Suggested Video Tutorials
Part 43 - Bootstrap tooltip
Part 44 - Bootstrap tooltip manual trigger
Part 45 - Bootstrap popover
In this video we will discuss the Bootstrap alert plugin.
Creating an alert with Bootstrap is easy. All we need to do is create a <div> element with classes alert and one of the following contextual state classes.
At the moment there is no way to close the alert. To be able to close the alert, include an anchor element inside the alert <div> as shown below. Clicking on the little x on the top right hand corner will close the alert.
If you want the alert to be hidden on the initial page load include the collapse class on the alert <div>. The alert should be shown only when we click the submit button.
HTML
jQuery code
At this point when you click the button, the alert will be shown. The fade option will animate the alert to fade. If you dismiss the alert by clicking on the little x button, the alert will be removed from the DOM, and clicking on the button again will not display the alert.
If you do not want the alert to be removed from the DOM, make the following changes.
1. Remove data-dismiss="alert" attribute from the anchor element
2. Provide an id to the anchor element. I have set it to linkClose
3. Add a click event handler for the anchor element, and call hide() method on the alert <div>. This will hide the alert <div> but will not remove it from the DOM. At this point, when you click on the button again, the alert will be displayed.
HTML
jQuery
Let's take this alert one more step further. Here is what we want to do. When we click the "Submit" button
1. The alert should be displayed
2. The alert should automatically close after 2 seconds, if we do not close it by clicking on the little x button
3. However, if we click the x button before 2 seconds, the alert should close.
To achieve this modify the jQuery code as shown below. Notice here we are using setTimeout() function to automatically hide the alert <div> after 2 seconds.
Part 43 - Bootstrap tooltip
Part 44 - Bootstrap tooltip manual trigger
Part 45 - Bootstrap popover
In this video we will discuss the Bootstrap alert plugin.
Creating an alert with Bootstrap is easy. All we need to do is create a <div> element with classes alert and one of the following contextual state classes.
alert contextual classes |
---|
alert-danger |
alert-success |
alert-info |
alert-warning |

<div class="alert
alert-danger">
<strong>Error!</strong> There is a problem
submitting your form
</div>
At the moment there is no way to close the alert. To be able to close the alert, include an anchor element inside the alert <div> as shown below. Clicking on the little x on the top right hand corner will close the alert.
<div class="alert
alert-danger">
<a href="#" class="close" data-dismiss="alert">×</a>
<strong>Error!</strong> There is a problem
submitting your form
</div>
If you want the alert to be hidden on the initial page load include the collapse class on the alert <div>. The alert should be shown only when we click the submit button.

HTML
<button id="btnSubmit" class="btn
btn-primary">
Submit
</button>
<br /><br />
<div id="myAlert" class="alert alert-danger
collapse">
<a href="#" class="close" data-dismiss="alert">×</a>
<strong>Error!</strong> There is a problem
submitting your form
</div>
<script type="text/javascript">
$(document).ready(function () {
$('#btnSubmit').click(function () {
$('#myAlert').show('fade');
});
});
</script>
At this point when you click the button, the alert will be shown. The fade option will animate the alert to fade. If you dismiss the alert by clicking on the little x button, the alert will be removed from the DOM, and clicking on the button again will not display the alert.
If you do not want the alert to be removed from the DOM, make the following changes.
1. Remove data-dismiss="alert" attribute from the anchor element
2. Provide an id to the anchor element. I have set it to linkClose
3. Add a click event handler for the anchor element, and call hide() method on the alert <div>. This will hide the alert <div> but will not remove it from the DOM. At this point, when you click on the button again, the alert will be displayed.
HTML
<button id="btnSubmit" class="btn
btn-primary">
Submit
</button>
<br /><br />
<div id="myAlert" class="alert alert-danger
collapse">
<a id="linkClose" href="#" class="close">×</a>
<strong>Error!</strong> There is a problem
submitting your form
</div>
jQuery
<script type="text/javascript">
$(document).ready(function () {
$('#btnSubmit').click(function () {
$('#myAlert').show('fade');
});
$('#linkClose').click(function () {
$('#myAlert').hide('fade');
});
});
</script>
Let's take this alert one more step further. Here is what we want to do. When we click the "Submit" button
1. The alert should be displayed
2. The alert should automatically close after 2 seconds, if we do not close it by clicking on the little x button
3. However, if we click the x button before 2 seconds, the alert should close.
To achieve this modify the jQuery code as shown below. Notice here we are using setTimeout() function to automatically hide the alert <div> after 2 seconds.
<script type="text/javascript">
$(document).ready(function () {
$('#btnSubmit').click(function () {
$('#myAlert').show('fade');
setTimeout(function () {
$('#myAlert').hide('fade');
}, 2000);
});
$('#linkClose').click(function () {
$('#myAlert').hide('fade');
});
});
</script>
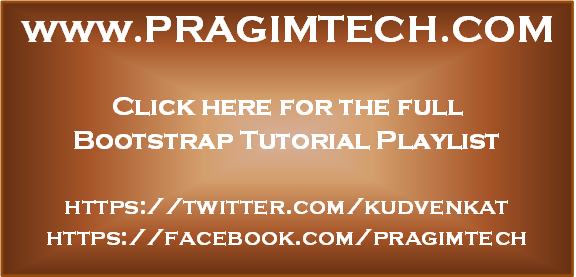
No comments:
Post a Comment
It would be great if you can help share these free resources