Suggested Videos
Part 23 - Angular select list validation | Text | Slides
Part 24 - Angular value vs ngValue | Text | Slides
Part 25 - Angular custom validator example template driven forms | Text | Slides
In this video we will discuss, how to make the select list custom required validator configurable and reusable. This is continuation to Part 25. Please watch Part 25 from Angular CRUD tutorial before proceeding.
Here is the SELECT list custom required validator we implemented in our previous video
Consider this line of code : Notice we have hard-coded the default option value '-1'. Because of this hard-coded value, we will not be able to reuse this validator with another SELECT list if it has a different default option value other than '-1'.
return control.value === '-1' ? { 'defaultSelected': true } : null;
To make this custom validator reusable, we want to be able to do pass the default option value from the template to our custom validator as shown below. Notice we are using our custom validator selector and passing it the default option value. In this case we are passing -101. If you have another SELECT list, and if it's default option value is -1, you simply pass that value.
<select appSelectValidator="-101" #department="ngModel" ....>
For this to work, we have to create a corresponding input property in the custom validator class. Modify SelectRequiredValidatorDirective as shown below. The changes are commented and self-explanatory
Please note : Since this is a directive input property, the input property name and the selector name must match.
@Input() appSelectValidator: string;
For some reason if you do not like the input property name, you can use an alias as shown below.
@Input('appSelectValidator') defaultValue: string;
We are now able to specify the default option value using the directive input property. This makes our custom validator configurable and reusable. We can now use this custom required validator to validate any SELECT list in our Angular application.
Part 23 - Angular select list validation | Text | Slides
Part 24 - Angular value vs ngValue | Text | Slides
Part 25 - Angular custom validator example template driven forms | Text | Slides
In this video we will discuss, how to make the select list custom required validator configurable and reusable. This is continuation to Part 25. Please watch Part 25 from Angular CRUD tutorial before proceeding.
Here is the SELECT list custom required validator we implemented in our previous video
import
{ Validator, AbstractControl, NG_VALIDATORS } from
'@angular/forms';
import
{ Directive } from '@angular/core';
@Directive({
selector: '[appSelectValidator]',
providers: [{
provide: NG_VALIDATORS,
useExisting: SelectRequiredValidatorDirective,
multi: true
}]
})
export
class SelectRequiredValidatorDirective implements
Validator {
validate(control: AbstractControl): { [key: string]: any } | null {
return control.value === '-1'
? { 'defaultSelected':
true } : null;
}
}
Consider this line of code : Notice we have hard-coded the default option value '-1'. Because of this hard-coded value, we will not be able to reuse this validator with another SELECT list if it has a different default option value other than '-1'.
return control.value === '-1' ? { 'defaultSelected': true } : null;
To make this custom validator reusable, we want to be able to do pass the default option value from the template to our custom validator as shown below. Notice we are using our custom validator selector and passing it the default option value. In this case we are passing -101. If you have another SELECT list, and if it's default option value is -1, you simply pass that value.
<select appSelectValidator="-101" #department="ngModel" ....>
For this to work, we have to create a corresponding input property in the custom validator class. Modify SelectRequiredValidatorDirective as shown below. The changes are commented and self-explanatory
import
{ Validator, AbstractControl, NG_VALIDATORS } from
'@angular/forms';
// Import input from @angular/core
package
import
{ Directive, Input } from '@angular/core';
@Directive({
selector: '[appSelectValidator]',
providers: [{
provide: NG_VALIDATORS,
useExisting: SelectRequiredValidatorDirective,
multi: true
}]
})
export
class SelectRequiredValidatorDirective implements
Validator {
// Create input property to receive the
// specified default option value
@Input() appSelectValidator: string;
validate(control: AbstractControl): { [key: string]: any } | null {
// Remove the hard-coded value and use the input
property instead
return control.value === this.appSelectValidator
?
{ 'defaultSelected':
true } : null;
}
}
Please note : Since this is a directive input property, the input property name and the selector name must match.
@Input() appSelectValidator: string;
For some reason if you do not like the input property name, you can use an alias as shown below.
@Input('appSelectValidator') defaultValue: string;
We are now able to specify the default option value using the directive input property. This makes our custom validator configurable and reusable. We can now use this custom required validator to validate any SELECT list in our Angular application.
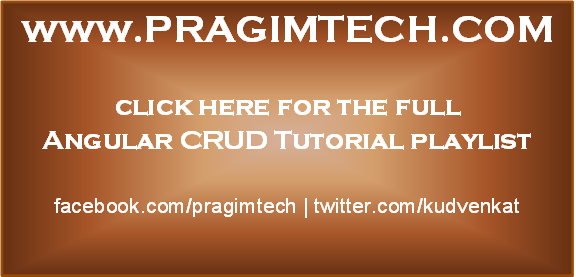
Dear Sir,
ReplyDeletePlease post some of the important interview question
related to the Angular(Latest). Basic to advance if as soon as possible.