Suggested Videos
Part 18 - For loop in JavaScript
Part 19 - Arrays in javascript
Part 20 - JavaScript array push and pop methods
There are several methods that can be used with the array object in JavaScript. Some methods modify the array object while the others do not. The methods that modify the array object are called as mutator methods.
The following are the examples of non-mutator methods
contains
indexOf
lastIndexOf
The following are the examples of mutator methods
push
pop
shift
unshift
reverse
sort
splice
We discusssed push(), pop(), shift() and unshift() methods in Part 20. In this video we will discuss
sort
reverse
splice
JavaScript sort method : Sorts the elements of an array. By default, the sort() method sorts the values by converting them to strings and then comparing those strings. This works well for strings but not for numbers. Let us look at an example.
Example : Notice that the strings are sorted correctly as expected.
var myArray = ["Sam","Mark","Tom","David"];
myArray.sort();
document.write(myArray);
Output : David,Mark,Sam,Tom
Now, let's look at an example of sorting numbers.
var myArray = [20, 1 , 10 , 2, 3];
myArray.sort();
document.write(myArray);
Output : 1,10,2,20,3
Notice that the numbers are not sorted as expected. We can fix this by providing a "compare function" as a parameter to the sort function. The compare function should return a negative, zero, or positive value.
Example :
var myArray = [20, 1, 10, 2, 3];
myArray.sort(function (a, b) { return a - b });
document.write(myArray);
Output : 1,2,3,10,20
Let's now discuss how the compare function work. The function has 2 parameters (a,b). This function subtracts a from b and returns the result. If the return value is
So, based on these return values, the numbers in the array are sorted.
Sorting the numbers in descending order : There are 2 ways to sort an array in descending order
1. Return (b-a) from the compare function instead of (a-b)
Example :
var myArray = [20, 1, 10, 2, 3];
myArray.sort(function (a, b) { return b - a });
document.write(myArray);
Output : 20,10,3,2,1
2. Sort the numbers first in ascending order and then use the reverse function to reverse the order of the elements in the array.
Example :
var myArray = [20, 1, 10, 2, 3];
myArray.sort(function (a, b) { return a - b }).reverse();
document.write(myArray);
Output : 20,10,3,2,1
JavaScript reverse method : reverses the order of the elements in an array.
JavaScript splice method : This method is used to add or remove elements from an array.
Syntax : array.splice(index,deleteCount,item1,.....,itemX)
Example :
var myArray = [1,2,5];
myArray.splice(2, 0, 3, 4);
document.write(myArray);
Output : 1,2,3,4,5
Example :
var myArray = [1,2,55,67,3];
myArray.splice(2, 2);
document.write(myArray);
Output : 1,2,3
Part 18 - For loop in JavaScript
Part 19 - Arrays in javascript
Part 20 - JavaScript array push and pop methods
There are several methods that can be used with the array object in JavaScript. Some methods modify the array object while the others do not. The methods that modify the array object are called as mutator methods.
The following are the examples of non-mutator methods
contains
indexOf
lastIndexOf
The following are the examples of mutator methods
push
pop
shift
unshift
reverse
sort
splice
We discusssed push(), pop(), shift() and unshift() methods in Part 20. In this video we will discuss
sort
reverse
splice
JavaScript sort method : Sorts the elements of an array. By default, the sort() method sorts the values by converting them to strings and then comparing those strings. This works well for strings but not for numbers. Let us look at an example.
Example : Notice that the strings are sorted correctly as expected.
var myArray = ["Sam","Mark","Tom","David"];
myArray.sort();
document.write(myArray);
Output : David,Mark,Sam,Tom
Now, let's look at an example of sorting numbers.
var myArray = [20, 1 , 10 , 2, 3];
myArray.sort();
document.write(myArray);
Output : 1,10,2,20,3
Notice that the numbers are not sorted as expected. We can fix this by providing a "compare function" as a parameter to the sort function. The compare function should return a negative, zero, or positive value.
Example :
var myArray = [20, 1, 10, 2, 3];
myArray.sort(function (a, b) { return a - b });
document.write(myArray);
Output : 1,2,3,10,20
Let's now discuss how the compare function work. The function has 2 parameters (a,b). This function subtracts a from b and returns the result. If the return value is
Positive | a is a number bigger than b |
Negative | a is a number smaller than b |
ZERO | a is equal to b |
So, based on these return values, the numbers in the array are sorted.
Sorting the numbers in descending order : There are 2 ways to sort an array in descending order
1. Return (b-a) from the compare function instead of (a-b)
Example :
var myArray = [20, 1, 10, 2, 3];
myArray.sort(function (a, b) { return b - a });
document.write(myArray);
Output : 20,10,3,2,1
2. Sort the numbers first in ascending order and then use the reverse function to reverse the order of the elements in the array.
Example :
var myArray = [20, 1, 10, 2, 3];
myArray.sort(function (a, b) { return a - b }).reverse();
document.write(myArray);
Output : 20,10,3,2,1
JavaScript reverse method : reverses the order of the elements in an array.
JavaScript splice method : This method is used to add or remove elements from an array.
Syntax : array.splice(index,deleteCount,item1,.....,itemX)
index | Required. Specifies at what position to add or remove items |
deleteCount | Required. The number of items to be removed. If set to 0, no items will be removed. |
item1,.....,itemX | Optional. The new item(s) to be added to the array |
Example :
var myArray = [1,2,5];
myArray.splice(2, 0, 3, 4);
document.write(myArray);
Output : 1,2,3,4,5
Example :
var myArray = [1,2,55,67,3];
myArray.splice(2, 2);
document.write(myArray);
Output : 1,2,3
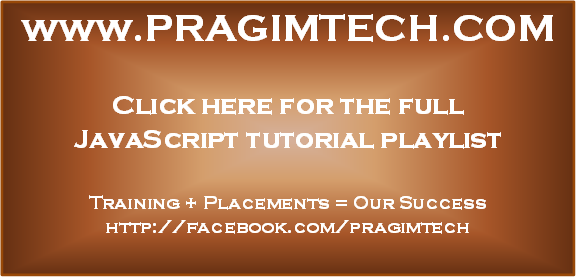
No comments:
Post a Comment
It would be great if you can help share these free resources