Suggested Videos
Part 57 - Angular canactivate guard example | Text | Slides
Part 58 - Passing data between components in angular | Text | Slides
Part 59 - Edit form in angular | Text | Slides
In this video we will discuss performing Delete operation in Angular. To set the expectations right, we will be deleting data on the client side. We will discuss deleting data from a database table in our upcoming videos when we implement the server side service.
So here is what we want to do. When we click the "Delete" button, we want to display a confirmation. Are you sure, you want to delete. If the user clicks "No", the confirmation should disappear without deleting. If the user clicks "Yes", only then the employee record should be deleted.
Changes in employee.service.ts : Include the following deleteEmployee() method. The easiest method to remove an element from an array is by using splice() method.
This method has 2 parameters. The first parameters specifies the zero based index of the element we want to remove from the array. The second parameter specifies the number of elements to remove. In our case, we want to remove just one element, so we specified 1 as the value.
Changes in display-employee.component.ts : The changes are commented and self explanatory
Changes in display-employee.component.html : Modify the HTML in panel-footer <div> as shown below.
Changes in list-employees.component.html : Bind to the child component notifyDelete event.
Changes in list-employees.component.ts : Include notifyDelete event handler method (onDeleteNotification) and delete the respective employee from the filteredEmployees array to which the view template is bound.
Part 57 - Angular canactivate guard example | Text | Slides
Part 58 - Passing data between components in angular | Text | Slides
Part 59 - Edit form in angular | Text | Slides
In this video we will discuss performing Delete operation in Angular. To set the expectations right, we will be deleting data on the client side. We will discuss deleting data from a database table in our upcoming videos when we implement the server side service.
So here is what we want to do. When we click the "Delete" button, we want to display a confirmation. Are you sure, you want to delete. If the user clicks "No", the confirmation should disappear without deleting. If the user clicks "Yes", only then the employee record should be deleted.

Changes in employee.service.ts : Include the following deleteEmployee() method. The easiest method to remove an element from an array is by using splice() method.
This method has 2 parameters. The first parameters specifies the zero based index of the element we want to remove from the array. The second parameter specifies the number of elements to remove. In our case, we want to remove just one element, so we specified 1 as the value.
deleteEmployee(id: number) {
const i = this.listEmployees.findIndex(e => e.id === id);
if (i !== -1) {
this.listEmployees.splice(i, 1);
}
}
Changes in display-employee.component.ts : The changes are commented and self explanatory
import {
Component, OnInit, Input,
Output, EventEmitter
} from '@angular/core';
import { Employee } from '../models/employee.model';
import { ActivatedRoute, Router } from '@angular/router';
import { EmployeeService } from './employee.service';
@Component({
selector: 'app-display-employee',
templateUrl: './display-employee.component.html',
styleUrls: ['./display-employee.component.css']
})
export class DisplayEmployeeComponent implements OnInit {
@Input() employee: Employee;
@Input() searchTerm: string;
// This output event will be used to
notify parent component i.e
// ListEmployeesComponent when an
employee is deleted. so the
// ListEmployeesComponent can delete
that respective employee
// from the filteredEmployees array to
which the template is bound
@Output() notifyDelete:
EventEmitter<number> = new
EventEmitter<number>();
// This property is used in the view
template to show and hide
// delete confirmation
confirmDelete = false;
private selectedEmployeeId: number;
constructor(private _route: ActivatedRoute, private _router: Router,
private _employeeService: EmployeeService) { }
ngOnInit() {
this.selectedEmployeeId = +this._route.snapshot.paramMap.get('id');
}
viewEmployee() {
this._router.navigate(['/employees', this.employee.id], {
queryParams: { 'searchTerm': this.searchTerm }
});
}
editEmployee() {
this._router.navigate(['/edit', this.employee.id]);
}
// Call the EmployeeService delete
method and raise notifyDelete event, so
// the
ListEemployeesComponent can delete the same employee from
it's
// filtered list array
deleteEmployee() {
this._employeeService.deleteEmployee(this.employee.id);
this.notifyDelete.emit(this.employee.id);
}
}
Changes in display-employee.component.html : Modify the HTML in panel-footer <div> as shown below.
<div class="panel-footer">
<button class="btn btn-primary" (click)="viewEmployee()">View</button>
<button class="btn btn-primary" (click)="editEmployee()">Edit</button>
<span *ngIf="confirmDelete">
<span>Are you sure you want to delete ?</span>
<button class="btn btn-danger" (click)="deleteEmployee()">Yes</button>
<button class="btn btn-primary" (click)="confirmDelete=false">No</button>
</span>
<span *ngIf="!confirmDelete">
<button class="btn btn-danger" (click)="confirmDelete=true">Delete</button>
</span>
</div>
Changes in list-employees.component.html : Bind to the child component notifyDelete event.
<div class="form-group">
<input type="text" class="form-control"
placeholder="Search By Name" [(ngModel)]="searchTerm" />
</div>
<div *ngFor="let employee of filteredEmployees">
<app-display-employee [employee]="employee" [searchTerm]="searchTerm"
(notifyDelete)="onDeleteNotification($event)">
</app-display-employee>
</div>
Changes in list-employees.component.ts : Include notifyDelete event handler method (onDeleteNotification) and delete the respective employee from the filteredEmployees array to which the view template is bound.
onDeleteNotification(id: number) {
const i = this.filteredEmployees.findIndex(e => e.id === id);
if (i !== -1) {
this.filteredEmployees.splice(i, 1);
}
}
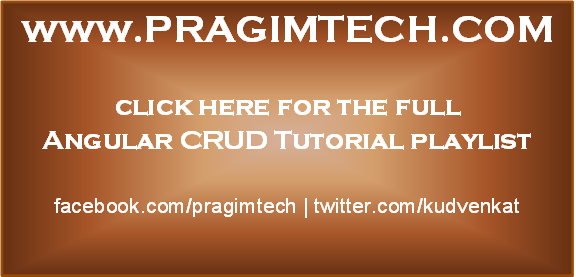
After Delete All Employee , I will try to create new employee , But it make a face some error
ReplyDelete