Suggested Videos
Part 48 - Angular pure pipe | Text | Slides
Part 49 - Angular impure pipe | Text | Slides
Part 50 - Data filter in angular component | Text | Slides
In this video we will discuss Query String Parameters in Angular. Creating and reading Query String Parameters is somewhat similar to creating and reading required and optional route parameters. We discussed required and optional route parameters in Parts 43 and 44 of Angular CRUD tutorial.
Query parameters are usually used when you want the parameters on the route to be optional and when you want to retain those parameters across multiple routes. For example, on the LIST route, you search for a specific employee. You then click on one of the employees in the search results to view that specific employee details on the DETAILS route. At this point, when we navigate back to the LIST route we want to retain the search term used, so we can display the filtered list instead of the full employee list.
Just like optional route parameters, query parameters are not part of the route configuration and therefore they are not used in route pattern matching.
Passing query string parameters in code : We use the second argument of the Router service navigate() method to pass query string parameters.
The query string parameters start with a question mark and are separated by & as you can see below.
http://localhost:4200/employees/3?searchTerm=John&testParam=testValue
Passing query string parameters in the HTML
Preserve or Merge Query String Parameters : By default, the query string parameters are not preserved or merged when navigating to a different route. To preserve or merge Query Params set queryParamsHandling to either preserve or merge respectively.
Preserve query string parameters in code
Please note : queryParamsHandling is available in Angular 4 and later versions. If you are using Angular 2, you would not have queryParamsHandling. You will have to use preserveQueryParams and set it to true. preserveQueryParams is deprecated since Angular 4.
Merge query string parameters in code :
Preserve query string parameters in the HTML :
Merge query string parameters in the HTML :
Next Video : How to read query params.
Part 48 - Angular pure pipe | Text | Slides
Part 49 - Angular impure pipe | Text | Slides
Part 50 - Data filter in angular component | Text | Slides
In this video we will discuss Query String Parameters in Angular. Creating and reading Query String Parameters is somewhat similar to creating and reading required and optional route parameters. We discussed required and optional route parameters in Parts 43 and 44 of Angular CRUD tutorial.
Query parameters are usually used when you want the parameters on the route to be optional and when you want to retain those parameters across multiple routes. For example, on the LIST route, you search for a specific employee. You then click on one of the employees in the search results to view that specific employee details on the DETAILS route. At this point, when we navigate back to the LIST route we want to retain the search term used, so we can display the filtered list instead of the full employee list.
Just like optional route parameters, query parameters are not part of the route configuration and therefore they are not used in route pattern matching.
Passing query string parameters in code : We use the second argument of the Router service navigate() method to pass query string parameters.
- Create an object with queryParams as the key.
- The value is an object with key/value pairs.
- The key is the name of the query parameter and the value is the value for the query parameter.
this._router.navigate(['employees', employeeId], {
queryParams: { 'searchTerm': this.searchTerm, 'testParam': 'testValue' }
});
The query string parameters start with a question mark and are separated by & as you can see below.
http://localhost:4200/employees/3?searchTerm=John&testParam=testValue
Passing query string parameters in the HTML
<a [routerLink]="['/employees']"
[queryParams]="{ 'searchTerm': 'john', 'testParam':
'testValue'}">
List
</a>
Preserve or Merge Query String Parameters : By default, the query string parameters are not preserved or merged when navigating to a different route. To preserve or merge Query Params set queryParamsHandling to either preserve or merge respectively.
Preserve query string parameters in code
this._router.navigate(['/employees', this._id], {
queryParamsHandling: 'preserve'
});
Please note : queryParamsHandling is available in Angular 4 and later versions. If you are using Angular 2, you would not have queryParamsHandling. You will have to use preserveQueryParams and set it to true. preserveQueryParams is deprecated since Angular 4.
Merge query string parameters in code :
this._router.navigate(['/employees', this._id], {
queryParams: { 'newParam': 'newValue' },
queryParamsHandling: 'merge'
});
Preserve query string parameters in the HTML :
<a [routerLink]="['/list']"
queryParamsHandling="preserve">
Back to List
</a>
Merge query string parameters in the HTML :
<a [routerLink]="['/list']"
[queryParams]="{'newParam': 'newValue'}" queryParamsHandling="merge">
Back to List
</a>
Next Video : How to read query params.
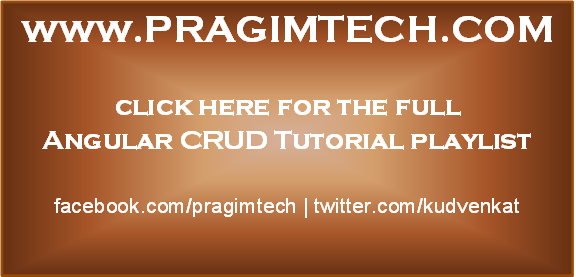
No comments:
Post a Comment
It would be great if you can help share these free resources