Suggested Videos
Part 28 - Angular populate formarray | Text | Slides
Part 29 - Angular reactive forms put example | Text | Slides
Part 30 - Angular reactive forms post example | Text | Slides
In this video we will discuss, what are Angular modules and why we need them in an Angular project.
In simple terms an Angular Module is a class decorated with @NgModule decorator.
An Angular Module is a mechanism to group components, directives, pipes and services that are related to a feature area of an angular application.
For example, if you are building an application to manage employees, you might have the following features in your application.
To group the components, directives, pipes and services related to a specific feature area, we create a module for each feature area. These modules are called feature modules.
In addition to feature modules, an Angular application also contains the following modules.
What are the advantages of splitting an angular application into multiple Angular Modules
Well, there are several benefits of Angular Modules.
@NgModule Decorator
As we have already discussed an Angular module is a class that is decorated with @NgModule decorator. The @NgModule decorator has the following properties.
In preparation for refactoring our application into multiple modules, let's create the following 2 components
Use the following Angular CLI command to create the PageNotFoundComponent
If you are new to Angular CLI, please check out our Angular CLI course at the following link.
https://www.youtube.com/playlist?list=PL6n9fhu94yhWUcq5Pc16uf8YKXoZ87Vh_
Copy and paste the following code in home.component.html
Please note : Create images folder in the assets folder. Download the following image. Name it Employees.jpg and place it in the images folder.
Copy and paste the following code in page-not-found.component.html
Include home and wild card routes in app-routing.module.ts
In the root component (app.component.html) include a menu item for the home route
In our next video we will discuss refactoring our application to include employee feature module.
Part 28 - Angular populate formarray | Text | Slides
Part 29 - Angular reactive forms put example | Text | Slides
Part 30 - Angular reactive forms post example | Text | Slides
In this video we will discuss, what are Angular modules and why we need them in an Angular project.
In simple terms an Angular Module is a class decorated with @NgModule decorator.
An Angular Module is a mechanism to group components, directives, pipes and services that are related to a feature area of an angular application.
For example, if you are building an application to manage employees, you might have the following features in your application.
Application Feature | Description |
---|---|
Employee Feature | Deals with creating, reading, updating and deleting employees |
Login Feature | Deals with login, logout, authenticate and authorize users |
Report Feature | Deals with generating employee reports like total number of employees by department, top 10 best employees etc |
To group the components, directives, pipes and services related to a specific feature area, we create a module for each feature area. These modules are called feature modules.
In addition to feature modules, an Angular application also contains the following modules.
Module Type | Description |
---|---|
Root Module | Every Angular application has at least one module, the root module. By default, this root application module is called AppModule. We bootstrap this root module to launch the application. If the application that you are building is a simple application with a few components, then all you need is the root module. As the application starts to grow and become complex, in addition to the root module, we may add several feature modules. We then import these feature modules into the root module. We will discuss creating feature modules in our upcoming videos |
Core Module | The most important use of this module is to include the providers of http services. Services in Angular are usually singletons. So to ensure that, only one instance of a given service is created across the entire application, we include all our singleton service providers in the core module. In most cases, a CoreModule is a pure services module with no declarations. The core module is then imported into the root module (AppModule) only. CoreModule should never be imported in any other module. We will discuss creating a core module in our upcoming videos |
Shared Module | This module contains reusable components, directives, and pipes that we want to use across our application. The Shared module is then imported into specific Feature Modules as needed. The Shared module might also export the commonly used Angular modules like CommonModule, FormsModule etc. so they can be easily used across your application, without importing them in every Feature Module. We will discuss creating a shared module in our upcoming videos |
Routing Modules | An angular application may also have one or more routing modules for application level routes and feature module routes |
What are the advantages of splitting an angular application into multiple Angular Modules
Well, there are several benefits of Angular Modules.
