Suggested Videos
Part 53 - JavaScript and object oriented programming
Part 54 - Object literal vs object constructor
Part 55 - Global namespace pollution in JavaScript
In this video, we will discuss how to use namespaces to avoid polluting the global scope. This is continuation to Part 55. Please watch Part 55 of JavaScript tutorial to understand the problem of global namespace pollution.
JavaScript lack namespaces. However we can use objects to create namespaces.
The following line says use the PragimTech object if it already exists, otherwise create an empty object.
var PragimTech = PragimTech || {};
The following line adds a nested namespace. A nested namespace is a namespace inside another namespace. In JavaScript to define a nested namespace, you define an object inside another object.
PragimTech.TeamA = PragimTech.TeamA || {};
Modify the script in TeamA.js file as shown below. In this example we are adding customer() function to PragimTech.TeamA namespace.
var PragimTech = PragimTech || {};
PragimTech.TeamA = PragimTech.TeamA || {};
PragimTech.TeamA.customer = function (firstName, lastName)
{
this.firstName = firstName;
this.lastName = lastName;
this.getFullName = function ()
{
return this.firstName + " " + this.lastName;
}
return this;
}
PragimTech will be added to the global namespace. window is the alias for global namespace in JavaScript. You can now access customer() function as shown below.
PragimTech.TeamA.customer("Tom", "Grover")
OR
window.PragimTech.TeamA.customer("Tom", "Grover")
Modify the script in TeamB.js file as shown below. In this example we are adding customer() function to PragimTech.TeamB namespace.
var PragimTech = PragimTech || {};
PragimTech.TeamB = PragimTech.TeamB || {};
PragimTech.TeamB.customer = function (firstName, middleName, lastName)
{
this.firstName = firstName;
this.middleName = middleName;
this.lastName = lastName;
this.getFullName = function ()
{
return this.firstName + " " + this.middleName + " " + this.lastName;
}
return this;
}
PragimTech will be added to the global namespace. You can now access customer() function as shown below.
PragimTech.TeamB.customer("Tom", "T", "Grover")
OR
window.PragimTech.TeamB.customer("Tom", "T", "Grover")
On any given HTML page you should be able to access both the versions of customer() function as shown below.
Part 53 - JavaScript and object oriented programming
Part 54 - Object literal vs object constructor
Part 55 - Global namespace pollution in JavaScript
In this video, we will discuss how to use namespaces to avoid polluting the global scope. This is continuation to Part 55. Please watch Part 55 of JavaScript tutorial to understand the problem of global namespace pollution.
JavaScript lack namespaces. However we can use objects to create namespaces.
The following line says use the PragimTech object if it already exists, otherwise create an empty object.
var PragimTech = PragimTech || {};
The following line adds a nested namespace. A nested namespace is a namespace inside another namespace. In JavaScript to define a nested namespace, you define an object inside another object.
PragimTech.TeamA = PragimTech.TeamA || {};
Modify the script in TeamA.js file as shown below. In this example we are adding customer() function to PragimTech.TeamA namespace.
var PragimTech = PragimTech || {};
PragimTech.TeamA = PragimTech.TeamA || {};
PragimTech.TeamA.customer = function (firstName, lastName)
{
this.firstName = firstName;
this.lastName = lastName;
this.getFullName = function ()
{
return this.firstName + " " + this.lastName;
}
return this;
}
PragimTech will be added to the global namespace. window is the alias for global namespace in JavaScript. You can now access customer() function as shown below.
PragimTech.TeamA.customer("Tom", "Grover")
OR
window.PragimTech.TeamA.customer("Tom", "Grover")
Modify the script in TeamB.js file as shown below. In this example we are adding customer() function to PragimTech.TeamB namespace.
var PragimTech = PragimTech || {};
PragimTech.TeamB = PragimTech.TeamB || {};
PragimTech.TeamB.customer = function (firstName, middleName, lastName)
{
this.firstName = firstName;
this.middleName = middleName;
this.lastName = lastName;
this.getFullName = function ()
{
return this.firstName + " " + this.middleName + " " + this.lastName;
}
return this;
}
PragimTech will be added to the global namespace. You can now access customer() function as shown below.
PragimTech.TeamB.customer("Tom", "T", "Grover")
OR
window.PragimTech.TeamB.customer("Tom", "T", "Grover")
On any given HTML page you should be able to access both the versions of customer() function as shown below.
<html>
<head>
<script type="text/javascript" src="TeamA.js" ></script>
<script type="text/javascript" src="TeamB.js" ></script>
</head>
<body>
<script type="text/javascript">
// Call the customer function that
is defined in TeamA.js
alert(PragimTech.TeamA.customer("Tom", "Grover").getFullName());
// Call the customer function that
is defined in TeamB.js
alert(PragimTech.TeamB.customer("Tom", "T",
"Grover").getFullName());
</script>
</body>
</html>
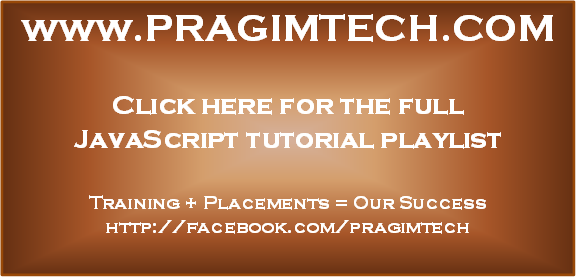
No comments:
Post a Comment
It would be great if you can help share these free resources