Suggested Videos
Part 34 - Binding event handlers using jquery on method
Part 35 - Passing data to event handler in jQuery
Part 36 - jQuery add event handler to dynamically created element
In this video we will discuss the concept of event delegation in jQuery.
Event delegation allows us to attach a single event listener, to a parent element, that will fire for all descendants matching a selector, whether those descendants exist now or are added in the future. Both on() and delegate() functions allow us to perform event delegation.
How does the following example work :
1. When you click on a list item (li), the event gets bubbled up to its parent (ul) as the list item (li) does not have it's own click event handler
2. The bubbled event is handled by the the parent (ul) element, as it has a click event handler.
3. When a new list item is added dynamicaly, you don't have to add the click event handler explicitly to it. Since the newly created list item (li) is added to the same parent element (ul), the click event of this list item also gets bubbled upto the same parent and will be handled by it.
4. undelegate() stops event delegation
If you are using jQuery 1.7 or higher version, jQuery recommends to use on() to perform event delegation instead of delegate(). The above example can be very easily rewritten using on() and off() functions, instead of delegate() and undelegate() functions as shown below. We discussed performing event delegation using on() function in detail in Part 36 of jQuery tutorial.
Part 34 - Binding event handlers using jquery on method
Part 35 - Passing data to event handler in jQuery
Part 36 - jQuery add event handler to dynamically created element
In this video we will discuss the concept of event delegation in jQuery.
Event delegation allows us to attach a single event listener, to a parent element, that will fire for all descendants matching a selector, whether those descendants exist now or are added in the future. Both on() and delegate() functions allow us to perform event delegation.
How does the following example work :
1. When you click on a list item (li), the event gets bubbled up to its parent (ul) as the list item (li) does not have it's own click event handler
2. The bubbled event is handled by the the parent (ul) element, as it has a click event handler.
3. When a new list item is added dynamicaly, you don't have to add the click event handler explicitly to it. Since the newly created list item (li) is added to the same parent element (ul), the click event of this list item also gets bubbled upto the same parent and will be handled by it.
4. undelegate() stops event delegation
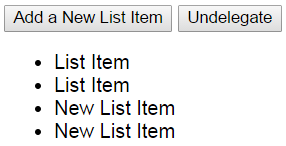
<html>
<head>
<title></title>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('ul').delegate('li', 'click', function () {
$(this).fadeOut(500);
});
$('#btnAdd').on('click', function () {
$('ul').append('<li>New List Item</li>');
});
$('#btnUndelegate').on('click', function () {
$('ul').undelegate('li', 'click');
});
});
</script>
</head>
<body style="font-family:Arial">
<input id="btnAdd" type="button" value="Add a New List
Item" />
<input id="btnUndelegate" type="button" value="Undelegate" />
<ul>
<li>List Item</li>
<li>List Item</li>
</ul>
</body>
</html>
If you are using jQuery 1.7 or higher version, jQuery recommends to use on() to perform event delegation instead of delegate(). The above example can be very easily rewritten using on() and off() functions, instead of delegate() and undelegate() functions as shown below. We discussed performing event delegation using on() function in detail in Part 36 of jQuery tutorial.
<html>
<head>
<title></title>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('ul').on('click', 'li', function () {
$(this).fadeOut(500);
});
$('#btnAdd').on('click', function () {
$('ul').append('<li>New List Item</li>');
});
$('#btnUndelegate').on('click', function () {
$('ul').off('click', 'li');
});
});
</script>
</head>
<body style="font-family:Arial">
<input id="btnAdd" type="button" value="Add a New List
Item" />
<input id="btnUndelegate" type="button" value="Undelegate" />
<ul>
<li>List Item</li>
<li>List Item</li>
</ul>
</body>
</html>
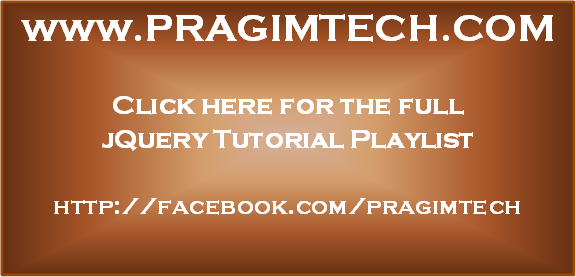
No comments:
Post a Comment
It would be great if you can help share these free resources