Suggested Videos
Part 39 - JavaScript event object
Part 40 - Event bubbling in JavaScript
Part 41 - Image gallery with thumbnails in JavaScript
Event capturing is the opposite of event bubbling. We discussed event bubbling in detail in Part 40 of JavaScript tutorial.
With event bubbling the event bubbles up from the inner most element to the outer most element in the DOM hierarchy. With event capuring the opposite happens, the event gets captured from the outer most element to innermost element. In real world event capturing is rarely used. Let us understand this with an example.
In the following example we have 3 <div> nested elements. Notice that we are using addEventListener() method to assign event handler to each <div> element. To enable event capturing we have set the third parameter (phase) of addEventListener() method to true. Now when you click on the innermost <div> (DIV3), notice that the events are fired from the outermost <div> to innermost <div> (DIV1 => DIV 2 => DIV 3).
Please note : IE8 and earlier versions does not support addEventListener() method. This implies that event capturing is not supported in IE8 and earlier versions, and hence the above code will not work in IE 8 and earlier versions.
Stopping event capturing : Stopping event capturing is very similar to stopping event bubbling. With the example below, when you click on any <div> element notice that DIV 1 element handles the click event and it does not get captured down.
Using addEventListener() method with last argument set to true is the only way to enable event capturing. If the thid parameter (phase) is set to true event capturing is enabled and if it is set to false event bubbling is enabled. If you want both even bubbling and capturing to be enabled, then assign handlers 2 times, once with the phase parameter set to false and once with the phase parameter set to true as shown below.
With both event capturing and bubbling enabled, events are first captured from the outermost element to the innermost element and then bubbles up from the innermost to the outermost element.
Part 39 - JavaScript event object
Part 40 - Event bubbling in JavaScript
Part 41 - Image gallery with thumbnails in JavaScript
Event capturing is the opposite of event bubbling. We discussed event bubbling in detail in Part 40 of JavaScript tutorial.
With event bubbling the event bubbles up from the inner most element to the outer most element in the DOM hierarchy. With event capuring the opposite happens, the event gets captured from the outer most element to innermost element. In real world event capturing is rarely used. Let us understand this with an example.
In the following example we have 3 <div> nested elements. Notice that we are using addEventListener() method to assign event handler to each <div> element. To enable event capturing we have set the third parameter (phase) of addEventListener() method to true. Now when you click on the innermost <div> (DIV3), notice that the events are fired from the outermost <div> to innermost <div> (DIV1 => DIV 2 => DIV 3).
<html>
<head>
<style type="text/css">
.divStyle
{
display: table-cell;
border: 5px solid black;
padding: 20px;
text-align: center;
}
</style>
</head>
<body>
<div id="DIV1" class="divStyle">
DIV 1
<div id="DIV2" class="divStyle">
DIV 2
<div id="DIV3" class="divStyle">
DIV 3
</div>
</div>
</div>
<script type="text/javascript">
var divElements = document.getElementsByTagName('div');
for (var i =
0; i < divElements.length; i++)
{
divElements[i].addEventListener("click", clickHandler, true);
}
function clickHandler()
{
alert(this.getAttribute("id") + " click event handled");
}
</script>
</body>
</html>
Please note : IE8 and earlier versions does not support addEventListener() method. This implies that event capturing is not supported in IE8 and earlier versions, and hence the above code will not work in IE 8 and earlier versions.
Stopping event capturing : Stopping event capturing is very similar to stopping event bubbling. With the example below, when you click on any <div> element notice that DIV 1 element handles the click event and it does not get captured down.
<html>
<head>
<style type="text/css">
.divStyle
{
display: table-cell;
border: 5px solid black;
padding: 20px;
text-align: center;
}
</style>
</head>
<body>
<div id="DIV1" class="divStyle">
DIV 1
<div id="DIV2" class="divStyle">
DIV 2
<div id="DIV3" class="divStyle">
DIV 3
</div>
</div>
</div>
<script type="text/javascript">
var divElements = document.getElementsByTagName('div');
for (var i =
0; i < divElements.length; i++)
{
divElements[i].addEventListener("click", clickHandler, true);
}
function clickHandler(event)
{
event.stopPropagation();
alert(this.getAttribute("id") + " click event handled");
}
</script>
</body>
</html>
Using addEventListener() method with last argument set to true is the only way to enable event capturing. If the thid parameter (phase) is set to true event capturing is enabled and if it is set to false event bubbling is enabled. If you want both even bubbling and capturing to be enabled, then assign handlers 2 times, once with the phase parameter set to false and once with the phase parameter set to true as shown below.
<html>
<head>
<style type="text/css">
.divStyle
{
display: table-cell;
border: 5px solid black;
padding: 20px;
text-align: center;
}
</style>
</head>
<body>
<div id="DIV1" class="divStyle">
DIV 1
<div id="DIV2" class="divStyle">
DIV 2
<div id="DIV3" class="divStyle">
DIV 3
</div>
</div>
</div>
<script type="text/javascript">
var divElements = document.getElementsByTagName('div');
for (var i =
0; i < divElements.length; i++)
{
divElements[i].addEventListener("click", clickHandler, false);
divElements[i].addEventListener("click", clickHandler, true);
}
function clickHandler()
{
alert(this.getAttribute("id") + " click event handled");
}
</script>
</body>
</html>
With both event capturing and bubbling enabled, events are first captured from the outermost element to the innermost element and then bubbles up from the innermost to the outermost element.
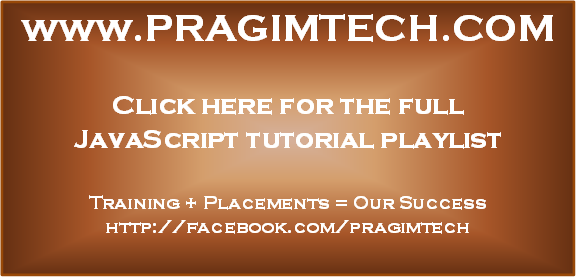
No comments:
Post a Comment
It would be great if you can help share these free resources