Suggested Videos
Part 55 - jquery load callback function
Part 56 - jquery ajax get function
Part 57 - load json data using jquery ajax
In this video we will discuss how to load XML data from the server using jQuery get function. This is continuation to Part 57, please watch Part 57 before proceeding.
The following steps modify the example we worked with in Part 57, so that HtmlPage1.html will be able to retrieve and display XML data from the server.
Step 1 : Modify the code in GetHelpText.aspx.cs as shown below.
Step 2 : Modify the jQuery code in HtmlPage1.html as shown below
Please Note :
1. Use fiddler to inspect the data that is sent to and received from GetHelpText.aspx
2. Type the following URL in the browser to see the generated XML
http://localhost:PortNumber/GetHelpText.aspx?HelpTextKey=firstName
Part 55 - jquery load callback function
Part 56 - jquery ajax get function
Part 57 - load json data using jquery ajax
In this video we will discuss how to load XML data from the server using jQuery get function. This is continuation to Part 57, please watch Part 57 before proceeding.
The following steps modify the example we worked with in Part 57, so that HtmlPage1.html will be able to retrieve and display XML data from the server.
Step 1 : Modify the code in GetHelpText.aspx.cs as shown below.
using System;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Xml.Serialization;
namespace Demo
{
public partial class GetHelpText : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
Response.ContentType = "text/xml";
XmlSerializer xmlSerializer = new XmlSerializer(typeof(HelpText));
xmlSerializer.Serialize(Response.OutputStream,
GetHelpTextByKey(Request["HelpTextKey"]));
}
private HelpText GetHelpTextByKey(string key)
{
HelpText helpText = new HelpText();
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("spGetHelpTextByKey", con);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter parameter = new SqlParameter("@HelpTextKey", key);
cmd.Parameters.Add(parameter);
con.Open();
helpText.Text =
cmd.ExecuteScalar().ToString();
helpText.Key = key;
}
return helpText;
}
}
public class HelpText
{
public string Key { get; set; }
public string Text { get; set; }
}
}
Step 2 : Modify the jQuery code in HtmlPage1.html as shown below
$(document).ready(function () {
var textBoxes = $('input[type="text"]');
textBoxes.focus(function () {
var helpDiv = $(this).attr('id');
$.get('GetHelpText.aspx', { HelpTextKey: helpDiv }, function (response) {
var jQueryXml = $(response);
var textElement = jQueryXml.find("Text");
$('#' + helpDiv + 'HelpDiv').html(textElement.text());
}, 'xml');
});
textBoxes.blur(function () {
var helpDiv = $(this).attr('id') + 'HelpDiv';
$('#' + helpDiv).html('');
});
});
Please Note :
1. Use fiddler to inspect the data that is sent to and received from GetHelpText.aspx
2. Type the following URL in the browser to see the generated XML
http://localhost:PortNumber/GetHelpText.aspx?HelpTextKey=firstName
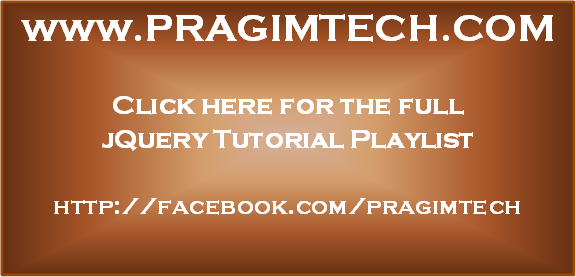
I am not getting xml back
ReplyDelete