Suggested Videos
Part 54 - Object literal vs object constructor
Part 55 - Global namespace pollution in JavaScript
Part 56 - Namespaces in JavaScript
In any object oriented programming language, classes can have private and public members. For example a class in C# can have private and public fields and functions.
An example is shown below.
JavaScript is object oriented programming language, so objects in JavaScript can also have private and public fields and functions. An example is shown below.
In the example above, we also have a privileged Function. So, what is a Privileged Function?
1. Privileged methods are created using "this" keyword and Public methods are created using prototype property of the constructor function.
2. Privileged method can access private variables and methods
3. Public methods can call Privileged methods but not Private methods.
4. Like Public methods, Privileged methods are also available outside the constructor function.
Public fields and functions are available both inside and outside of the Employee() constructor function. Private fields and functions are available only inside the Employee() constructor function. Attempting to access private fields and properties outside of the constructor function will result in undefined error.
Can we modify a private field outside of the constructor function?
Straight answer, no you can't.
In the example below, when we call the private field (employee.privateFullName) it results in undefined error. On the next line we are adding a new public field with same name as the private field to the employee object. Is this going to change the private field (privateFullName). The answer is NO. You cannot access or modify private fields outside of the object. In this example, you are just adding new public field (employee.privateFullName) to the employee object.
Here is the summary
Private fields - Declared using the var keyword inside the object, and can only be accessed by private functions and privileged methods.
Public fields - Declared using this keyword and are available outside the object.
Private functions - Declared inside the object using one of the 2 ways shown below. Can be called only by privileged methods.
var privateGetFullName = function ()
{
privateFullName = firstName + " " + lastName;
return privateFullName;
}
OR
function privateGetFullName()
{
privateFullName = firstName + " " + lastName;
return privateFullName;
}
Privileged methods - Declared using this keyoword and are available both within and outside the object.
// Privileged Function
this.privilegedGetFullName = function ()
{
return privateGetFullName();
}
Public methods - Defined by using the object's prototype property and are available both within and outside the object.
// Public Function
Employee.prototype.publicGetFullName = function ()
{
return this.privilegedGetFullName();
}
Part 54 - Object literal vs object constructor
Part 55 - Global namespace pollution in JavaScript
Part 56 - Namespaces in JavaScript
In any object oriented programming language, classes can have private and public members. For example a class in C# can have private and public fields and functions.
An example is shown below.
public class Employee
{
// Private Field
private string privateFullName;
// Public Fields
public string firstName;
public string lastName;
// Private Function
private string privateGetFullName()
{
privateFullName = this.firstName
+ " " + this.lastName;
return privateFullName;
}
// Public Function
public string publicGetFullName()
{
return privateGetFullName();
}
}
JavaScript is object oriented programming language, so objects in JavaScript can also have private and public fields and functions. An example is shown below.
function Employee(firstName, lastName)
{
// Private Field
var privateFullName;
// Public Fields
this.firstName = firstName;
this.lastname = lastName;
// Private Function
var privateGetFullName = function
()
{
privateFullName = firstName + " " + lastName;
return privateFullName;
}
// Privileged Function
this.privilegedGetFullName = function
()
{
return privateGetFullName();
}
// Public Function
Employee.prototype.publicGetFullName =
function ()
{
return this.privilegedGetFullName();
}
}
In the example above, we also have a privileged Function. So, what is a Privileged Function?
1. Privileged methods are created using "this" keyword and Public methods are created using prototype property of the constructor function.
2. Privileged method can access private variables and methods
3. Public methods can call Privileged methods but not Private methods.
4. Like Public methods, Privileged methods are also available outside the constructor function.
Public fields and functions are available both inside and outside of the Employee() constructor function. Private fields and functions are available only inside the Employee() constructor function. Attempting to access private fields and properties outside of the constructor function will result in undefined error.
var employee = new
Employee("Tom", "Grover");
// Calling public function works
fine
document.write(employee.publicGetFullName()
+ "<br/>");
// Calling Privileged function
works fine
document.write(employee.privilegedGetFullName()
+ "<br/>");
// Calling private method -
undefined error
employee.privateGetFullName()
// Calling private field -
undefined error
employee.privateFullName
Can we modify a private field outside of the constructor function?
Straight answer, no you can't.
In the example below, when we call the private field (employee.privateFullName) it results in undefined error. On the next line we are adding a new public field with same name as the private field to the employee object. Is this going to change the private field (privateFullName). The answer is NO. You cannot access or modify private fields outside of the object. In this example, you are just adding new public field (employee.privateFullName) to the employee object.
var employee = new
Employee("Tom", "Grover");
employee.privateFullName // Calling private field - undefined error
// Add a field with same name as
private field
employee.privateFullName = "ABC";
document.write(employee.publicGetFullName()
+ "<br/>");
document.write(employee.privateFullName);
Here is the summary
Private fields - Declared using the var keyword inside the object, and can only be accessed by private functions and privileged methods.
Public fields - Declared using this keyword and are available outside the object.
Private functions - Declared inside the object using one of the 2 ways shown below. Can be called only by privileged methods.
var privateGetFullName = function ()
{
privateFullName = firstName + " " + lastName;
return privateFullName;
}
OR
function privateGetFullName()
{
privateFullName = firstName + " " + lastName;
return privateFullName;
}
Privileged methods - Declared using this keyoword and are available both within and outside the object.
// Privileged Function
this.privilegedGetFullName = function ()
{
return privateGetFullName();
}
Public methods - Defined by using the object's prototype property and are available both within and outside the object.
// Public Function
Employee.prototype.publicGetFullName = function ()
{
return this.privilegedGetFullName();
}
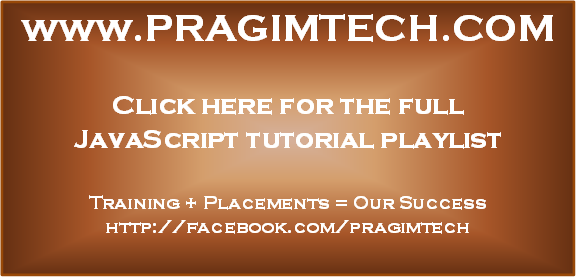
No comments:
Post a Comment
It would be great if you can help share these free resources