Suggested Videos
Part 9 - Converting strings to numbers in JavaScript
Part 10 - Strings in JavaScript
Part 11 - Substrings in JavaScript
In this video we will discuss a simple real time example of where we can use indexOf(), lastIndexOf() and substring() methods
Design the web form as shown below : This form allows the use to enter an email address and when they click "Get email & domain parts" button, we should retrieve email part and domain part from the email address and display in respective textboxes.
Here is the HTML
In the head section of the webform, include the following script section
Finally set the onclick attriibute of the button to call the JavaScript function
In Part 11 of JavaScript Tutorial we discussed indexOf() function. lastIndexOf() is also very useful function for manipulating strings.
lastIndexOf() method returns the position of the last occurrence of a specified value in a string. Since it's job is to return the last index of the specified value, this method searches the given string from the end to the beginning and returns the index of the first match it finds. This method returns -1 if the specified value is not present in the given string.
Example : Retrieve the last index position of dot (.) in the given string
Output : 42
Simple real time example where lastIndexOf and substring methods can be used
Design the web form as shown below : This form allows the use to enter a web site URL and when they click "Get top level domain" button, we should retrieve domain name and display in "Top level domian" textbox.
Here is the HTML
In the head section of the webform, include the following script section
Finally set the onclick attriibute of the button to call the JavaScript function
Part 9 - Converting strings to numbers in JavaScript
Part 10 - Strings in JavaScript
Part 11 - Substrings in JavaScript
In this video we will discuss a simple real time example of where we can use indexOf(), lastIndexOf() and substring() methods
Design the web form as shown below : This form allows the use to enter an email address and when they click "Get email & domain parts" button, we should retrieve email part and domain part from the email address and display in respective textboxes.

Here is the HTML
<table style="border:1px solid black; font-family:Arial">
<tr>
<td>
Email Address
</td>
<td>
<asp:TextBox ID="txtEmailAddress" runat="server" Width="250px">
</asp:TextBox>
</td>
</tr>
<tr>
<td>
Email Part
</td>
<td>
<asp:TextBox ID="txtEmailPart" runat="server" Width="250px"></asp:TextBox>
</td>
</tr>
<tr>
<td>
Domian Part
</td>
<td>
<asp:TextBox ID="txtDomainPart" runat="server" Width="250px"></asp:TextBox>
</td>
</tr>
<tr>
<td></td>
<td>
<input type="button" value="Get email & domain parts" style="width:250px"/>
</td>
</tr>
</table>
In the head section of the webform, include the following script section
function getEmailandDomainParts()
{
var emailAddress = document.getElementById("txtEmailAddress").value;
var emailPart = emailAddress.substring(0, emailAddress.indexOf("@"));
var domainPart
= emailAddress.substring(emailAddress.indexOf("@") + 1);
document.getElementById("txtEmailPart").value = emailPart;
document.getElementById("txtDomainPart").value =
domainPart;
}
Finally set the onclick attriibute of the button to call the JavaScript function
<input type="button" value="Get email & domain parts" style="width:250px"
onclick="getEmailandDomainParts()"/>
In Part 11 of JavaScript Tutorial we discussed indexOf() function. lastIndexOf() is also very useful function for manipulating strings.
lastIndexOf() method returns the position of the last occurrence of a specified value in a string. Since it's job is to return the last index of the specified value, this method searches the given string from the end to the beginning and returns the index of the first match it finds. This method returns -1 if the specified value is not present in the given string.
Example : Retrieve the last index position of dot (.) in the given string
var url
= "http://www.csharp-video-tutorials.blogspot.com";
alert(url.lastIndexOf("."));
Output : 42
Simple real time example where lastIndexOf and substring methods can be used
Design the web form as shown below : This form allows the use to enter a web site URL and when they click "Get top level domain" button, we should retrieve domain name and display in "Top level domian" textbox.

Here is the HTML
<table style="border: 1px solid black; font-family: Arial">
<tr>
<td>
Website URL
</td>
<td>
<asp:TextBox ID="txtURL" runat="server" Width="300px"></asp:TextBox>
</td>
</tr>
<tr>
<td>
Top level domian
</td>
<td>
<asp:TextBox ID="txtDomian" runat="server" Width="300px"></asp:TextBox>
</td>
</tr>
<tr>
<td>
</td>
<td>
<input type="button" value="Get top level domain" style="width: 300px" />
</td>
</tr>
</table>
In the head section of the webform, include the following script section
<script type="text/javascript">
function getDomainName()
{
var url = document.getElementById("txtURL").value;
var domainName = url.substr(url.lastIndexOf("."));
document.getElementById("txtDomian").value = domainName;
}
</script>
Finally set the onclick attriibute of the button to call the JavaScript function
<input type="button" value="Get top level domain" style="width: 300px"
onclick="getDomainName()" />
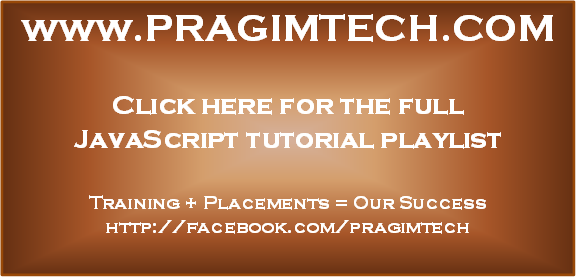
its very usefull thank you soo much venkat gaaru
ReplyDeleteYour Javascript in Asp.net and all other video tutorials are very helpful. However, I am trying to find out your Javascript in MVC tutorials. Please let me know where do I get those?
ReplyDelete