contains the server-side c# or visual basic code that handles the page events. For example, if you have a WebForm with name WebForm1. It's actually a pair of files - WebForm1.aspx (the display template) and WebForm1.aspx.cs (the code-behind class)
Similarly, each razor page is also a pair of files - .cshtml and .cshtml.cs
With the MVC design pattern we have the Model, View and Controller. It is the Controller in the MVC design pattern that receives the request and handles it. The controller creates the model. The model has the classes that describe the data. In addition to the data, Model also contains the logic to retrieve data from the underlying data source such as a database. In addition to creating the Model, the controller also selects a View and passes the Model object to the View. The view contains the presentation logic to display the Model data provided to it by the Controller.
In MVC, in addition to Model, View and Controller, we also have Actions and ViewModels. If we are building a fairly complex portal, chances are we may end up with controllers that work with many different dependencies and view models and return many different views. In short we may end up with large controllers with many actions that are not related to each other in any way. This not only results in unnecessary complexity but also violate the fundamental principles of programming like Single Responsibility Principle and Open/Closed Principle.
On the other hand a razor page is just a pair of files - a display template and the corresponding PageModel class. As the name implies the display template contains the HTML. The PageModel class contains the server side code and combines the responsibilities of a Controller and a ViewModel. Everything we put in the PageModel class is related to the Page. So, unlike controllers in MVC, bloating the PageModel class with unrelated methods is almost impossible. Since the PageModel class and the display template are at one place and closely related to each other, building individual pages with razor pages is fairly straightforward while still using all the architectural features of ASP.NET Core MVC like dependency injection, middleware components, configuration system, model binding, validation etc.
So the recommendation from Microsoft is to use razor pages if we are building a Web UI (web pages) and ASP.NET Core MVC if we are building a Web API.
Whether you use ASP.NET Core MVC or Razor Pages for building a web application, there's no difference from performance standpoint.
It's also possible to combine both the patterns (i.e ASP.NET Core MVC and Razor Pages) in a given single asp.net core web application.
What to learn Razor Pages or MVC
I personally think an asp.net core developer must have both the skills - Razor Pages and MVC. So if you are starting to learn ASP.NET Core, I suggest start with ASP.NET Core tutorial for beginners course and then this razor pages tutorial.
C# tutorial for beginners
ASP.NET Core MVC tutorial for beginners
SQL Server tutorial for beginners
In this video and in our upcoming videos in this series, we will discuss everything you need to know to build web applications using ASP.NET core Razor Pages.
At this point, you might be wondering we can build a web application using ASP.NET core MVC as well. So, why do we need a different technology like razor pages again. Well, we will discuss the difference between MVC and Razor pages and the guidelines on when to use one over the other in just a bit. Before that, let's understand the prerequisites to follow along with this course. Install the software's required and setup our machine.
Course Prerequisites
To follow along with this course you need to have a basic working knowledge of
- ASP.NET Core
- C#
- HTML and CSS
Knowledge of the following ASP.NET Core concepts will be extremely helpful. We discussed these in detail in our ASP.NET Core MVC tutorial for beginners course.
- Middleware components
- HTTP request processing pipeline
- configuration system
- tag helpers
- layout files
- layout sections
- routing
- static files
- viewimports
- viewstart
- model binding and validation
- dependency injection
- database migrations
- logging
Software required
We need 2 software's - Visual Studio 2019 and .NET Core 3.0
Visual Studio 2019 : Visual Studio 2019 is now released. So we will be using this as the editor. The community edition is free and can be downloaded from the following URL.
https://visualstudio.microsoft.com/vs/
Click on Community 2019 option from the dropdownlist

An executable will be downloaded. Run the executable as an administrator. On the workloads selection screen, select ASP.NET and web development workload and then click the install button.
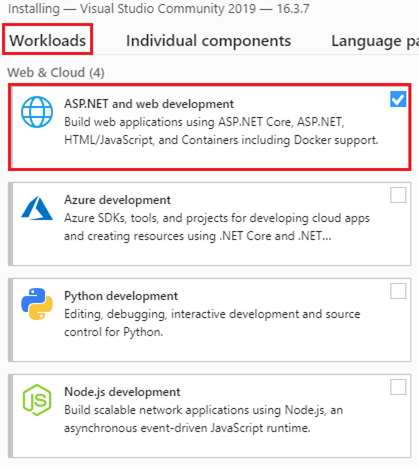
.NET Core 3.0 : As of this recording, the latest version of .NET Core is 3.0 and this is the version we will use in this course. Download and install .NET Core 3.0 from the following link.
https://dotnet.microsoft.com/download/dotnet-core
Create razor pages web application
We will use Visual Studio 2019 to create a new web application using razor pages. The following are the steps.
Launch Visual Studio 2019
Click on Create a new project option

