Suggested Videos
Part 59 - jquery make a post request
Part 60 - jquery ajax method
Part 61 - Calling asp.net web services using jquery ajax
In this video we will discuss
1. How to make an asp.net web service return JSON data
2. Consume JSON data returned by the web service using jQuery AJAX
This is continuation to Part 61, please watch Part 61 before proceeding.
There are 2 approaches to make an asp.net web service return JSON data and consume it using jQuery AJAX.
Approach 1 : With Approach 1, the web service code does not change in any way.
We need to add/change the following options of the jquery request object.
1. Add contentType option and set it to application/json; charset=utf-8 to specify that we will be sending a JSON string.
2. Use JSON.stringify() method to convert the data you are sending to the server to a JSON string
3. Change the dataType to json, to specify that you are expecting JSON data from the server
4. Finally modify the success function to display Name, Gender and Salary property values from the JSON object.
Please note : By defaut, the JSON object returned by the web service has a property d. So to retrieve Name property value, use data.d.Name.
Approach 2 : With Approach 2 both the web service code and the jQuery code need to change.
Modify the ASP.NET web service as shown below to return JSON data
1. Serialize the employee object to JSON string using JavaScriptSerializer and write it to the response stream.
2. Since the method is not returning anything set the return type of the method to void.
Modify the jQuery code in HtmlPage1.html as shown below.
1. contentType option is no longer required, so you may remove it.
2. You don't have to convert the data that you are sending to the server to JSON string, so you may remove JSON.stringify() method
3. The JSON object returned from the server will not have property d, so you don't have to use d on the data object. For example, you can now retrieve Name property simply by using data.Name.
In our next video we will discuss how to call an asp.net web service that returns JSON arrays using jQuery AJAX.
Part 59 - jquery make a post request
Part 60 - jquery ajax method
Part 61 - Calling asp.net web services using jquery ajax
In this video we will discuss
1. How to make an asp.net web service return JSON data
2. Consume JSON data returned by the web service using jQuery AJAX
This is continuation to Part 61, please watch Part 61 before proceeding.
There are 2 approaches to make an asp.net web service return JSON data and consume it using jQuery AJAX.
Approach 1 : With Approach 1, the web service code does not change in any way.
We need to add/change the following options of the jquery request object.
1. Add contentType option and set it to application/json; charset=utf-8 to specify that we will be sending a JSON string.
2. Use JSON.stringify() method to convert the data you are sending to the server to a JSON string
3. Change the dataType to json, to specify that you are expecting JSON data from the server
4. Finally modify the success function to display Name, Gender and Salary property values from the JSON object.
Please note : By defaut, the JSON object returned by the web service has a property d. So to retrieve Name property value, use data.d.Name.
$(document).ready(function () {
$('#btnGetEmployee').click(function () {
var empId = $('#txtId').val();
$.ajax({
url: 'EmployeeService.asmx/GetEmployeeById',
contentType: "application/json;
charset=utf-8",
data:
JSON.stringify({ employeeId: empId }),
dataType: "json",
method: 'post',
success: function (data) {
$('#txtName').val(data.d.Name);
$('#txtGender').val(data.d.Gender);
$('#txtSalary').val(data.d.Salary);
},
error: function (err) {
alert(err);
}
});
});
});
Approach 2 : With Approach 2 both the web service code and the jQuery code need to change.
Modify the ASP.NET web service as shown below to return JSON data
1. Serialize the employee object to JSON string using JavaScriptSerializer and write it to the response stream.
2. Since the method is not returning anything set the return type of the method to void.
using System;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Web.Script.Serialization;
using System.Web.Script.Services;
using System.Web.Services;
namespace Demo
{
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class EmployeeService : System.Web.Services.WebService
{
[WebMethod]
public void GetEmployeeById(int employeeId)
{
Employee employee = new Employee();
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("spGetEmployeeById", con);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter parameter = new SqlParameter();
parameter.ParameterName = "@Id";
parameter.Value = employeeId;
cmd.Parameters.Add(parameter);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
employee.ID = Convert.ToInt32(rdr["Id"]);
employee.Name = rdr["Name"].ToString();
employee.Gender = rdr["Gender"].ToString();
employee.Salary = Convert.ToInt32(rdr["Salary"]);
}
}
JavaScriptSerializer js = new JavaScriptSerializer();
Context.Response.Write(js.Serialize(employee));
}
}
}
Modify the jQuery code in HtmlPage1.html as shown below.
1. contentType option is no longer required, so you may remove it.
2. You don't have to convert the data that you are sending to the server to JSON string, so you may remove JSON.stringify() method
3. The JSON object returned from the server will not have property d, so you don't have to use d on the data object. For example, you can now retrieve Name property simply by using data.Name.
$(document).ready(function () {
$('#btnGetEmployee').click(function () {
var empId = $('#txtId').val();
$.ajax({
url: 'EmployeeService.asmx/GetEmployeeById',
data: { employeeId: empId },
dataType: "json",
method: 'post',
success: function (data) {
$('#txtName').val(data.Name);
$('#txtGender').val(data.Gender);
$('#txtSalary').val(data.Salary);
},
error: function (err) {
alert(err);
}
});
});
});
In our next video we will discuss how to call an asp.net web service that returns JSON arrays using jQuery AJAX.
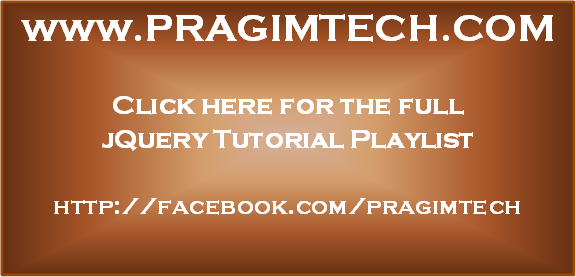
Hi Sir,
ReplyDeleteCan u please tell me. How to work with a url where the service will build a query string and handle the json data ?
Thanks,
Lokesh
sir could you please tell me how to populate the json return result to asp.net server controls. if i use html controls the control gets populated with result.
ReplyDeleteThanks
Saravanan
Hello sir,
ReplyDeletei have faced a bit of problem while executing approach 2,
inside the ready function data was not received as json.
then i tried to parse it in json.
then-after i got output on web page.
I am posting the code here.
$(document).ready(function () {
$('#btnGetEmployee').click(function () {
var empId = $("#txtId").val();
$.ajax({
url: 'EmployeeServiceJson.asmx/GetEmployeeById',
data: { employeeId: empId },
method: 'post',
datatype: "json",
success: function (data) {
var obj= JSON.parse(data);
$('#txtName').val(obj.Name);
$('#txtGender').val(obj.Gender);
$('#txtSalary').val(obj.Salary);
},
error: function (err) {
alert(err);
}
});
});
});