Suggested Videos
Part 80 - Edit role in asp.net core | Text | Slides
Part 81 - Add or remove users from role in asp.net core | Text | Slides
Part 82 - ASP.NET Core role based authorization | Text | Slides
In this video we will discuss how to show or hide navigation menu items based on the logged-in user role in asp.net core mvc.
If the logged-in user is in Admin role, then we want to display Manage Roles navigation menu item.
If the logged-in user IS NOT in Admin role, then Manage Roles navigation menu item should not be displayed.
Show or hide navigation menu based on logged-in user role
What if the user types the URL in address bar
The URL associated with Manage Roles navigation menu item is /Administration/ListRoles. What if the user types this URL directly in the address bar.
The Authorize attribute on the AdministrationController protects from the unauthorised access. If the logged-in user is not in Admin role, asp.net core automatically redirects the user to /Account/AccessDenied.
AccessDenied action in AccountController
AccessDenied View
This is how AccessDenied view looks when rendered
Part 80 - Edit role in asp.net core | Text | Slides
Part 81 - Add or remove users from role in asp.net core | Text | Slides
Part 82 - ASP.NET Core role based authorization | Text | Slides
In this video we will discuss how to show or hide navigation menu items based on the logged-in user role in asp.net core mvc.
If the logged-in user is in Admin role, then we want to display Manage Roles navigation menu item.

If the logged-in user IS NOT in Admin role, then Manage Roles navigation menu item should not be displayed.

Show or hide navigation menu based on logged-in user role
- Navigation menu is in the laylout view (_Layout.cshtml).
- Inject SignInManager service into the layout view using @inject directive
- Use the SignInManager service, IsSignedIn() method and IsInRole() method to check if the user is signed in and if the user is in the Admin role
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link" asp-controller="home" asp-action="index">List</a>
</li>
<li class="nav-item">
<a class="nav-link" asp-controller="home" asp-action="create">Create</a>
</li>
@if
(SignInManager.IsSignedIn(User) && User.IsInRole("Admin"))
{
<li class="nav-item">
<a class="nav-link" asp-controller="Administration" asp-action="ListRoles">
Manage Roles
</a>
</li>
}
</ul>
What if the user types the URL in address bar
The URL associated with Manage Roles navigation menu item is /Administration/ListRoles. What if the user types this URL directly in the address bar.
The Authorize attribute on the AdministrationController protects from the unauthorised access. If the logged-in user is not in Admin role, asp.net core automatically redirects the user to /Account/AccessDenied.
[Authorize(Roles = "Admin")]
public class AdministrationController : Controller
{
// Code
}
public class AdministrationController : Controller
{
// Code
}
AccessDenied action in AccountController
public class AccountController : Controller
{
[HttpGet]
[AllowAnonymous]
public IActionResult AccessDenied()
{
return View();
}
// Other actions
}
{
[HttpGet]
[AllowAnonymous]
public IActionResult AccessDenied()
{
return View();
}
// Other actions
}
AccessDenied View
<div class="text-center">
<h1 class="text-danger">Access Denied</h1>
<h6 class="text-danger">You do not have
persmission to view this resource</h6>
<img src="~/images/noaccess.png" style="height:300px; width:300px" />
</div>
This is how AccessDenied view looks when rendered
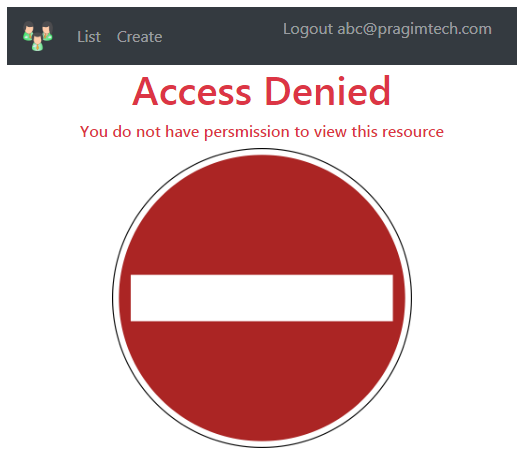

how Authorize attribute knows to redirect to accountcontroller
ReplyDeleteAccessDenied action method?
I have not mention that routing path in my application