Suggested Videos
Part 9 - How to enable tracing and message logging in wcf
Part 10 - Message Contract in WCF
Part 11 - Difference between datacontract and messagecontract in wcf
After a WCF service is deployed on the production server, the WSDL document should not be changed in any way that would break the existing clients. This is because the clients have already generated proxy classes and relevant configuration to interact with the service. If you intend to make changes, the changes should be done in such a way that they support backward compatibility.
WCF contracts are version tolerant by default. The DataContractSerializer which is the default engine for serialization in WCF allows missing, non-required data and ignores superfluous data for service contracts, data contracts & message contracts.
If you remove the existing operation contracts, the service would throw an exception if they try to invoke that missing operation contract.
[ServiceContract]
public interface IEmployeeService
{
[OperationContract]
Employee GetEmployee(int Id);
//[OperationContract]
//void SaveEmployee(Employee Employee);
}
If you add a new operation contract, the existing clients will not know about the new method and would continue to work in the same way as before.
[ServiceContract]
public interface IEmployeeService
{
[OperationContract]
Employee GetEmployee(int Id);
[OperationContract]
void SaveEmployee(Employee Employee);
[OperationContract]
string GetEmployeeNameById(int Id);
}
For example, removing Gender property which is not required from the DataContract will not break the existing clients. The existing clients will continue to send data for Gender property to the service. At the service side, Gender property value will simply be ignored.
[DataContract(Namespace = "http://pragimtech.com/Employee")]
public class Employee
{
[DataMember(Order = 1)]
public int Id { get; set; }
[DataMember(Order = 2)]
public string Name { get; set; }
//[DataMember(Order = 3)]
//public string Gender { get; set; }
[DataMember(Order = 4)]
public DateTime DateOfBirth { get; set; }
[DataMember(Order = 5)]
public EmployeeType Type { get; set; }
}
Along the same lines if a new non required field City is added, the existing clients will not send data for this field, and the service WILL NOT throw an exception as it is not a required field.
[DataContract(Namespace = "http://pragimtech.com/Employee")]
public class Employee
{
[DataMember(Order = 1)]
public int Id { get; set; }
[DataMember(Order = 2)]
public string Name { get; set; }
//[DataMember(Order = 3)]
//public string Gender { get; set; }
[DataMember(Order = 4)]
public DateTime DateOfBirth { get; set; }
[DataMember(Order = 5)]
public EmployeeType Type { get; set; }
[DataMember(Order = 5)]
public string City { get; set; }
}
On the other hand, if you make it a required field, the service would throw an exception.
For a full list of changes and the impact that could have on the existing clients, please refer to the following MSDN article
http://msdn.microsoft.com/en-us/library/ff384251.aspx
Part 9 - How to enable tracing and message logging in wcf
Part 10 - Message Contract in WCF
Part 11 - Difference between datacontract and messagecontract in wcf
After a WCF service is deployed on the production server, the WSDL document should not be changed in any way that would break the existing clients. This is because the clients have already generated proxy classes and relevant configuration to interact with the service. If you intend to make changes, the changes should be done in such a way that they support backward compatibility.
WCF contracts are version tolerant by default. The DataContractSerializer which is the default engine for serialization in WCF allows missing, non-required data and ignores superfluous data for service contracts, data contracts & message contracts.
If you remove the existing operation contracts, the service would throw an exception if they try to invoke that missing operation contract.
[ServiceContract]
public interface IEmployeeService
{
[OperationContract]
Employee GetEmployee(int Id);
//[OperationContract]
//void SaveEmployee(Employee Employee);
}
If you add a new operation contract, the existing clients will not know about the new method and would continue to work in the same way as before.
[ServiceContract]
public interface IEmployeeService
{
[OperationContract]
Employee GetEmployee(int Id);
[OperationContract]
void SaveEmployee(Employee Employee);
[OperationContract]
string GetEmployeeNameById(int Id);
}
For example, removing Gender property which is not required from the DataContract will not break the existing clients. The existing clients will continue to send data for Gender property to the service. At the service side, Gender property value will simply be ignored.
[DataContract(Namespace = "http://pragimtech.com/Employee")]
public class Employee
{
[DataMember(Order = 1)]
public int Id { get; set; }
[DataMember(Order = 2)]
public string Name { get; set; }
//[DataMember(Order = 3)]
//public string Gender { get; set; }
[DataMember(Order = 4)]
public DateTime DateOfBirth { get; set; }
[DataMember(Order = 5)]
public EmployeeType Type { get; set; }
}
Along the same lines if a new non required field City is added, the existing clients will not send data for this field, and the service WILL NOT throw an exception as it is not a required field.
[DataContract(Namespace = "http://pragimtech.com/Employee")]
public class Employee
{
[DataMember(Order = 1)]
public int Id { get; set; }
[DataMember(Order = 2)]
public string Name { get; set; }
//[DataMember(Order = 3)]
//public string Gender { get; set; }
[DataMember(Order = 4)]
public DateTime DateOfBirth { get; set; }
[DataMember(Order = 5)]
public EmployeeType Type { get; set; }
[DataMember(Order = 5)]
public string City { get; set; }
}
On the other hand, if you make it a required field, the service would throw an exception.
For a full list of changes and the impact that could have on the existing clients, please refer to the following MSDN article
http://msdn.microsoft.com/en-us/library/ff384251.aspx
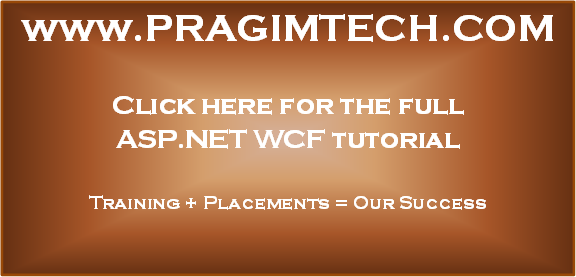
Hi Sir,
ReplyDeleteCan you please give the detailed explanation about "Multiple Endpoints at a Single ListenUri" using EndpointAddress and ListenUri.