Suggested Videos
Part 29 - Concat operator in LINQ
Part 30 - SequenceEqual Operator in LINQ
Part 31 - Quantifiers in LINQ
What is LinqPad
LinqPad is a free tool that you can download from http://www.linqpad.net. It helps learn, write and test linq queries.
Copy and paste the following LINQ query in LinqPad. To execute the query, you can either press the Green Execute button on the LinqPad or press F5. Dump() method is similar to Console.WriteLine() in a console application.
Notice that the results of the query are shown in the Results window. Next to the results window, you also have the following options
1. ? (lambda Symbol) - Use this button to get the lambda equivalent of a LINQ Query
2. SQL - Shows the generated SQL statement that will be executed against the underlying database
3. IL - Shows the Intermediate Language code
For the above query, Lambda and SQL windows will not show anything. To get the Lambda equivalent of a LINQ query, use .AsQueryable() on the source collection as shown below.
AsQueryable() can also be used on the source collection as shown below.
LinqPad can execute
1. Statements
2. Expressions
3. Program
LinqPad can also be used with databases and WCF Data Services.
Adding a database connection in LinqPad
Step 1 : Click "Add connection"
Step 2 : Under LinqPad Driver, select "Default (LINQ to SQL)"
Step 3 : Click Next
Step 4 : Select "SQL Server" as the "Provider"
Step 5 : Specify the Server Name. In my case I am connecting to the local SQL Server. So I used . (DOT)
Step 6 : Select the Authentication
Step 7 : Select the Database
Step 8 : Click OK
At this point LinqPad connects to the database, and shows all the table entities. The relationships between the entities are also shown. The Green Split arrow indicates One-to-Many relationship and the Blue Split Arrow indicates Many-to-One relationship.
We can now start writing linq queries targeting the SQL Server database.
The following LINQ query fetches all the employee names that start with letter 'M' and sorts them in descending order
from e in Employees
where e.Name.StartsWith("M")
orderby e.Name descending
select e.Name
After executing the query, click on the SQL button to see the Transact-SQL that is generated.
Adding a WCF Data Services connection in LinqPad
Step 1 : Click "Add connection"
Step 2 : Under LinqPad Driver, select "WCF Data Services"
Step 3 : Click Next
Step 4 : Type the URI for the WCF Data Service. http://services.odata.org/V3/Northwind/Northwind.svc/
Step 5 : Click OK
We can now start writing linq queries targeting the WCF Data Service.
The following LINQ query fetches all the product names that start with letter 'C' and sorts them in ascending order
from p in Products
where p.ProductName.StartsWith("C")
orderby p.ProductName ascending
select p
Part 29 - Concat operator in LINQ
Part 30 - SequenceEqual Operator in LINQ
Part 31 - Quantifiers in LINQ
What is LinqPad
LinqPad is a free tool that you can download from http://www.linqpad.net. It helps learn, write and test linq queries.
Copy and paste the following LINQ query in LinqPad. To execute the query, you can either press the Green Execute button on the LinqPad or press F5. Dump() method is similar to Console.WriteLine() in a console application.
int[] numbers = { 1, 2, 3, 4,
5, 6, 7, 8, 9, 10 };
var result = from
n in numbers
where n % 2 == 0
orderby n descending
select n;
result.Dump();

Notice that the results of the query are shown in the Results window. Next to the results window, you also have the following options
1. ? (lambda Symbol) - Use this button to get the lambda equivalent of a LINQ Query
2. SQL - Shows the generated SQL statement that will be executed against the underlying database
3. IL - Shows the Intermediate Language code
For the above query, Lambda and SQL windows will not show anything. To get the Lambda equivalent of a LINQ query, use .AsQueryable() on the source collection as shown below.
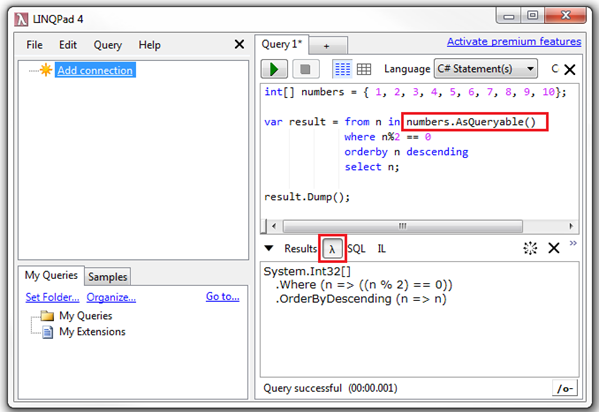
AsQueryable() can also be used on the source collection as shown below.
var numbers = new
int[] { 1, 2, 3, 4,
5, 6, 7, 8, 9, 10 }.AsQueryable();
var result = from
n in numbers
where n % 2 == 0
orderby n descending
select n;
result.Dump();
LinqPad can execute
1. Statements
2. Expressions
3. Program
LinqPad can also be used with databases and WCF Data Services.
Adding a database connection in LinqPad
Step 1 : Click "Add connection"
Step 2 : Under LinqPad Driver, select "Default (LINQ to SQL)"
Step 3 : Click Next

Step 4 : Select "SQL Server" as the "Provider"
Step 5 : Specify the Server Name. In my case I am connecting to the local SQL Server. So I used . (DOT)
Step 6 : Select the Authentication
Step 7 : Select the Database
Step 8 : Click OK

At this point LinqPad connects to the database, and shows all the table entities. The relationships between the entities are also shown. The Green Split arrow indicates One-to-Many relationship and the Blue Split Arrow indicates Many-to-One relationship.

We can now start writing linq queries targeting the SQL Server database.
The following LINQ query fetches all the employee names that start with letter 'M' and sorts them in descending order
from e in Employees
where e.Name.StartsWith("M")
orderby e.Name descending
select e.Name
After executing the query, click on the SQL button to see the Transact-SQL that is generated.

Adding a WCF Data Services connection in LinqPad
Step 1 : Click "Add connection"
Step 2 : Under LinqPad Driver, select "WCF Data Services"
Step 3 : Click Next

Step 4 : Type the URI for the WCF Data Service. http://services.odata.org/V3/Northwind/Northwind.svc/
Step 5 : Click OK

We can now start writing linq queries targeting the WCF Data Service.
The following LINQ query fetches all the product names that start with letter 'C' and sorts them in ascending order
from p in Products
where p.ProductName.StartsWith("C")
orderby p.ProductName ascending
select p
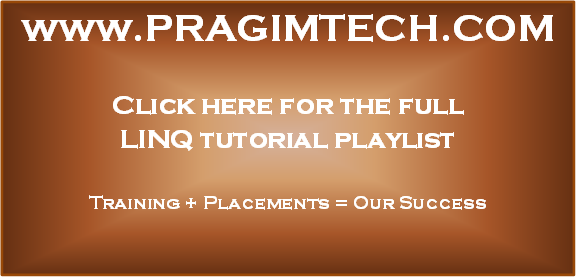
No comments:
Post a Comment
It would be great if you can help share these free resources