Suggested Videos
Part 29 - Hosting wcf service in IIS
Part 30 - Advantages and disadvantages of hosting WCF service in IIS
Part 31 - WAS hosting in WCF
In this video we will discuss, the different Message Exchange Patterns that are available in WCF.
Message Exchange Pattern describes how the client and the wcf service exchange messages. WCF supports the following 3 Message Exchange Patterns. The default Message Exchange Pattern in WCF is Request-Reply.
1. Request-Reply (Default)
2. One-Way
3. Duplex
Request-Reply:
1. This is the default Message Exchange Pattern
2. Client sends a message to a WCF Service and then waits for a reply. During this time the client client stops processing until a response is received from the wcf service.
3. The client waits for the service call to complete even if the operation return type is void.
4. All WCF bindings except the MSMQ-based bindings support the Request-Reply Message Exchange Pattern.
5. IsOneWay parameter of OperationContract attribute specifies the Message Exchange Pattern. The default value for IsOneWay parameter is false. This means that, if we don't specify this parameter, then the Message Exchange Pattern is Request/Reply. So the following 2 declarations are equivalent.
[ServiceContract]
public interface ISampleService
{
[OperationContract(IsOneWay=false)]
string RequestReplyOperation();
[OperationContract(IsOneWay = false)]
string RequestReplyOperation_ThrowsException();
}
OR
[ServiceContract]
public interface ISampleService
{
[OperationContract]
string RequestReplyOperation();
[OperationContract]
string RequestReplyOperation_ThrowsException();
}
6. In a Request-Reply message exchange pattern faults and exceptions get reported to the client immediately if any.
Service Implementation:
Create a windows client application and add a reference to the WCF service.
Desing the windows form as shown below.
Here is the client code:
Next Video: OneWay message exchange pattern
Part 29 - Hosting wcf service in IIS
Part 30 - Advantages and disadvantages of hosting WCF service in IIS
Part 31 - WAS hosting in WCF
In this video we will discuss, the different Message Exchange Patterns that are available in WCF.
Message Exchange Pattern describes how the client and the wcf service exchange messages. WCF supports the following 3 Message Exchange Patterns. The default Message Exchange Pattern in WCF is Request-Reply.
1. Request-Reply (Default)
2. One-Way
3. Duplex
Request-Reply:
1. This is the default Message Exchange Pattern
2. Client sends a message to a WCF Service and then waits for a reply. During this time the client client stops processing until a response is received from the wcf service.
3. The client waits for the service call to complete even if the operation return type is void.
4. All WCF bindings except the MSMQ-based bindings support the Request-Reply Message Exchange Pattern.
5. IsOneWay parameter of OperationContract attribute specifies the Message Exchange Pattern. The default value for IsOneWay parameter is false. This means that, if we don't specify this parameter, then the Message Exchange Pattern is Request/Reply. So the following 2 declarations are equivalent.
[ServiceContract]
public interface ISampleService
{
[OperationContract(IsOneWay=false)]
string RequestReplyOperation();
[OperationContract(IsOneWay = false)]
string RequestReplyOperation_ThrowsException();
}
OR
[ServiceContract]
public interface ISampleService
{
[OperationContract]
string RequestReplyOperation();
[OperationContract]
string RequestReplyOperation_ThrowsException();
}
6. In a Request-Reply message exchange pattern faults and exceptions get reported to the client immediately if any.
Service Implementation:
public class SampleService : ISampleService
{
// This method simply introduces 2 seconds of
artifical processing
// time using Thread.Sleep(2000) and returns
a string
public string RequestReplyOperation()
{
DateTime dtStart = DateTime.Now;
Thread.Sleep(2000);
DateTime dtEnd = DateTime.Now;
return dtEnd.Subtract(dtStart).Seconds.ToString()
+ "
seconds processing time";
}
// This method throws an
NotImplementedException exception
public string RequestReplyOperation_ThrowsException()
{
throw new NotImplementedException();
}
}
Create a windows client application and add a reference to the WCF service.
Desing the windows form as shown below.

Here is the client code:
using System;
using System.Windows.Forms;
namespace WindowsClient
{
public partial class
Form1 : Form
{
SampleService.SampleServiceClient
client;
public Form1()
{
InitializeComponent();
client = new
SampleService.SampleServiceClient();
}
private void btnRequestReplyOperation_Click
(object sender, EventArgs
e)
{
try
{
listBox1.Items.Add("Request-Reply Operation Started @ "
+ DateTime.Now.ToString());
btnRequestReplyOperation.Enabled
= false;
listBox1.Items.Add(client.RequestReplyOperation());
btnRequestReplyOperation.Enabled
= true;
listBox1.Items.Add("Request-Reply Operation Completed @ "
+ DateTime.Now.ToString());
listBox1.Items.Add("");
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void btnRequestReplyOperation_ThrowsException_Click
(object sender, EventArgs
e)
{
try
{
listBox1.Items.Add("Request-Reply Throws Exception Operation Started @
"
+ DateTime.Now.ToString());
client.RequestReplyOperation_ThrowsException();
listBox1.Items.Add("Request-Reply Throws Exception Operation Completed
@ "
+ DateTime.Now.ToString());
listBox1.Items.Add("");
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void btnClear_Click(object sender, EventArgs
e)
{
listBox1.Items.Clear();
}
}
}
Next Video: OneWay message exchange pattern
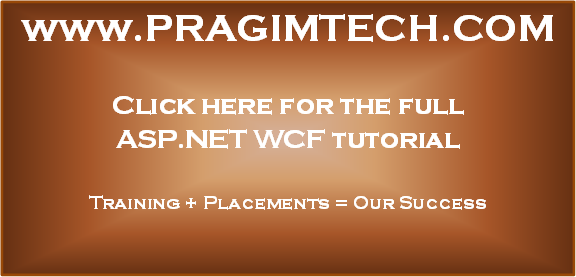
can you share sample application with this example
ReplyDeleteHi Sir,
ReplyDeleteThank You for sharing such a good video.
I have seen this video and you are telling that even in void return type client will wait until service response, but in my case when i use void type than client are free after call to service even service not response.
This is correct or not.
Please reply me.
Thanks in advance.
Mayur