Suggested Videos
Part 81 - jquery tooltip widget
Part 82 - jquery tooltip from database
Part 83 - jquery ui progress bar
In this video we will discuss, using jQuery progressbar with asp.net file upload control.
While the files are being uploaded we want to display the jQuery progress bar.
As soon as the upload is complete, we want to display complete message in the progress bar, and it should slowly fade out.
Step 1 : Create new asp.net web application project. Name it Demo.
Step 2 : Add a New Folder to the project. Name it Uploads.
Step 3 : Add a new Generic Handler to the project. Name it UploadHandler.ashx. Copy and paste the following code
Step 4 : Add a WebForm to the project. Name it WebForm1.aspx. Copy and paste the following HTML and jQuery code
Please note : contentType option should be set to false to tell jQuery to not set any content type header. processData option should also be set to false, otherwise, jQuery will try to convert your FormData into a string, which will fail.
Part 81 - jquery tooltip widget
Part 82 - jquery tooltip from database
Part 83 - jquery ui progress bar
In this video we will discuss, using jQuery progressbar with asp.net file upload control.
While the files are being uploaded we want to display the jQuery progress bar.
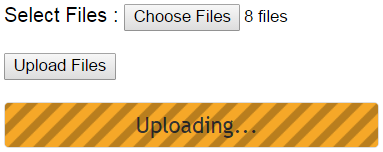
As soon as the upload is complete, we want to display complete message in the progress bar, and it should slowly fade out.
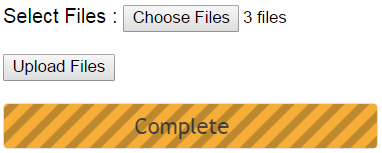
Step 1 : Create new asp.net web application project. Name it Demo.
Step 2 : Add a New Folder to the project. Name it Uploads.
Step 3 : Add a new Generic Handler to the project. Name it UploadHandler.ashx. Copy and paste the following code
using System.IO;
using System.Web;
namespace Demo
{
public class UploadHandler : IHttpHandler
{
public void ProcessRequest(HttpContext context)
{
if (context.Request.Files.Count > 0)
{
HttpFileCollection selectedFiles = context.Request.Files;
for (int i = 0; i < selectedFiles.Count; i++)
{
System.Threading.Thread.Sleep(1000);
HttpPostedFile
PostedFile = selectedFiles[i];
string FileName =
context.Server.MapPath("~/Uploads/"
+ Path.GetFileName(PostedFile.FileName));
PostedFile.SaveAs(FileName);
}
}
}
public bool IsReusable
{
get
{
return false;
}
}
}
}
Step 4 : Add a WebForm to the project. Name it WebForm1.aspx. Copy and paste the following HTML and jQuery code
<%@ Page Language="C#" AutoEventWireup="true"
CodeBehind="WebForm1.aspx.cs" Inherits="Demo.WebForm1" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src="jquery-1.11.2.js"></script>
<script src="jquery-ui.js"></script>
<link href="jquery-ui.css" rel="stylesheet" />
<script type="text/javascript">
$(document).ready(function () {
$("#btnUpload").click(function (event) {
var files = $("#FileUpload1")[0].files;
if (files.length > 0) {
var formData = new FormData();
for (var i = 0; i < files.length;
i++) {
formData.append(files[i].name, files[i]);
}
var progressbarLabel = $('#progressBar-label');
var progressbarDiv = $('#progressbar');
$.ajax({
url: 'UploadHandler.ashx',
method: 'post',
data: formData,
contentType: false,
processData: false,
success: function () {
progressbarLabel.text('Complete');
progressbarDiv.fadeOut(2000);
},
error: function (err) {
alert(err.statusText);
}
});
progressbarLabel.text('Uploading...');
progressbarDiv.progressbar({
value: false
}).fadeIn(500);
}
});
});
</script>
</head>
<body style="font-family:
Arial">
<form id="form1" runat="server">
Select Files :
<asp:FileUpload ID="FileUpload1" runat="server" AllowMultiple="true" />
<br /><br />
<input type="button" id="btnUpload" value="Upload
Files" />
<br /><br />
<div style="width: 300px">
<div id="progressbar" style="position: relative; display: none">
<span style="position: absolute; left: 35%; top: 20%" id="progressBar-label">
Uploading...
</span>
</div>
</div>
</form>
</body>
</html>
Please note : contentType option should be set to false to tell jQuery to not set any content type header. processData option should also be set to false, otherwise, jQuery will try to convert your FormData into a string, which will fail.
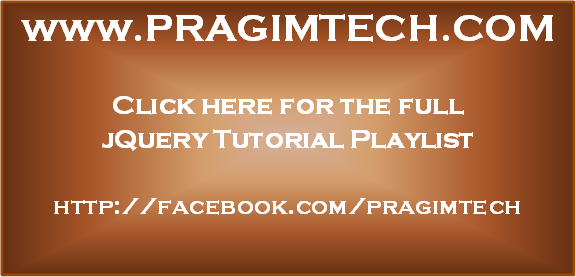
Sir,
ReplyDeletePlease provide us a video explaining large files upload (1GB+) with real % ProgressBar.
Thanks
If I run this example it works with 1 file, but if try to upload 2 of more files I get an "Internal Server Error". Can somebody explain why and how can I solve this problem somewhere in Windows settings.
ReplyDeletehi venkat thanks for great teachings,
ReplyDeletein my case Allowmultiple="true" is not working multiple=true is working why like that
require .NET4.5 or above.
Deletevar files = $("#FileUpload1")[0].files;
ReplyDeletethis code has been used what does it mean by ?
i couldn't get it.
I changed it to
Deletevar files = $("#<%=FileUpload1.ClientID%>").get(0).files;
and it works good.
What if my file upload control is inside a gridview item template
ReplyDeleteHow to rename file before move in folder?
ReplyDeleteHow to implement progress bar with percentage completion in multiple tabs..? Please help me with this sir..
ReplyDelete