Suggested Videos
Part 4 - Thread Safety in Singleton - Text - Slides
Part 5 - Lazy vs Eager loading in Singleton - Text - Slides
Part 6 - Static Class vs Singleton - Text - Slides
In this tutorial we will discuss how to create a simple employee web application using ASP.NET MVC and we will create a custom logger library using Singleton design pattern which logs exceptions to an external file
Logger Library
Part 4 - Thread Safety in Singleton - Text - Slides
Part 5 - Lazy vs Eager loading in Singleton - Text - Slides
Part 6 - Static Class vs Singleton - Text - Slides
In this tutorial we will discuss how to create a simple employee web application using ASP.NET MVC and we will create a custom logger library using Singleton design pattern which logs exceptions to an external file
Logger Library
ILog.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Logger
{
public interface ILog
{
void LogException(string message);
}
}
Log.cs
using System;
using
System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Logger
{
public sealed class Log : ILog
{
private Log()
{
}
private static readonly Lazy<Log> instance =
new Lazy<Log>(() => new Log());
public static Log GetInstance
{
get
{
return
instance.Value;
}
}
public void LogException(string message)
{
string fileName = string.Format("{0}_{1}.log", "Exception", DateTime.Now.ToShortDateString());
string logFilePath = string.Format(@"{0}\{1}", AppDomain.CurrentDomain.BaseDirectory, fileName);
StringBuilder sb = new StringBuilder();
sb.AppendLine("----------------------------------------");
sb.AppendLine(DateTime.Now.ToString());
sb.AppendLine(message);
using (StreamWriter writer =
new StreamWriter(logFilePath,
true))
{
writer.Write(sb.ToString());
writer.Flush();
}
}
}
}
Create and MVC
Application and Create EmployeePortal DB with Employee Table
Employee Table
CREATE TABLE [dbo].[Employee] (
[Id] INT IDENTITY (1, 1) NOT NULL,
[Name] VARCHAR (50) NOT NULL,
[JobDescription] VARCHAR (50) NOT NULL,
[Number] VARCHAR (50) NOT NULL,
[Department] VARCHAR (50) NOT NULL,
PRIMARY KEY CLUSTERED ([Id] ASC)
);
Generate Model using
ADO.Net entity model generator using the above Table. Post generation, Add an
Employee controller and use generated model which further creates views for
Employee which facilitates CRUD operations on the employee.
Sample EmployeeController.cs
using Logger;
using System;
using
System.Collections.Generic;
using System.Data;
using System.Data.Entity;
using System.Linq;
using System.Net;
using System.Web;
using System.Web.Mvc;
using Web.Models;
namespace Web.Controllers
{
public class EmployeesController : Controller
{
private ILog _ILog;
private EmployeePortalEntities db = new EmployeePortalEntities();
public
EmployeesController()
{
_ILog = Log.GetInstance;
}
protected override void OnException(ExceptionContext filterContext)
{
_ILog.LogException(filterContext.Exception.ToString());
filterContext.ExceptionHandled = true;
this.View("Error").ExecuteResult(this.ControllerContext);
}
// GET: Employees
public ActionResult Index()
{
return
View(db.Employees.ToList());
}
// GET: Employees/Details/5
public ActionResult Details(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Employee employee =
db.Employees.Find(id);
if (employee == null)
{
return
HttpNotFound();
}
return
View(employee);
}
// GET: Employees/Create
public ActionResult Create()
{
return View();
}
// POST: Employees/Create
// To protect from overposting attacks, please enable the
specific properties you want to bind to, for
// more details see
http://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create([Bind(Include = "Id,Name,JobDescription,Number,Department")] Employee employee)
{
if
(ModelState.IsValid)
{
db.Employees.Add(employee);
db.SaveChanges();
return
RedirectToAction("Index");
}
return
View(employee);
}
// GET: Employees/Edit/5
public ActionResult Edit(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Employee employee =
db.Employees.Find(id);
if (employee == null)
{
return
HttpNotFound();
}
return
View(employee);
}
// POST: Employees/Edit/5
// To
protect from overposting attacks, please enable the specific properties you
want to bind to, for
// more details see
http://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Edit([Bind(Include = "Id,Name,JobDescription,Number,Department")] Employee employee)
{
if
(ModelState.IsValid)
{
db.Entry(employee).State = EntityState.Modified;
db.SaveChanges();
return RedirectToAction("Index");
}
return
View(employee);
}
// GET: Employees/Delete/5
public ActionResult Delete(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Employee employee =
db.Employees.Find(id);
if (employee == null)
{
return
HttpNotFound();
}
return View(employee);
}
// POST: Employees/Delete/5
[HttpPost, ActionName("Delete")]
[ValidateAntiForgeryToken]
public ActionResult
DeleteConfirmed(int id)
{
Employee employee =
db.Employees.Find(id);
db.Employees.Remove(employee);
db.SaveChanges();
return
RedirectToAction("Index");
}
protected override void Dispose(bool disposing)
{
if (disposing)
{
db.Dispose();
}
base.Dispose(disposing);
}
}
}
- Run the application and all the exceptions will be logged under the file created by the logger class library.
- This Proves that singleton design pattern comes handy in the situations where we need to have a single instance of the object.
- Now, to consider another example, we can design Cache Management to use and leverage on Singleton design pattern as we can handle reads and writes to external caches using the Single Cache instance object.
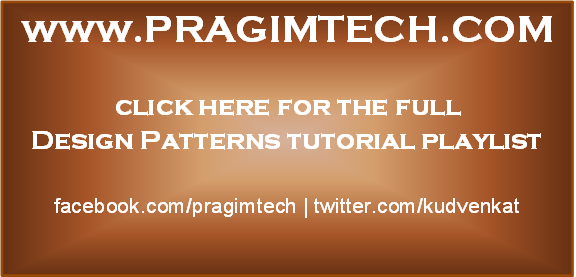
Thanks for explaining singleton, but what will be the advantage of using singleton over static class for log class, please explain. I remember using a logger third part tool which exposes static class implementation. Please explain if I got the concept wrong.
ReplyDelete@rajiv, A static class cannot implement an interface. And in this example we have implemented an interface
Delete