On the subsequent screen, select ASP.NET Core Web Application template and click Next
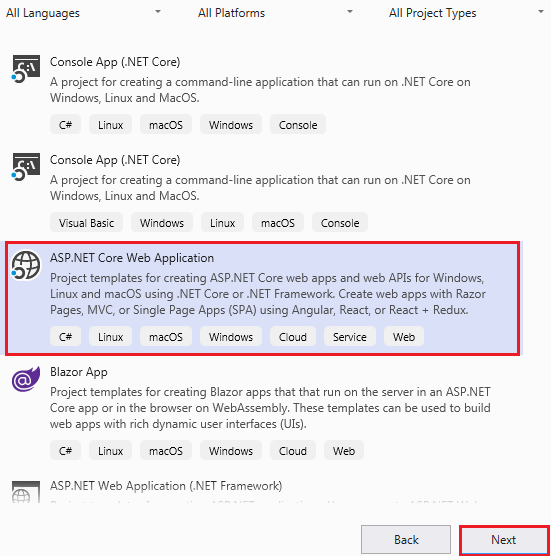
On the next screen, provide a name for the project. I named it RazorPagesTutorial. Specify a location where the project should be created and then click Create button.
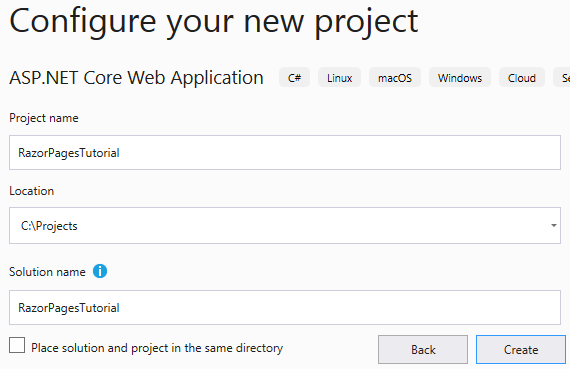
Understanding Razor Pages
Razor pages are in the Pages folder in the root web application folder
Index.cshtml
@page
@model
IndexModel
@{
ViewData["Title"] = "Home page";
}
<div class="text-center">
<h1 class="display-4">Welcome</h1>
</div>
- Index.cshtml is the display template and has the extension .cshtml.
- It is much like a razor view file in MVC.
- @page directive specifies it's a razor page.
- @model directive specifies the model. The model is the corresponding PageModel class which is shown below.
using Microsoft.AspNetCore.Mvc.RazorPages;
using Microsoft.Extensions.Logging;
namespace RazorPagesTutorial.Pages
{
public class IndexModel : PageModel
{
private readonly ILogger<IndexModel> _logger;
public IndexModel(ILogger<IndexModel> logger)
{
_logger = logger;
}
public void OnGet()
{
}
}
}
using Microsoft.Extensions.Logging;
namespace RazorPagesTutorial.Pages
{
public class IndexModel : PageModel
{
private readonly ILogger<IndexModel> _logger;
public IndexModel(ILogger<IndexModel> logger)
{
_logger = logger;
}
public void OnGet()
{
}
}
}
- Index.cshtml.cs is the corresponding PageModel class.
- It has the same name as the display template and ends with .cs extension. CS because the programming language is CSHARP.
- The class in this file is the model for the display template. It derives from the PageModel class.
- Just like MVC, razor pages also support dependency injection.
- The built-in ILogger service is injected using the constructor.
- This ILogger service enables us to log to several different logging destinations.
- In addition to Dependency Injection and Logging, other asp.net core features like configuration sources, model binding, model validation etc are also supported by razor pages.
The PageModel class (Index.html.cs)
public class IndexModel : PageModel
{
public string Message { get; set; }
public void OnGet()
{
Message = "Hello World!";
}
}
{
public string Message { get; set; }
public void OnGet()
{
Message = "Hello World!";
}
}
- Razor pages use public properties to expose data to the display templates.
- The public property Message is available in the display template.
- In addition to these public properties which carry data to the display template, the PageModel class also includes methods like OnGet() and OnPost().
- These are the methods that respond to HTTP GET and POST requests respectively.
@page
@model
IndexModel
@{
ViewData["Title"] = "Home page";
}
<div class="text-center">
<h1>@Model.Message</h1>
</div>
Notice, the public property Message is available in the display template through @Model.Message
When we run this project and navigate to https://localhost:44342/index we see the index page in the browser. Similarly if you have a ContactUs.chtml razor page and when you navigate to https://localhost:44342/ContactUs, you will see the ContactUs page in the browser. The extension .CSHTML is not required in the URL.
ASP.NET Webforms vs Razor Pages
ASP.NET Core Razor Pages framework is a new technology to build page-focused web applications quicker and more efficiently with clean separation of concerns. Razor pages are introduced in .NET Core 2.0. It is lightweight, flexible and provides the developer the full control over the rendered HTML.

