Suggested Videos
Part 35 - Angular Injector | Text | Slides
Part 36 - Angular root injector | Text | Slides
Part 37 - Angular router navigate method | Text | Slides
In this video we will discuss using Promises instead of Observables in Angular.
In Angular we can use either Promises or Observables. By default the Angular Http service returns an Observable. To prove this, hover the mouse over the get() method of the Http service in employee.service.ts file. Notice from the intellisense, that it returns Observable<Response>
We discussed Observables in Part 27 of Angular 2 tutorial. To use Promises instead of Observables we will have to first make a change to the service to return a Promise instead of an Observable.
In employee.service.ts file modify getEmployeeByCode() method as shown below. The changes are commented so they are self-explanatory
Modify the code in employee.component.ts file as shown below. The code that we have changed is commented and is self-explanatory.
With the above changes, we are now using a Promise instead of an Observable and the application works the same way as before.
There are several differences between Observables and Promises. We will discuss these differences in our next video.
Part 35 - Angular Injector | Text | Slides
Part 36 - Angular root injector | Text | Slides
Part 37 - Angular router navigate method | Text | Slides
In this video we will discuss using Promises instead of Observables in Angular.
In Angular we can use either Promises or Observables. By default the Angular Http service returns an Observable. To prove this, hover the mouse over the get() method of the Http service in employee.service.ts file. Notice from the intellisense, that it returns Observable<Response>

We discussed Observables in Part 27 of Angular 2 tutorial. To use Promises instead of Observables we will have to first make a change to the service to return a Promise instead of an Observable.
In employee.service.ts file modify getEmployeeByCode() method as shown below. The changes are commented so they are self-explanatory
import { Injectable } from '@angular/core';
import { IEmployee } from './employee';
import { Http, Response } from '@angular/http';
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/operator/map';
import 'rxjs/add/operator/catch';
import 'rxjs/add/Observable/throw';
// import
toPromise operator
import 'rxjs/add/operator/toPromise';
@Injectable()
export class
EmployeeService {
constructor(private _http:
Http) { }
getEmployees():
Observable<IEmployee[]> {
return this._http.get('http://localhost:24535/api/employees')
.map((response: Response) =>
<IEmployee[]>response.json())
.catch(this.handleError);
}
// Notice we
changed the return type of the method to Promise<IEmployee>
// from
Observable<IEmployee>. We are using toPromise() operator to
// return a
Promise. When an exception is thrown handlePromiseError()
// logs the
error to the console and throws the exception again
getEmployeeByCode(empCode: string): Promise<IEmployee> {
return this._http.get("http://localhost:24535/api/employees/" + empCode)
.map((response: Response) =>
<IEmployee>response.json())
.toPromise()
.catch(this.handlePromiseError);
}
// This method
is introduced to handle exceptions
handlePromiseError(error: Response) {
console.error(error);
throw (error);
}
handleError(error: Response) {
console.error(error);
return Observable.throw(error);
}
}
Modify the code in employee.component.ts file as shown below. The code that we have changed is commented and is self-explanatory.
import { Component, OnInit } from '@angular/core';
import { IEmployee } from './employee';
import { EmployeeService } from './employee.service';
import { ActivatedRoute } from '@angular/router';
import { Router } from '@angular/router';
@Component({
selector: 'my-employee',
templateUrl: 'app/employee/employee.component.html',
styleUrls: ['app/employee/employee.component.css']
})
export class
EmployeeComponent implements OnInit {
employee: IEmployee;
statusMessage: string = 'Loading
data. Please wait...';
constructor(private
_employeeService: EmployeeService,
private _activatedRoute: ActivatedRoute,
private _router: Router) { }
ngOnInit() {
let empCode: string = this._activatedRoute.snapshot.params['code'];
// The only
change that we need to make here is use
// then()
method instead of subscribe() method
this._employeeService.getEmployeeByCode(empCode)
.then((employeeData) => {
if (employeeData == null) {
this.statusMessage =
'Employee with the specified Employee Code does not exist';
}
else {
this.employee = employeeData;
}
},
(error) => {
this.statusMessage =
'Problem with the service. Please try again after sometime';
console.error(error);
});
}
onBackButtonClick(): void {
this._router.navigate(['/employees']);
}
}
With the above changes, we are now using a Promise instead of an Observable and the application works the same way as before.
There are several differences between Observables and Promises. We will discuss these differences in our next video.
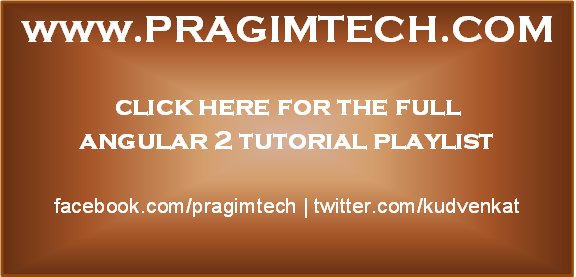
Hi venkat sir Happy Teachers Day,....sorry for the late wishes from my side,....
ReplyDeleteI am getting the following error even after converting the observable to Promise.Please Help
ReplyDeleteType 'Promise' is not assignable to type 'Promise'.
Hi
DeleteMake changes in the getEmployeeCode() it's working fine now
getEmployeeByCode(empCode: string): Promise {
return this._http.get("http://localhost:10665/api/employees/" + empCode)
.map(response => response.json())
.toPromise()
.catch(this.handlePromiseError);
}
Try this:
DeletehandlePromiseError(error:Response):Promise {
console.error(error);
throw(error);
}
I had to:
DeletehandlePromiseError(error:Response):Promise<IEmployee> {
console.error(error);
throw(error);
}
try this may fix the error
DeletehandlePromiseError(error: Response):Promise {
console.error(error);
throw(error);
This, or the Anonymous's response works :
ReplyDeletehandlePromiseError(error: Response): Promise {
console.error(error);
throw (error);
}
I am still getting emp101 for all links in the program whether I use Observable or Promise. Makes no difference. Can you tell me why this is happening?
ReplyDeleteI am still getting same the following error even after converting the observable to Promise.Please Help
ReplyDeleteType 'Promise' is not assignable to type 'Promise'.
I had to:
DeletehandlePromiseError(error:Response):Promise {
console.error(error);
throw(error);
}
I have an error message:
ReplyDelete'Promise' is not assignable to type 'Promise'.
Type 'void | IEmployee' is not assignable to type 'IEmployee'.
Type 'void' is not assignable to type 'IEmployee'.
just make the return type of getEmployeesByCode as Promise and change the code as below:
ReplyDeletegetEmployeesByCode(empCode: string): Promise {
return this._http.get("<>" + empCode)
.map((response: Response) => response.json())
.toPromise()
.catch(this.handlePromiseError);
}
handlePromiseError(error: Response) {
console.error(error);
throw(error);
}