Suggested Videos
Part 23 - GridView insert update delete in asp.net
Part 24 - GridView insert update delete in asp.net using objectdatasource
Part 25 - GridView insert update delete without using datasource controls
We will be using tblProduct table for this demo. Please use the SQL script below to create and populate this table.
Create table tblProduct
(
ProdcutId int identity primary key,
Name nvarchar(50),
UnitPrice int,
QuantitySold int
)
Insert into tblProduct values ('LCD TV', 250, 120)
Insert into tblProduct values ('iPhone', 410, 220)
Insert into tblProduct values ('iPad', 175, 320)
Insert into tblProduct values ('HP Laptop', 275, 346)
Insert into tblProduct values ('Dell Desktop', 212, 314)
Insert into tblProduct values ('Blade Server', 460, 211)
We want to display total UntiPrice and QuantitySold in the footer of gridview. To achieve this
1. Create tblProducts table using the sql script
2. Drag and drop a gridview and a sqldatasource control on the webform.
3. By default the footer of a gridview control is not shown. Set "ShowFooter" property of GridView1 control to true, so that the footer will become visible.
4. Configure sqldatasource control to retrieve data from tblProducts table.
5. Associate SqlDataSource1 control with GridView1 control using the DataSourceID property of gridview control.
6. Generate event handler method for rowdatabound event of GridView1 control.
7. Copy and paste the following code in the code behind file. The code is well commented and self explanatory.
// Declare variables to hold total unit price and quantity sold
int totalUnitPrice = 0;
int totalQuanitySold = 0;
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
// Loop thru each data row and compute total unit price and quantity sold
if (e.Row.RowType == DataControlRowType.DataRow)
{
totalUnitPrice += Convert.ToInt32(DataBinder.Eval(e.Row.DataItem, "UnitPrice"));
totalQuanitySold += Convert.ToInt32(DataBinder.Eval(e.Row.DataItem, "QuantitySold"));
}
// Display totals in the gridview footer
else if (e.Row.RowType == DataControlRowType.Footer)
{
e.Row.Cells[1].Text = "Grand Total";
e.Row.Cells[1].Font.Bold = true;
e.Row.Cells[2].Text = totalUnitPrice.ToString();
e.Row.Cells[2].Font.Bold = true;
e.Row.Cells[3].Text = totalQuanitySold.ToString();
e.Row.Cells[3].Font.Bold = true;
}
}
Run the application and notice that the totals are displayed in the footer of the gridview control as expected.
Part 23 - GridView insert update delete in asp.net
Part 24 - GridView insert update delete in asp.net using objectdatasource
Part 25 - GridView insert update delete without using datasource controls
We will be using tblProduct table for this demo. Please use the SQL script below to create and populate this table.
Create table tblProduct
(
ProdcutId int identity primary key,
Name nvarchar(50),
UnitPrice int,
QuantitySold int
)
Insert into tblProduct values ('LCD TV', 250, 120)
Insert into tblProduct values ('iPhone', 410, 220)
Insert into tblProduct values ('iPad', 175, 320)
Insert into tblProduct values ('HP Laptop', 275, 346)
Insert into tblProduct values ('Dell Desktop', 212, 314)
Insert into tblProduct values ('Blade Server', 460, 211)
We want to display total UntiPrice and QuantitySold in the footer of gridview. To achieve this
1. Create tblProducts table using the sql script
2. Drag and drop a gridview and a sqldatasource control on the webform.
3. By default the footer of a gridview control is not shown. Set "ShowFooter" property of GridView1 control to true, so that the footer will become visible.
4. Configure sqldatasource control to retrieve data from tblProducts table.
5. Associate SqlDataSource1 control with GridView1 control using the DataSourceID property of gridview control.
6. Generate event handler method for rowdatabound event of GridView1 control.
7. Copy and paste the following code in the code behind file. The code is well commented and self explanatory.
// Declare variables to hold total unit price and quantity sold
int totalUnitPrice = 0;
int totalQuanitySold = 0;
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
// Loop thru each data row and compute total unit price and quantity sold
if (e.Row.RowType == DataControlRowType.DataRow)
{
totalUnitPrice += Convert.ToInt32(DataBinder.Eval(e.Row.DataItem, "UnitPrice"));
totalQuanitySold += Convert.ToInt32(DataBinder.Eval(e.Row.DataItem, "QuantitySold"));
}
// Display totals in the gridview footer
else if (e.Row.RowType == DataControlRowType.Footer)
{
e.Row.Cells[1].Text = "Grand Total";
e.Row.Cells[1].Font.Bold = true;
e.Row.Cells[2].Text = totalUnitPrice.ToString();
e.Row.Cells[2].Font.Bold = true;
e.Row.Cells[3].Text = totalQuanitySold.ToString();
e.Row.Cells[3].Font.Bold = true;
}
}
Run the application and notice that the totals are displayed in the footer of the gridview control as expected.
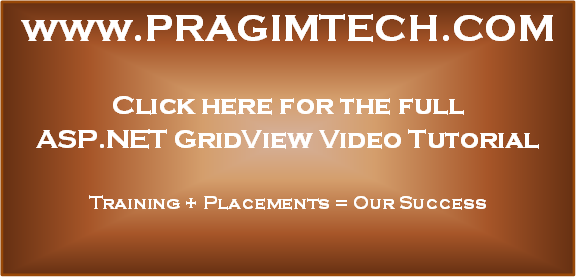
No comments:
Post a Comment
It would be great if you can help share these free resources