Suggested Videos
Part 60 - jquery ajax method
Part 61 - Calling asp.net web services using jquery ajax
Part 62 - Handling json data returned from asp.net web services
In this video we will discuss how to handle JSON arrays returned from asp.net web service using jQuery.
When we click Get All Employees button, we want to retrieve all the Employees from the database table and display on the page using jQuery.
This is continuation to Part 62, please watch Part 62 before proceeding.
Step 1 : Copy and paste the following code in EmployeeService.asmx.cs
Step 2 : Copy and paste the following HTML and jQuery code in HtmlPage1.html
Part 60 - jquery ajax method
Part 61 - Calling asp.net web services using jquery ajax
Part 62 - Handling json data returned from asp.net web services
In this video we will discuss how to handle JSON arrays returned from asp.net web service using jQuery.
When we click Get All Employees button, we want to retrieve all the Employees from the database table and display on the page using jQuery.

This is continuation to Part 62, please watch Part 62 before proceeding.
Step 1 : Copy and paste the following code in EmployeeService.asmx.cs
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data.SqlClient;
using System.Web.Script.Serialization;
using System.Web.Script.Services;
using System.Web.Services;
namespace Demo
{
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class EmployeeService : System.Web.Services.WebService
{
[WebMethod]
public void GetAllEmployees()
{
List<Employee> listEmployees = new List<Employee>();
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("Select * from
tblEmployee", con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Employee employee = new Employee();
employee.ID = Convert.ToInt32(rdr["Id"]);
employee.Name = rdr["Name"].ToString();
employee.Gender = rdr["Gender"].ToString();
employee.Salary = Convert.ToInt32(rdr["Salary"]);
listEmployees.Add(employee);
}
}
JavaScriptSerializer js = new JavaScriptSerializer();
Context.Response.Write(js.Serialize(listEmployees));
}
}
}
Step 2 : Copy and paste the following HTML and jQuery code in HtmlPage1.html
<html>
<head>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('#btnGetAllEmployees').click(function () {
$.ajax({
url: 'EmployeeService.asmx/GetAllEmployees',
dataType: "json",
method: 'post',
success: function (data) {
var employeeTable = $('#tblEmployee tbody');
employeeTable.empty();
$(data).each(function (index, emp) {
employeeTable.append('<tr><td>' + emp.ID + '</td><td>'
+ emp.Name + '</td><td>' +
emp.Gender
+ '</td><td>' +
emp.Salary + '</td></tr>');
});
},
error: function (err) {
alert(err);
}
});
});
});
</script>
</head>
<body style="font-family:Arial">
<input type="button" id="btnGetAllEmployees" value="Get All Employees" />
<br /><br />
<table id="tblEmployee" border="1" style="border-collapse:collapse">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Gender</th>
<th>Salary</th>
</tr>
</thead>
<tbody></tbody>
</table>
</body>
</html>
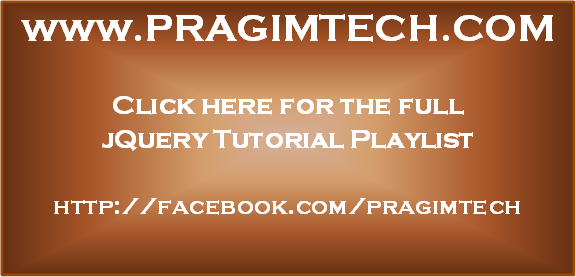
Sir Could you please upload a video how we can insert the Json Arry into sql Server table Using webservice
ReplyDeleteThanks
i think we can use sql bulk copy class by the right answer i could not found on internet
ReplyDelete