Suggested Videos
Part 84 - List all users from asp.net core identity database | Text | Slides
Part 85 Edit identity user in asp.net core | Text | Slides
Part 86 - Delete identity user in asp.net core | Text | Slides
In this video we will discuss how to display DELETE confirmation in ASP.NET Core.
We will discuss the following two types of confirmation when the DELETE button is clicked.
Delete confirmation dialog in asp.net core
When the DELETE button is clicked we want to display the following browser confirm dialog.
Yes or No Delete buttons instead of confirmation dialog
These days most people do not like confirmation dialog. Instead they want something inline, like the Yes and No buttons below.
confirmDelete JavaScript function
How to get confirmDelete JavaScript function into the view
Part 84 - List all users from asp.net core identity database | Text | Slides
Part 85 Edit identity user in asp.net core | Text | Slides
Part 86 - Delete identity user in asp.net core | Text | Slides
In this video we will discuss how to display DELETE confirmation in ASP.NET Core.
We will discuss the following two types of confirmation when the DELETE button is clicked.
Delete confirmation dialog in asp.net core
When the DELETE button is clicked we want to display the following browser confirm dialog.

- This is very easy to achieve. All we need is the onclick attribute on the DELETE button.
- The JavaScript confirm() function displays the confirmation dialog to the user.
- If the Cancel button is clicked on the confirmation dialog, the confirm() function returns false and nothing happens.
- If the OK button is clicked, the form is submitted and the respective user record is deleted from the database
<button type="submit" class="btn btn-danger"
onclick="return confirm('Are you sure you want to delete user : @user.UserName')">
Delete
</button>
Yes or No Delete buttons instead of confirmation dialog
These days most people do not like confirmation dialog. Instead they want something inline, like the Yes and No buttons below.
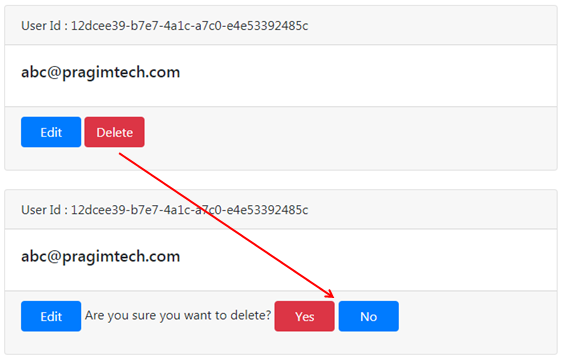
- The span elements that surround the Delete, Yes and No buttons will be dynamically generated for every user on the list page.
- If you have more than one user on the page there will be more than one span element
- To ensure these span elements have unique ID's we are appending User.Id which is a Guid and guaranteed to be unique
<div class="card-footer">
<form method="post" asp-action="DeleteUser" asp-route-id="@user.Id">
<a asp-controller="Administration" asp-action="Edituser"
asp-route-id="@user.Id" class="btn btn-primary">Edit</a>
<span id="confirmDeleteSpan_@user.Id" style="display:none">
<span>Are you
sure you want to delete?</span>
<button type="submit" class="btn btn-danger">Yes</button>
<a href="#" class="btn btn-primary"
onclick="confirmDelete('@user.Id', false)">No</a>
</span>
<span id="deleteSpan_@user.Id">
<a href="#" class="btn btn-danger"
onclick="confirmDelete('@user.Id', true)">Delete</a>
</span>
</form>
</div>
confirmDelete JavaScript function
function confirmDelete(uniqueId, isDeleteClicked) {
var deleteSpan = 'deleteSpan_' + uniqueId;
var confirmDeleteSpan = 'confirmDeleteSpan_' + uniqueId;
if (isDeleteClicked) {
$('#' + deleteSpan).hide();
$('#' + confirmDeleteSpan).show();
} else {
$('#' + deleteSpan).show();
$('#' + confirmDeleteSpan).hide();
}
}
How to get confirmDelete JavaScript function into the view
- We need confirmDelete() JavaScript function on ListUsers view
- In the layout file (_Layout.cshtml) we have a defined a "Scripts" section. This section is optional.
- We discussed sections in detail in Part 29 of ASP.NET Core tutorial.
- We need this confirmDelete() function only in this ListUsers view
- So, we are using the optional Scripts section to include CustomScript.js file which contains confirmDelete() function.
@section Scripts {
<script src="~/js/CustomScript.js"></script>
}

No comments:
Post a Comment
It would be great if you can help share these free resources