Suggested Videos
Part 5 - Two way databinding in AngularJS
Part 6 - AngularJS ng-repeat directive
Part 7 - Handling events in AngularJS
In this video we will discuss filters in AngularJS
Filters in angular can do 3 different things
1. Format data
2. Sort data
3. Filter data
Filters can be used with a binding expression or a directive
To apply a filter use pipe (|) character
Syntax : {{ expression | filterName:parameter }}
Angular filters for formatting data
Angular Date formats
Angular date format documentation
https://docs.angularjs.org/api/ng/filter/date
limitTo filter : Can be used to limit the number of rows or characters in a string.
Syntax : {{ expression | limitTo : limit : begin}}
The following example uses all the above filters
Script.js
HtmlPage1.html
Styles.css
Upcoming videos : Sorting and filtering data using angular filters
Part 5 - Two way databinding in AngularJS
Part 6 - AngularJS ng-repeat directive
Part 7 - Handling events in AngularJS
In this video we will discuss filters in AngularJS
Filters in angular can do 3 different things
1. Format data
2. Sort data
3. Filter data
Filters can be used with a binding expression or a directive
To apply a filter use pipe (|) character
Syntax : {{ expression | filterName:parameter }}
Angular filters for formatting data
Filter | Description |
lowercase | Formats all characters to lowercase |
uppercase | Formats all characters to uppercase |
number | Formats a number as text. Includes comma as thousands separator and the number of decimal places can be specified |
currency | Formats a number as a currency. $ is default. Custom currency and decimal places can be specified |
date | Formats date to a string based on the requested format |
Angular Date formats
Format | Result |
yyyy | 4 digit year. Exampe 1998 |
yy | 2 digit year. Example 1998 => 98 |
MMMM | January - December |
MMM | Jan - Dec |
MM | 01 - 12 |
M | 1 - 12 (No leading ZEROS) |
dd | 01 - 31 |
d | 1 - 31 (No leading ZEROS) |
Angular date format documentation
https://docs.angularjs.org/api/ng/filter/date
limitTo filter : Can be used to limit the number of rows or characters in a string.
Syntax : {{ expression | limitTo : limit : begin}}
The following example uses all the above filters

Script.js
var app = angular
.module("myModule", [])
.controller("myController",
function
($scope) {
var employees = [
{
name: "Ben",
dateOfBirth: new Date("November 23,
1980"),
gender: "Male",
salary: 55000.788
},
{
name: "Sara",
dateOfBirth: new Date("May 05,
1970"),
gender: "Female", salary: 68000
},
{
name: "Mark",
dateOfBirth: new Date("August 15,
1974"),
gender: "Male",
salary: 57000
},
{
name: "Pam", dateOfBirth: new Date("October 27,
1979"),
gender: "Female",
salary: 53000
},
{
name: "Todd",
dateOfBirth: new Date("December 30,
1983"),
gender: "Male",
salary: 60000
}
];
$scope.employees = employees;
$scope.rowCount = 3;
});
HtmlPage1.html
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title></title>
<script src="Scripts/angular.min.js"></script>
<script src="Scripts/Script.js"></script>
<link href="Styles.css" rel="stylesheet" />
</head>
<body ng-app="myModule">
<div ng-controller="myController">
Rows to display : <input type="number" step="1"
ng-model="rowCount" max="5" min="0" />
<br /><br />
<table>
<thead>
<tr>
<th>Name</th>
<th>Date of Birth</th>
<th>Gender</th>
<th>Salary (number filter)</th>
<th>Salary (currency filter)</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="employee in
employees | limitTo:rowCount">
<td> {{ employee.name | uppercase }} </td>
<td> {{ employee.dateOfBirth | date:"dd/MM/yyyy" }}
</td>
<td> {{ employee.gender }}
</td>
<td> {{ employee.salary | number:2 }} </td>
<td> {{ employee.salary | currency : "£" : 1 }} </td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
Styles.css
body {
font-family: Arial;
}
table {
border-collapse: collapse;
}
td {
border: 1px solid black;
padding: 5px;
}
th {
border: 1px solid black;
padding: 5px;
text-align: left;
}
Upcoming videos : Sorting and filtering data using angular filters
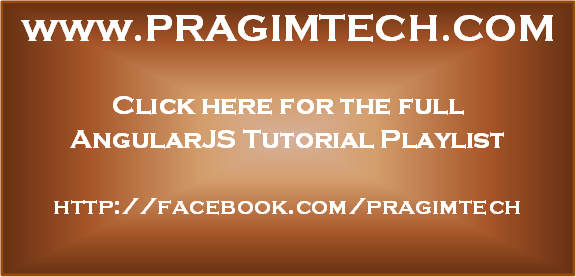
Hi vankat good tutorial
ReplyDeleteGreat work, I'm a big fan of yours ! greetings from Mauritius
ReplyDeleteExcellent in depth Explanations!
ReplyDeleteA correction: While trying to work with the code sample the increment did not work, in the HTML page ng-model directive for rowCount had to be shifted position within the input tag to make it work. Strange but worked only when declared before the step attribute or at the end of the input tag.
This Worked:
input type="number" ng-model="rowCount" step="1" max="5" min="0"
This did not work:
input type="number" step="1" ng-model="rowCount" max="5" min="0"
Your videos are too good .
ReplyDeleteThanks
please post tutorials on node js