Benefit | Description |
---|---|
Organizing Angular Application |
First of all, Modules are a great way to organise an angular application. Every feature area is present in it's own feature module. All Shared pieces (like components, directives & pipes) are present in a Shared module. All Singleton services are present in a core module. As we clearly know what is present in each module, it's easier to understand, find and change code if required |
Code Reuse | Modules are great way to reuse code. For example, if you have components, directives or pipes that you want to reuse, you include them in a Shared module and import it into the module where you need them rather than duplicating code. Code duplication is just plain wrong, and results in unmaintainable and error prone code. We will discuss creating a Shared module and how it can help us reuse code in our upcoming videos |
Code Maintenance | Since Angular Modules promote code reuse and separation of concerns, they are essential for writing maintainable code in angular projects |
Performance | Another great reason to refactor your application into modules is performance. Angular modules can be loaded either eagerly when the application starts or lazily on demand when they are actually needed or in the background. Lazy loading angular modules can significantly boost the application start up time. We will discuss lazy loading modules in our upcoming videos |
@NgModule Decorator
As we have already discussed an Angular module is a class that is decorated with @NgModule decorator. The @NgModule decorator has the following properties.
- declarations
- bootstrap
- providers
- imports
- exports
In preparation for refactoring our application into multiple modules, let's create the following 2 components
- HomeComponent
- PageNotFoundComponent
ng g c home --flat
Use the following Angular CLI command to create the PageNotFoundComponent
ng g c page-not-found --flat
If you are new to Angular CLI, please check out our Angular CLI course at the following link.
https://www.youtube.com/playlist?list=PL6n9fhu94yhWUcq5Pc16uf8YKXoZ87Vh_
Copy and paste the following code in home.component.html
<div
class="panel
panel-primary">
<div class="panel-heading">
<h3 class="panel-title">Employee
Management System</h3>
</div>
<div class="panel-body">
<img src="../assets/images/Employees.jpg"
class="img-responsive"/>
</div>
</div>
Please note : Create images folder in the assets folder. Download the following image. Name it Employees.jpg and place it in the images folder.

Copy and paste the following code in page-not-found.component.html
<h1>
The page you are looking for cannot be found.
</h1>
Include home and wild card routes in app-routing.module.ts
import
{ HomeComponent } from './home.component';
import
{ PageNotFoundComponent } from './page-not-found.component';
const appRoutes: Routes =
[
// home route
{ path: 'home',
component: HomeComponent },
{ path: 'list',
component: ListEmployeesComponent },
{ path: 'create',
component: CreateEmployeeComponent },
{ path: 'edit/:id',
component: CreateEmployeeComponent },
// redirect to the home route if the client side route
path is empty
{ path: '', redirectTo: '/home',
pathMatch: 'full'
},
// wild card route
{ path: '**',
component: PageNotFoundComponent }
];
In the root component (app.component.html) include a menu item for the home route
<div
class="container">
<nav class="navbar
navbar-default">
<ul class="nav
navbar-nav">
<!--
Include this Home menu item for the home route-->
<li>
<a
routerLinkActive="active"
routerLink="home">Home</a>
</li>
<li>
<a
routerLinkActive="active"
routerLink="list">List</a>
</li>
<li>
<a
routerLinkActive="active"
routerLink="create">Create</a>
</li>
</ul>
</nav>
<router-outlet></router-outlet>
</div>
In our next video we will discuss refactoring our application to include employee feature module.
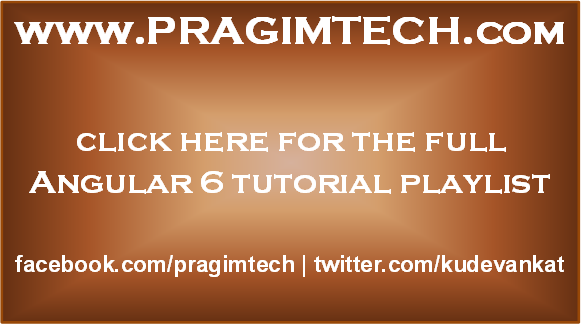
No comments:
Post a Comment
It would be great if you can help share these free resources