Suggested Videos
Part 41 - Image gallery with thumbnails in JavaScript
Part 42 - JavaScript event capturing
Part 43 - Preventing browser default action
In this video, we will discuss how to use JavaScript and detect which mouse button is clicked.
Depending on the browser, event.button or event.which properties of the event object are used to determine which mouse button is clicked.
IE 8 & earlier versions use event.button property
IE 9 & later versions and most other W3C compliant browsers use event.which property
Depending on the browser used, the following code returns left, middle and right click codes.
When the above script is tested with Google chrome or IE 9 (or later version), I get the following output. Notice that these browsers support event.which property.
When the above script is tested with IE 8 (or earlier version), I get the following output. Notice that IE & earlier versions support event.button property.
The following JavaScript code detects which mouse button is clicked. It works in all versions of IE and most other W3C complaint browsers.
Part 41 - Image gallery with thumbnails in JavaScript
Part 42 - JavaScript event capturing
Part 43 - Preventing browser default action
In this video, we will discuss how to use JavaScript and detect which mouse button is clicked.
Depending on the browser, event.button or event.which properties of the event object are used to determine which mouse button is clicked.
IE 8 & earlier versions use event.button property

IE 9 & later versions and most other W3C compliant browsers use event.which property

Depending on the browser used, the following code returns left, middle and right click codes.
<input type="button" value="Click Me" onmouseup="getMouseClickCode(event)" />
<input type="button" value="Clear" onclick="clearText()" />
<br />
<br />
<textarea id="txtArea" rows="3" cols="10"></textarea>
<script type="text/javascript">
function clearText()
{
document.getElementById("txtArea").value = "";
}
function getMouseClickCode(event)
{
if (event.which)
{
document.getElementById("txtArea").value += "event.which = " +
event.which
+ "\r\n";
}
else
{
document.getElementById("txtArea").value += "event.button = " +
event.button + "\r\n";
}
}
document.oncontextmenu
= disableRightClick;
function disableRightClick(event)
{
event = event || window.event;
if (event.preventDefault)
{
event.preventDefault();
}
else
{
event.returnValue = false
}
}
</script>
When the above script is tested with Google chrome or IE 9 (or later version), I get the following output. Notice that these browsers support event.which property.

When the above script is tested with IE 8 (or earlier version), I get the following output. Notice that IE & earlier versions support event.button property.

The following JavaScript code detects which mouse button is clicked. It works in all versions of IE and most other W3C complaint browsers.
<script type="text/javascript">
function whichMouseButtonClicked(event)
{
var whichButton;
if (event.which)
{
switch (event.which)
{
case 1:
whichButton = "Left Button Clicked";
break;
case 2:
whichButton = "Middle Button Clicked";
break;
case 3:
whichButton = "Right Button Clicked";
break;
default:
whichButton = "Invalid Button Clicked";
break;
}
}
else
{
switch (event.button)
{
case 1:
whichButton = "Left Button Clicked";
break;
case 4:
whichButton = "Middle Button Clicked";
break;
case 2:
whichButton = "Right Button Clicked";
break;
default:
whichButton = "Invalid Button Clicked";
break;
}
}
alert(whichButton);
}
document.oncontextmenu = disableRightClick;
function disableRightClick(event)
{
event = event || window.event;
if (event.preventDefault)
{
event.preventDefault();
}
else
{
event.returnValue = false
}
}
</script>
<button onmouseup="whichMouseButtonClicked(event)">Click Me</button>
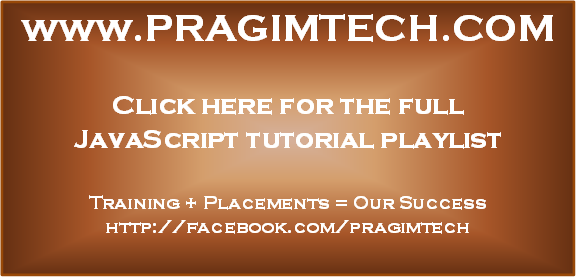
No comments:
Post a Comment
It would be great if you can help share these free resources