Suggested Videos
Part 139 - How to upload and download files using asp.net and c#
Part 140 - Creating an image gallery using asp.net and c#
Part 141 - Contact us page using asp.net and c#
In Part 141, we discussed designing "contact us" page. In this video, we will implement the server side code. Once the form is submitted, an email should be sent to the support group. The email should contain all the details that are entered in the contact us form.
Please make sure to include the following 2 namespaces.
using System;
using System.Net.Mail;
contactus.aspx.cs:
protected void Button1_Click(object sender, EventArgs e)
{
try
{
if (Page.IsValid)
{
MailMessage mailMessage = new MailMessage();
mailMessage.From = new MailAddress("YourGmailID@gmail.com");
mailMessage.To.Add("AdministratorID@YourCompany.com");
mailMessage.Subject = txtSubject.Text;
mailMessage.Body = "<b>Sender Name : </b>" + txtName.Text + "<br/>"
+ "<b>Sender Email : </b>" + txtEmail.Text + "<br/>"
+ "<b>Comments : </b>" + txtComments.Text;
mailMessage.IsBodyHtml = true;
SmtpClient smtpClient = new SmtpClient("smtp.gmail.com", 587);
smtpClient.EnableSsl = true;
smtpClient.Credentials = new
System.Net.NetworkCredential("YourGmailID@gmail.com", "YourGmailPassowrd");
smtpClient.Send(mailMessage);
Label1.ForeColor = System.Drawing.Color.Blue;
Label1.Text = "Thank you for contacting us";
txtName.Enabled = false;
txtEmail.Enabled = false;
txtComments.Enabled = false;
txtSubject.Enabled = false;
Button1.Enabled = false;
}
}
catch (Exception ex)
{
// Log the exception information to
// database table or event viewer
Label1.ForeColor = System.Drawing.Color.Red;
Label1.Text = "There is an unkwon problem. Please try later";
}
}
Part 139 - How to upload and download files using asp.net and c#
Part 140 - Creating an image gallery using asp.net and c#
Part 141 - Contact us page using asp.net and c#
In Part 141, we discussed designing "contact us" page. In this video, we will implement the server side code. Once the form is submitted, an email should be sent to the support group. The email should contain all the details that are entered in the contact us form.
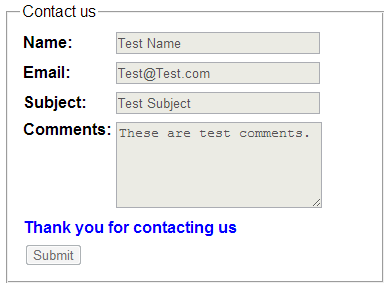
Please make sure to include the following 2 namespaces.
using System;
using System.Net.Mail;
contactus.aspx.cs:
protected void Button1_Click(object sender, EventArgs e)
{
try
{
if (Page.IsValid)
{
MailMessage mailMessage = new MailMessage();
mailMessage.From = new MailAddress("YourGmailID@gmail.com");
mailMessage.To.Add("AdministratorID@YourCompany.com");
mailMessage.Subject = txtSubject.Text;
mailMessage.Body = "<b>Sender Name : </b>" + txtName.Text + "<br/>"
+ "<b>Sender Email : </b>" + txtEmail.Text + "<br/>"
+ "<b>Comments : </b>" + txtComments.Text;
mailMessage.IsBodyHtml = true;
SmtpClient smtpClient = new SmtpClient("smtp.gmail.com", 587);
smtpClient.EnableSsl = true;
smtpClient.Credentials = new
System.Net.NetworkCredential("YourGmailID@gmail.com", "YourGmailPassowrd");
smtpClient.Send(mailMessage);
Label1.ForeColor = System.Drawing.Color.Blue;
Label1.Text = "Thank you for contacting us";
txtName.Enabled = false;
txtEmail.Enabled = false;
txtComments.Enabled = false;
txtSubject.Enabled = false;
Button1.Enabled = false;
}
}
catch (Exception ex)
{
// Log the exception information to
// database table or event viewer
Label1.ForeColor = System.Drawing.Color.Red;
Label1.Text = "There is an unkwon problem. Please try later";
}
}

Hi venkat, I am getting a error like this
ReplyDeleteA potentially dangerous Request.Form value was detected from the client (ctl00$ctl00$ContentPlaceHolder1$MainContent$txtComments="these are t...").
please help me
Can you please help, I am getting error: there is an unknown problem. Please try later
ReplyDeleteYour unknown problem is your email. Enter your real gmail name and password.
ReplyDeleteThanks!
ReplyDeleteWorks great! do you have a tutorial on keeping all the form requests on DB?
hi venkat sir ur video is very usefull..but it's work loacaly not on remote server.why...?
ReplyDeleteI have an errors like (Please enter your valid email) ...
ReplyDeletewhat is actually problem? many thanks
hello sir I did everything u said but msg came "There is an unkwon problem. Please try later" and in my id msg came like there is someone trying to access in ur account so plz help
ReplyDeletehello sir I did everything u said but message came "There is an unknown problem. Please try later" and in my id messageg came like there is someone trying to access in your account Please help
Deletewhat did you do to solve this problem? is it working locally?
Delete