In some respects, razor pages are similar to the classic asp.net webforms framework. In ASP.NET Webforms, we have an ASPX page and a code-dehind class. The ASPX page contains the HTML and controls the visual part. The code-behind class contains the server-side c# or visual basic code that handles the page events. For example, if you have a WebForm with name WebForm1. It's actually a pair of files - WebForm1.aspx (the display template) and WebForm1.aspx.cs (the code-behind class)
Similarly, each razor page is also a pair of files - .cshtml and .cshtml.cs
- .cshtml - Is the display template. So it contains the HTML and razor syntax.
- .cshtml.cs - Contains the server side C# code that handles the page events and provides the data the template needs.
With the MVC design pattern we have the Model, View and Controller. It is the Controller in the MVC design pattern that receives the request and handles it. The controller creates the model. The model has the classes that describe the data. In addition to the data, Model also contains the logic to retrieve data from the underlying data source such as a database. In addition to creating the Model, the controller also selects a View and passes the Model object to the View. The view contains the presentation logic to display the Model data provided to it by the Controller.

In MVC, in addition to Model, View and Controller, we also have Actions and ViewModels. If we are building a fairly complex portal, chances are we may end up with controllers that work with many different dependencies and view models and return many different views. In short we may end up with large controllers with many actions that are not related to each other in any way. This not only results in unnecessary complexity but also violate the fundamental principles of programming like Single Responsibility Principle and Open/Closed Principle.
On the other hand a razor page is just a pair of files - a display template and the corresponding PageModel class. As the name implies the display template contains the HTML. The PageModel class contains the server side code and combines the responsibilities of a Controller and a ViewModel. Everything we put in the PageModel class is related to the Page. So, unlike controllers in MVC, bloating the PageModel class with unrelated methods is almost impossible. Since the PageModel class and the display template are at one place and closely related to each other, building individual pages with razor pages is fairly straightforward while still using all the architectural features of ASP.NET Core MVC like dependency injection, middleware components, configuration system, model binding, validation etc.
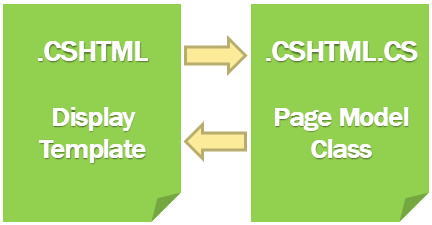
So the recommendation from Microsoft is to use razor pages if we are building a Web UI (web pages) and ASP.NET Core MVC if we are building a Web API.
Whether you use ASP.NET Core MVC or Razor Pages for building a web application, there's no difference from performance standpoint.
It's also possible to combine both the patterns (i.e ASP.NET Core MVC and Razor Pages) in a given single asp.net core web application.
What to learn Razor Pages or MVC
I personally think an asp.net core developer must have both the skills - Razor Pages and MVC. So if you are starting to learn ASP.NET Core, I suggest start with ASP.NET Core tutorial for beginners course and then this razor pages tutorial.

No comments:
Post a Comment
It would be great if you can help share these free resources