Suggested Videos:
Part 12 - Difference between int and Int32 in c#
Part 13 - Reverse each word in a string using c#
Part 14 - C# abstract class virtual method
One of our YouTube channel subscribers faced this c# question in an interview for an entry level c# developer role. So here is the question.
What is the Access Modifier of the default parameter-less constructor in C#
The answer is Public
Let's prove this. Consider the following Customer class
The Customer class does not have an explicit constructor defined. In C#, if we do not provide an explicit constructor, a default parameter-less constructor is automatically provided. The access modifier for that constructor is Public.
We can prove this using the following reflection code.
Output:
Is Constructor : True
Is Public : True
The following code checks if the constructor is private or protected
Part 12 - Difference between int and Int32 in c#
Part 13 - Reverse each word in a string using c#
Part 14 - C# abstract class virtual method
One of our YouTube channel subscribers faced this c# question in an interview for an entry level c# developer role. So here is the question.
What is the Access Modifier of the default parameter-less constructor in C#
The answer is Public
Let's prove this. Consider the following Customer class
class Customer
{
}
{
}
The Customer class does not have an explicit constructor defined. In C#, if we do not provide an explicit constructor, a default parameter-less constructor is automatically provided. The access modifier for that constructor is Public.
We can prove this using the following reflection code.
using System;
using System.Reflection;
namespace Demo
{
class Program
{
static void Main(string[] args)
{
Type type = typeof(Customer);
// Type.EmptyTypes represents an Empty Type[] array
// We use an Empty Type[] array to get the Constructor that
// takes no parameters
ConstructorInfo constructorInfo = type.GetConstructor(Type.EmptyTypes);
Console.WriteLine("Is Constructor : " + constructorInfo.IsConstructor);
Console.WriteLine("Is Public : " + constructorInfo.IsPublic);
}
}
class Customer
{
}
}
using System.Reflection;
namespace Demo
{
class Program
{
static void Main(string[] args)
{
Type type = typeof(Customer);
// Type.EmptyTypes represents an Empty Type[] array
// We use an Empty Type[] array to get the Constructor that
// takes no parameters
ConstructorInfo constructorInfo = type.GetConstructor(Type.EmptyTypes);
Console.WriteLine("Is Constructor : " + constructorInfo.IsConstructor);
Console.WriteLine("Is Public : " + constructorInfo.IsPublic);
}
}
class Customer
{
}
}
Output:
Is Constructor : True
Is Public : True
The following code checks if the constructor is private or protected
using System;
using System.Reflection;
namespace Demo
{
class Program
{
static void Main(string[] args)
{
Type type = typeof(Customer);
ConstructorInfo constructorInfo = type.GetConstructor(
BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic,
null, Type.EmptyTypes, null);
Console.WriteLine("Is Constructor : " + constructorInfo.IsConstructor);
Console.WriteLine("Is Protected : " + constructorInfo.IsFamily);
}
}
class Customer
{
protected Customer()
{ }
}
}
using System.Reflection;
namespace Demo
{
class Program
{
static void Main(string[] args)
{
Type type = typeof(Customer);
ConstructorInfo constructorInfo = type.GetConstructor(
BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic,
null, Type.EmptyTypes, null);
Console.WriteLine("Is Constructor : " + constructorInfo.IsConstructor);
Console.WriteLine("Is Protected : " + constructorInfo.IsFamily);
}
}
class Customer
{
protected Customer()
{ }
}
}
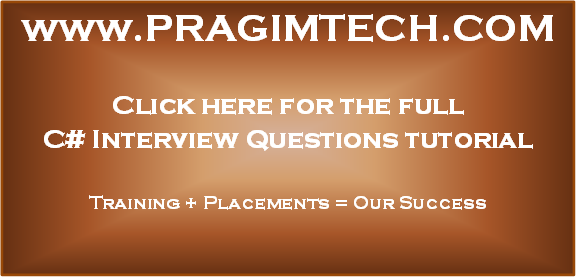
No comments:
Post a Comment
It would be great if you can help share these free resources