Suggested Videos
Part 1 - What is the use of JavaScript in ASP.NET
Part 2 - Why do we need both client side and server side validation
In this video we will discuss the disadvantages or downsides of using JavaScript.
There are two main disadvantages of JavaScript.
Security : JavaScript runs on the client machine. So a malicious user may use Javascript to do a variety of things like tracking your browsing history, stealing passwords etc. This is one of the main reasons why people disable JavaScript.
Browser Compatibility : Not all browsers treat the same piece of JavaScript in the same manner. This means the functionality and the user interface may vary from browser to browser. That is why cross-browser testing is very important. However, with JavaScript libraries like jQuery Browser Compatibility is no longer a major issue.
JavaScript Browser Compatibility Examples
Example 1 : innerText property is supported in IE & Chrome, but not in Firefox. This means the ValidatForm() JavaScript function that we worked with in Part 1, will only work in IE & Chrome but not in Firefox.
For the above JavaScript function to work in all the browsers that is in IE, Chrome & Firefox, replace innerText property with textContent as shown below.
Example 2 : The following JavaScript function ddlGenderSelectionChanged() works in Chrome and Firefox but not in Internet Explorer.
For the JavaScript function to work in all browsers (Chrome, Firefox & Internet Explorer) it needs to be modified as shown below.
Part 1 - What is the use of JavaScript in ASP.NET
Part 2 - Why do we need both client side and server side validation
In this video we will discuss the disadvantages or downsides of using JavaScript.
There are two main disadvantages of JavaScript.
Security : JavaScript runs on the client machine. So a malicious user may use Javascript to do a variety of things like tracking your browsing history, stealing passwords etc. This is one of the main reasons why people disable JavaScript.
Browser Compatibility : Not all browsers treat the same piece of JavaScript in the same manner. This means the functionality and the user interface may vary from browser to browser. That is why cross-browser testing is very important. However, with JavaScript libraries like jQuery Browser Compatibility is no longer a major issue.
JavaScript Browser Compatibility Examples
Example 1 : innerText property is supported in IE & Chrome, but not in Firefox. This means the ValidatForm() JavaScript function that we worked with in Part 1, will only work in IE & Chrome but not in Firefox.
function ValidatForm()
{
var ret = true;
if (document.getElementById("txtFirstName").value
== "")
{
document.getElementById("lblFirstName").innerText =
"First Name is required";
ret = false;
}
else {
document.getElementById("lblFirstName").innerText =
"";
}
if (document.getElementById("txtLastName").value
== "")
{
document.getElementById("lblLastName").innerText =
"Last Name is required";
ret = false;
}
else
{
document.getElementById("lblLastName").innerText =
"";
}
if (document.getElementById("txtEmail").value
== "")
{
document.getElementById("lblEmail").innerText = "Email is required";
ret = false;
}
else
{
document.getElementById("lblEmail").innerText = "";
}
return ret;
}
For the above JavaScript function to work in all the browsers that is in IE, Chrome & Firefox, replace innerText property with textContent as shown below.
function ValidatForm() {
var ret = true;
if (document.getElementById("txtFirstName").value
== "")
{
document.getElementById("lblFirstName").textContent =
"First Name is required";
ret = false;
}
else
{
document.getElementById("lblFirstName").textContent =
"";
}
if (document.getElementById("txtLastName").value
== "")
{
document.getElementById("lblLastName").textContent =
"Last Name is required";
ret = false;
}
else
{
document.getElementById("lblLastName").textContent =
"";
}
if (document.getElementById("txtEmail").value
== "")
{
document.getElementById("lblEmail").textContent =
"Email is required";
ret = false;
}
else
{
document.getElementById("lblEmail").textContent =
"";
}
return ret;
}
Example 2 : The following JavaScript function ddlGenderSelectionChanged() works in Chrome and Firefox but not in Internet Explorer.
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title></title>
<script type="text/javascript" language="javascript">
function ddlGenderSelectionChanged() {
alert('You
selected ' + ddlGender.value);
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<select id="ddlGender" onchange="ddlGenderSelectionChanged()">
<option>Male</option>
<option>Female</option>
</select>
</div>
</form>
</body>
</html>
For the JavaScript function to work in all browsers (Chrome, Firefox & Internet Explorer) it needs to be modified as shown below.
<script type="text/javascript" language="javascript">
function ddlGenderSelectionChanged()
{
alert('You selected ' + document.getElementById('ddlGender').value);
}
</script>
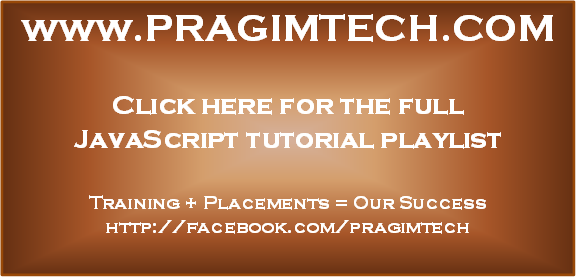
No comments:
Post a Comment
It would be great if you can help share these free resources