Suggested Videos
Part 71 - Calling live json web service using jquery ajax
Part 72 - Autocomplete textbox using jquery in asp.net
Part 73 - Autocomplete textbox using jquery and asp.net web service
In this video we will discuss how to implement accordion panel in an ASP.NET web forms application using jQuery.
What is accordion
accordion is great for displaying collapsible content panels for presenting information in a limited amount of space.
Without accordion, the page will be as shown below
2 simple steps to produce an accordion using jQuery
Step 1 : The HTML of the accordion container needs pairs of headers and content panels as shown below
Step 2 : Invoke accordion() function on the container div
$('#accordion').accordion();
Steps to retrieve data from a database table and present it using jQuery accordion in an asp.net webforms application
Step 1 : SQL Script to create and populate table tblCountries
Step 2 : Create a stored procedure to retrieve country data
Step 3 : Create new asp.net web application project. Name it Demo.
Step 4 : Include a connection string in the web.config file to your database.
Step 5 : Add Country class to the project. Copy and paste the following code.
Step 6 : Add a WebService (ASMX) to the project. Name it CountryService.asmx. Copy and paste the following code.
Step 7 : Download jQuery UI from jqueryui.com. Copy and paste the following files in your project.
a) jquery-ui.js
b) jquery-ui.css
c) images
Step 8 : Add a WebForm to the ASP.NET project. Copy and paste the following HTML and jQuery code.
jQuery Accordion Widget Options
For the complete list of options, methods and events of accordion widget
http://api.jqueryui.com/accordion/
Part 71 - Calling live json web service using jquery ajax
Part 72 - Autocomplete textbox using jquery in asp.net
Part 73 - Autocomplete textbox using jquery and asp.net web service
In this video we will discuss how to implement accordion panel in an ASP.NET web forms application using jQuery.
What is accordion
accordion is great for displaying collapsible content panels for presenting information in a limited amount of space.

Without accordion, the page will be as shown below

2 simple steps to produce an accordion using jQuery
Step 1 : The HTML of the accordion container needs pairs of headers and content panels as shown below
<div id="accordion" style="width: 400px">
<h3>Header 1</h3>
<div>Content Panel 1</div>
<h3>Header 2</h3>
<div>Content Panel 2</div>
<h3>Header 3</h3>
<div>Content Panel 3</div>
</div>
Step 2 : Invoke accordion() function on the container div
$('#accordion').accordion();
Steps to retrieve data from a database table and present it using jQuery accordion in an asp.net webforms application

Step 1 : SQL Script to create and populate table tblCountries
Create table tblCountries
(
ID int primary
key identity,
Name nvarchar(20),
CountryDescription nvarchar(1000)
)
Go
Insert into tblCountries values ('India','India Description')
Insert into tblCountries values ('United States','United States Description')
Insert into tblCountries values ('United Kingdom','United Kingdom Description')
Insert into tblCountries values ('Canada','Canada Description')
Step 2 : Create a stored procedure to retrieve country data
Create procedure spGetCountries
as
Begin
Select *
from tblCountries
End
Step 3 : Create new asp.net web application project. Name it Demo.
Step 4 : Include a connection string in the web.config file to your database.
<add name="DBCS"
connectionString="server=.;database=SampleDB;integrated security=SSPI"/>
Step 5 : Add Country class to the project. Copy and paste the following code.
namespace Demo
{
public class Country
{
public int ID { get; set; }
public string Name { get; set; }
public string CountryDescription { get; set; }
}
}
Step 6 : Add a WebService (ASMX) to the project. Name it CountryService.asmx. Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Web.Services;
namespace Demo
{
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class CountryService : System.Web.Services.WebService
{
[WebMethod]
public List<Country> GetCountries()
{
List<Country> listCountries = new List<Country>();
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("spGetCountries", con);
cmd.CommandType = CommandType.StoredProcedure;
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Country country = new Country();
country.ID = Convert.ToInt32(rdr["ID"]);
country.Name = rdr["Name"].ToString();
country.CountryDescription
= rdr["CountryDescription"].ToString();
listCountries.Add(country);
}
}
return listCountries;
}
}
}
Step 7 : Download jQuery UI from jqueryui.com. Copy and paste the following files in your project.
a) jquery-ui.js
b) jquery-ui.css
c) images
Step 8 : Add a WebForm to the ASP.NET project. Copy and paste the following HTML and jQuery code.
<%@ Page Language="C#" AutoEventWireup="true"
CodeBehind="WebForm1.aspx.cs" Inherits="Demo.WebForm1" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src="jquery-1.11.2.js"></script>
<script src="jquery-ui.js"></script>
<link href="jquery-ui.css" rel="stylesheet" />
<script type="text/javascript">
$(document).ready(function () {
$.ajax({
url: 'CountryService.asmx/GetCountries',
method: 'post',
contentType: 'application/json;charset=utf-8',
dataType: 'json',
success: function (data) {
var htmlString = '';
$(data.d).each(function (index, country) {
htmlString += '<h3>' +
country.Name + '</h3><div>'
+
country.CountryDescription + '</div>';
});
$('#accordion').html(htmlString).accordion({
collapsible: true,
active: 2,
});
}
});
});
</script>
</head>
<body style="font-family:
Arial">
<form id="form1" runat="server">
<div id="accordion" style="width: 600px">
</div>
</form>
</body>
</html>
jQuery Accordion Widget Options
Option | Description |
collapsible | By default, atleast one section need to be active. If you want to collapse all the sections, including the one that is active, set this option to true. |
active | This option can be set to a boolean value or integer. Setting active to false will collapse all panels. This requires the collapsible option to be true. An Integer value will make the corresponding panel active. Panels use zero-based index. |
For the complete list of options, methods and events of accordion widget
http://api.jqueryui.com/accordion/
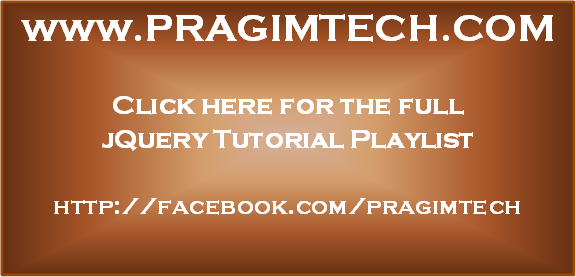
No comments:
Post a Comment
It would be great if you can help share these free resources